How to go back last page
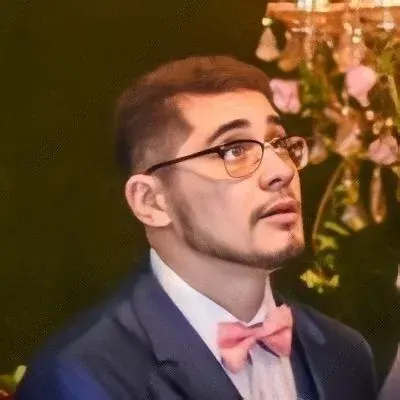
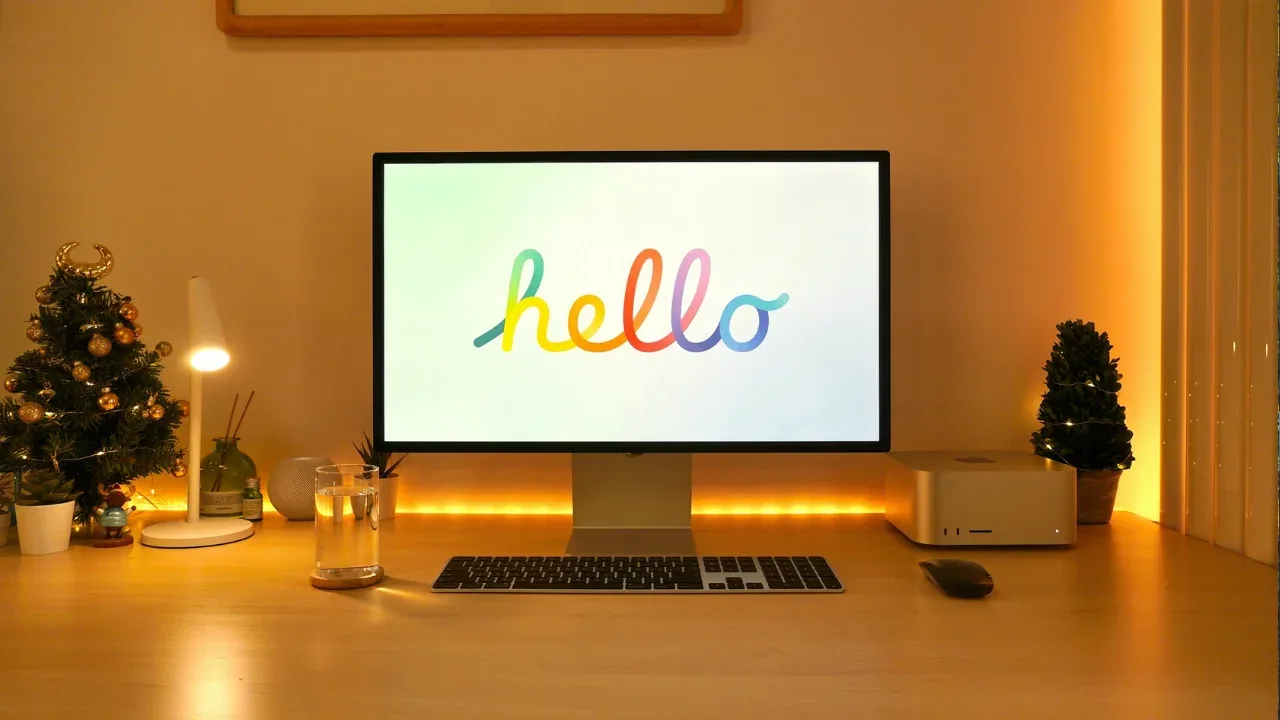
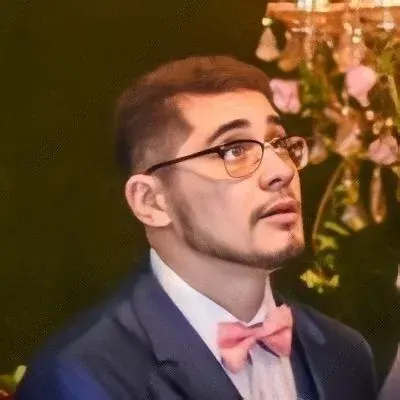
🔙 How to Go Back to the Last Page in Angular 2? 🔙
Have you ever found yourself navigating through different pages in your Angular 2 app and wished there was a smart way to go back to the previous page without all the hassle? If so, you're in luck! In this blog post, we will explore how to achieve this functionality in a simple and efficient manner.
But first, let's discuss the common issues or problems you might encounter when trying to implement a "Go Back" feature in your Angular 2 app.
🚫 Common Issues 🚫
1️⃣ Issue 1: Manually hardcoding the previous page route can lead to maintenance headaches if the page structure changes.
2️⃣ Issue 2: The Angular router does not provide a direct method to navigate to the previous page.
💡 Easy Solutions 💡
1️⃣ Solution 1: Utilize the browser's built-in history functionality.
By calling the window.history.back()
method, you can effectively navigate back to the previous page. This method triggers the same behavior as clicking the browser's back button.
2️⃣ Solution 2: Leverage the Angular router's history tracking.
Fortunately, Angular's router does keep track of the navigation history, and we can leverage this to achieve our goal. The router provides a NavigationExtras
object that allows us to control the navigation behavior.
To go back to the last page, you can use the following code:
import { Router } from '@angular/router';
// Inject the Router into your component
constructor(private router: Router) {}
// Call this method to go back to the previous page
goBack() {
this.router.navigate([''], { skipLocationChange: true });
}
In this example, we use an empty array in the navigate
method to navigate to the current route with the addition of skipLocationChange: true
. This prevents the URL from being updated, giving the illusion of going back to the previous page.
📣 Call-to-Action 📣
Now that you have learned two easy solutions for going back to the last page in Angular 2, it's time to implement it in your own app! Be sure to try out both solutions and see which one works best for your specific use case.
If you encountered any issues or have any other questions, feel free to leave a comment below. We would love to hear from you and help you out!
✨ Remember, navigating through pages should be a seamless and hassle-free experience for your users. By implementing a simple "Go Back" feature, you can greatly improve the usability of your Angular 2 app. Happy coding! ✨