How to manage Angular2 "expression has changed after it was checked" exception when a component property depends on current datetime
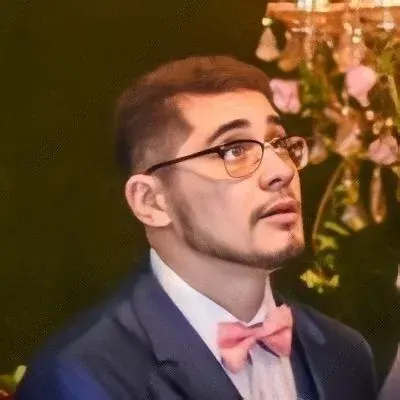
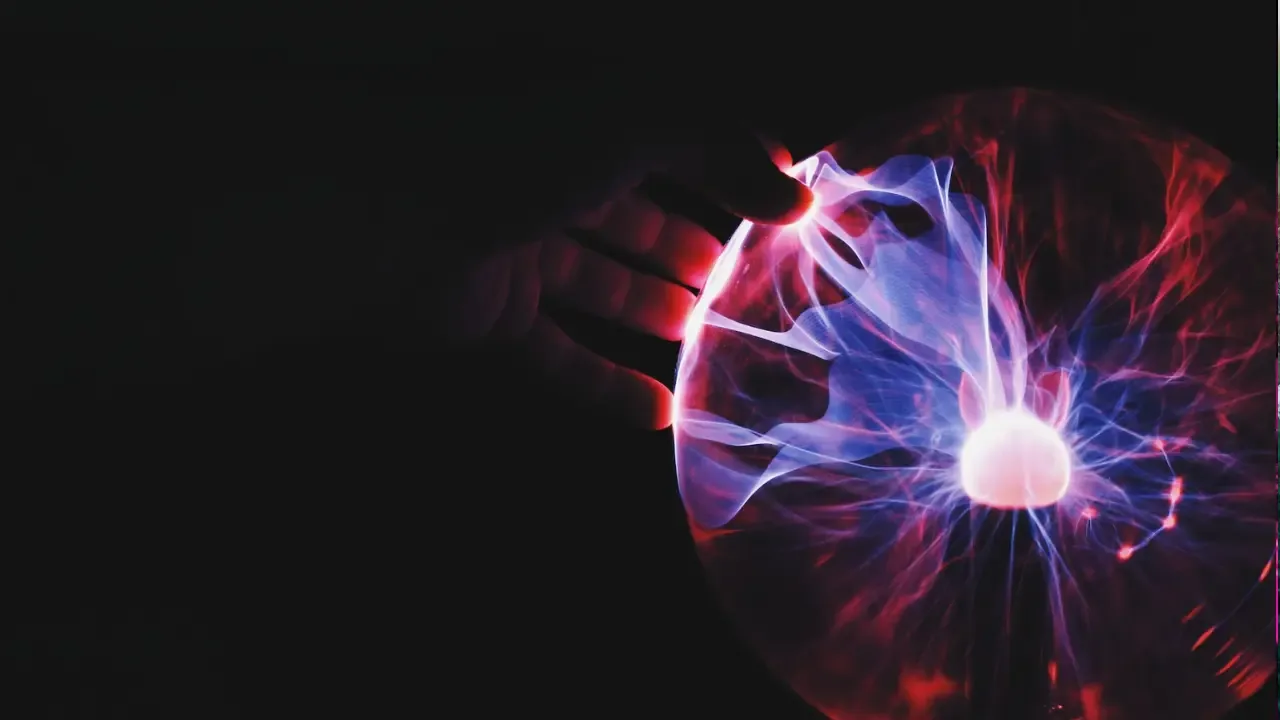
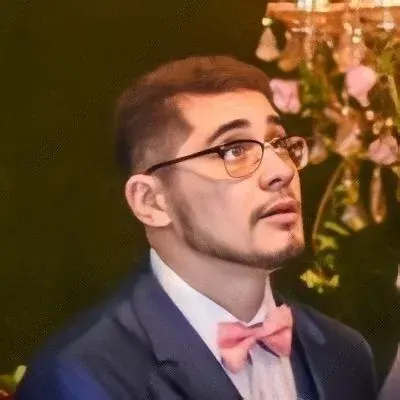
How to Manage Angular2 "expression has changed after it was checked" Exception When a Component Property Depends on Current Datetime
Have you ever encountered the annoying "expression has changed after it was checked" exception in Angular2 when a component property depends on the current datetime? Fear not, for we have got you covered! In this blog post, we will address common issues and provide easy solutions to help you overcome this problem.
The Problem: Why Does the Exception Occur?
Let's dive into the context of the issue. You have a component with styles that depend on the current datetime. Inside your component, you have a function called fontColor
which calculates the color based on the datetime of a Dto
object. The lightnessAmp
variable in the function is calculated from the current datetime, and the color changes if the Dto
object's datetime is within the last 24 hours.
Now, the problem arises when Angular's change detection mechanism kicks in. Angular checks for changes in component properties and ensures their consistency throughout the application. However, in cases where a component property depends on the current datetime, the property may change between the checking and updating phases, resulting in the "expression has changed after it was checked" exception.
The Solution: How to Fix the Exception
To solve this issue, we need to approach it from a different angle. Instead of updating the current datetime at each lifecycle hook method, we can use a more Angular-friendly approach.
Solution 1: Using ChangeDetectorRef
Angular provides a ChangeDetectorRef
class that can be used to manually trigger change detection for a component. By injecting ChangeDetectorRef
into your component and calling the detectChanges()
method, you can ensure that the component's properties are updated synchronously before Angular checks for any changes.
Here's an example of how you can implement this solution:
import { ChangeDetectorRef, Component } from '@angular/core';
@Component({
// component metadata
})
export class YourComponent {
constructor(private cdr: ChangeDetectorRef) {}
private updateFontColor(dto: Dto): void {
// Your logic to update font color based on dto
this.cdr.detectChanges();
}
}
By calling this.cdr.detectChanges()
after updating the font color, you explicitly tell Angular to check for any changes and update the component's properties accordingly, thus avoiding the exception.
Solution 2: Using setTimeout
Another workaround to prevent the exception is to delay the property update using setTimeout
. By wrapping the property update in a setTimeout
function, you ensure that the update occurs after Angular has finished its change detection cycle.
Here's an example implementation:
import { Component, OnInit } from '@angular/core';
@Component({
// component metadata
})
export class YourComponent implements OnInit {
private fontColor: string;
ngOnInit() {
this.updateFontColor();
}
private updateFontColor(): void {
setTimeout(() => {
// Your logic to update font color based on current datetime
}, 0);
}
}
By using setTimeout
with a minimal delay of 0
, you allow Angular to complete its change detection cycle before the property update occurs, effectively bypassing the exception.
Conclusion: Conquering the Exception!
Mastering the "expression has changed after it was checked" exception in Angular2 when a component property depends on the current datetime is no longer a daunting task. By implementing the solutions provided above, you can confidently manage this exception and ensure smooth functioning of your application.
So go ahead, try out these solutions, and say goodbye to frustrating exceptions! If you have any other questions or face different challenges, feel free to reach out to us. Happy coding! 💻🚀
Have you encountered the "expression has changed after it was checked" exception in Angular2? Share your experiences and solutions with us in the comments below!