How to set environment via `ng serve` in Angular 6
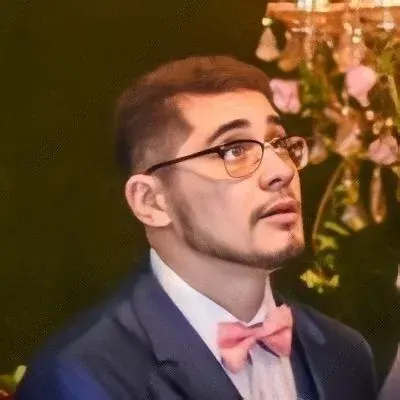
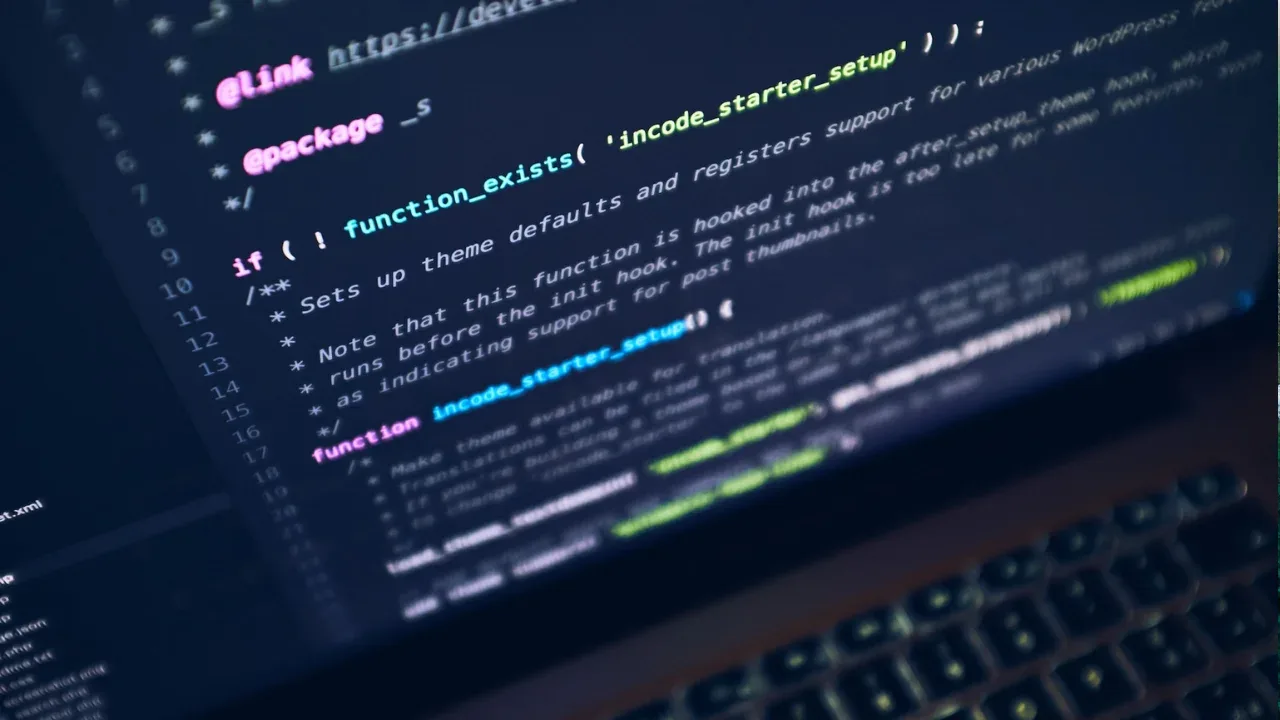
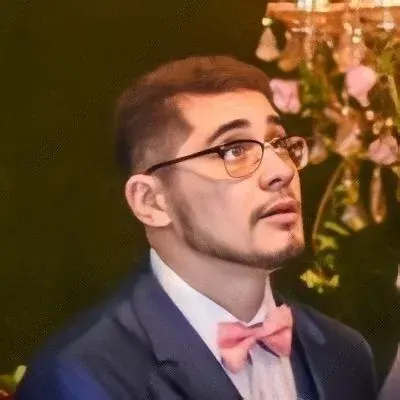
🚀🔧 How to set environment via ng serve
in Angular 6
So you've updated your Angular 5.2 app to Angular 6, followed all the instructions in the Angular update guide, and now you're trying to serve your app with a specific environment using the ng serve
command. However, you encountered an error message saying "Unknown option: '--env'". Don't worry, I've got you covered! In this guide, I'll explain why this error occurs and provide you with easy solutions to set the environment in Angular 6. Let's dive in! 💪
Why the error occurs
In Angular 6, the option to set the environment via --env
has been removed. Instead, Angular now uses the concept of configurations defined in the angular.json
file. This change was introduced to improve the build and serve processes and make them more flexible.
Easy solution #1: Use configurations in angular.json
To set the environment in Angular 6, you need to define the configurations in your angular.json
file. This file is automatically created when you generate a new Angular project using the Angular CLI. Here's how you can do it:
Open the
angular.json
file in your project's root folder.Look for the
"configurations"
section and find the configuration you want to use (e.g.,"local"
).Within the configuration object, you can specify the necessary properties such as
"fileReplacements"
or"assets"
according to your environment.
Example of a configuration for the "local"
environment:
"configurations": {
"local": {
"fileReplacements": [
{
"replace": "src/environments/environment.ts",
"with": "src/environments/environment.local.ts"
}
],
"assets": [
"src/favicon.ico",
"src/assets"
]
}
}
Save the changes in the
angular.json
file.Now you can serve your app with the desired environment using the
--configuration
flag.
For example, to serve your app with the "local"
configuration:
ng serve --configuration=local
Easy solution #2: Define custom configurations
Sometimes, you may want to define custom configurations based on your specific needs. Angular 6 allows you to do that as well. Here's how:
Open the
angular.json
file in your project's root folder.Under the
"projects"
section, find the project you're working on (e.g.,"my-app"
).Within the project object, you can add a
"architect"
section if it doesn't already exist.Inside the
"architect"
section, add a"configurations"
section with the desired configuration name.
Example of adding a custom configuration called "dev"
:
"my-app": {
"architect": {
"configurations": {
"dev": {
"fileReplacements": [
{
"replace": "src/environments/environment.ts",
"with": "src/environments/environment.dev.ts"
}
],
"assets": [
"src/favicon.ico",
"src/assets"
]
}
}
}
}
Save the changes in the
angular.json
file.Now you can serve your app with the custom configuration using the
--configuration
flag.
For example, to serve your app with the "dev"
configuration:
ng serve --configuration=dev
That's it! 🎉 You should now be able to set the environment in Angular 6 using the new configuration system.
📣 Call-to-action: Share your success story!
I hope this guide helped you resolve the issue and set the environment in your Angular 6 app using the ng serve
command. If you found this guide useful, consider sharing it with your friends and colleagues who might be facing the same problem. And don't forget to follow my blog for more exciting Angular tips and tricks! 😊