How to force a component"s re-rendering in Angular 2?
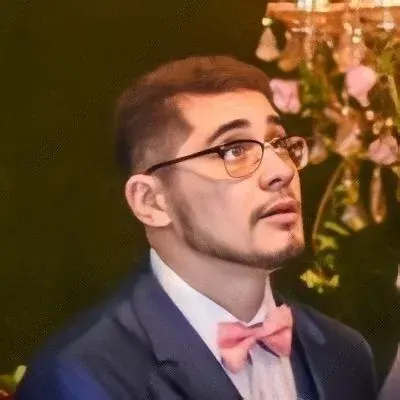
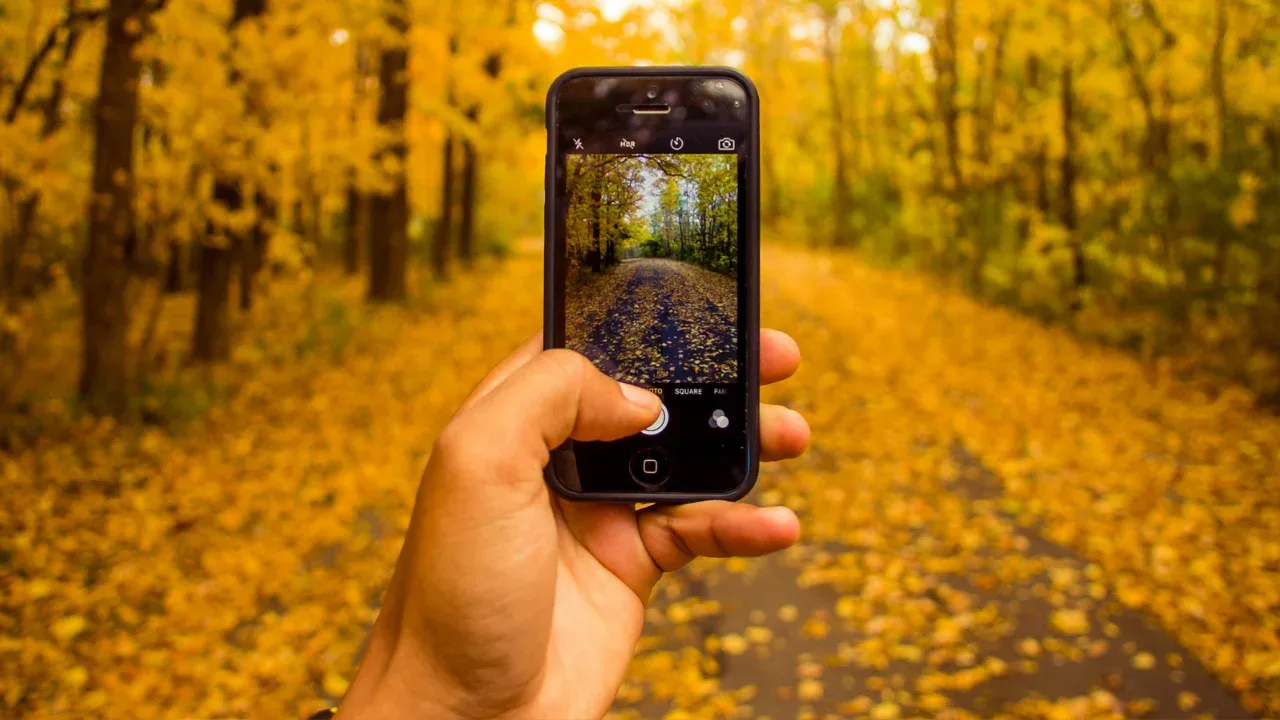
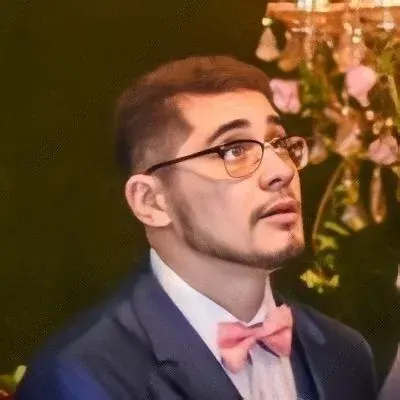
How to Force a Component's Re-rendering in Angular 2? 😎💪
Have you ever found yourself in a situation where you need to force a component to re-render its view in Angular 2? Whether you're working with Redux or for debugging purposes, there are times when you need to update a component's view even if the data hasn't changed. In this blog post, we will explore common issues, provide easy solutions, and help you gain a deeper understanding of how to make it happen.
Why Do You Need to Force a Re-rendering? 🤔
Before we dive into the solutions, let's briefly discuss why you may need to force a component to re-render in the first place. Here are a few common scenarios:
Redux Integration: If you're using Redux or any other state management library, you may need to re-render a component to reflect the updated state changes.
Debugging Purposes: When debugging, it can be useful to force a re-render to ensure that the latest changes are reflected in the component's view.
Now, let's explore some possible solutions to this problem and find out how we can tackle it effectively!
Solution 1: Triggering a Change Detection Cycle 🔄
Angular's change detection mechanism is responsible for updating the component's view whenever there is a change in its data bindings. By triggering a change detection cycle manually, we can force a re-render of the component. Here's how you can achieve this:
import { ChangeDetectorRef } from '@angular/core';
// Inject the ChangeDetectorRef in your component's constructor
constructor(private cdr: ChangeDetectorRef) {}
// Call the detectChanges() method to trigger a change detection cycle
forceRerender() {
this.cdr.detectChanges();
}
In the above example, we import the ChangeDetectorRef
from the @angular/core
package and inject it in the component's constructor. Then, by calling the detectChanges()
method, we force Angular to re-render the component's view.
Solution 2: Using the *ngIf
Directive 📝
Another approach to force a component re-rendering is by leveraging the power of the *ngIf
directive. This directive can be used to conditionally render a component based on a boolean expression. By toggling the flag linked to the *ngIf
directive, we can force Angular to re-render the component. Let's see it in action:
@Component({
selector: 'app-my-component',
template: `
<div *ngIf="shouldRenderComponent">
<!-- Your component's content here -->
</div>
`
})
export class MyComponent {
shouldRenderComponent = true;
// Call this method to force a re-rendering
forceRerender() {
this.shouldRenderComponent = false;
setTimeout(() => {
this.shouldRenderComponent = true;
});
}
}
In the above example, we have a boolean flag shouldRenderComponent
linked to the *ngIf
directive. When we want to force a re-render, we toggle the flag to false
using this.shouldRenderComponent = false
. Then, after a small delay using setTimeout
, we toggle it back to true
to trigger a re-render of the component.
Call-to-Action: Share Your Experience! 🚀📢
We hope these solutions help you force a component's re-rendering in Angular 2. Now, it's your turn! Have you ever encountered the need to force a re-rendering in your Angular 2 projects? How did you tackle it? Share your experience in the comments below and let's keep the conversation going! 💬💡
Remember, debugging and state management are crucial aspects of building robust Angular applications, so don't hesitate to explore new techniques and share your knowledge with the community. Happy coding! 😄👩💻👨💻