How to extend / inherit components?
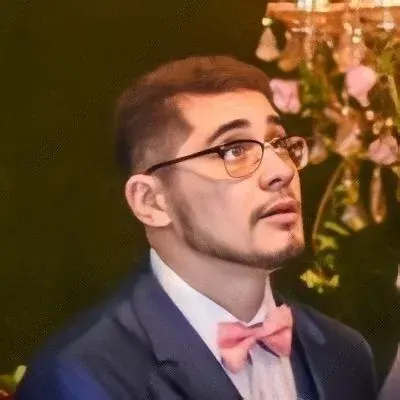
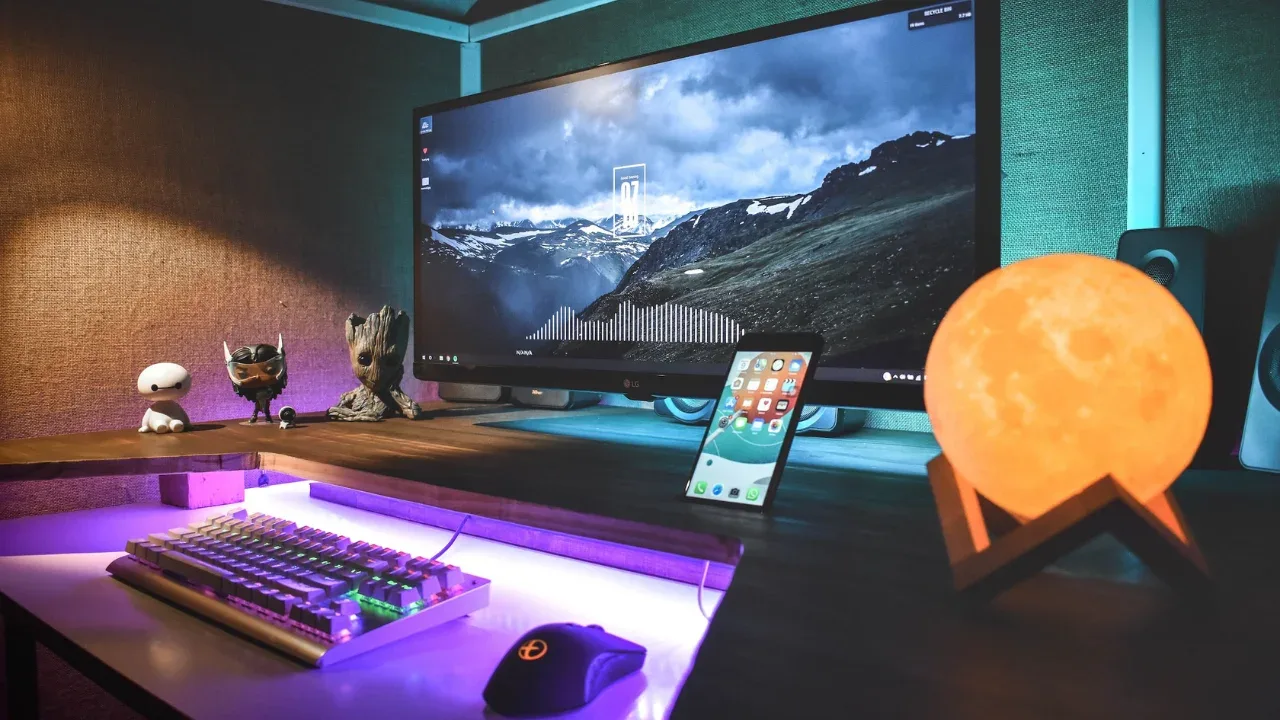
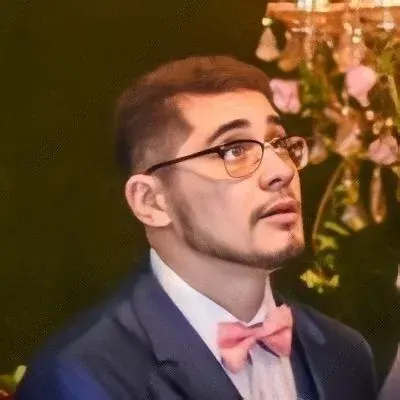
How to Extend/Inherit Angular 2 Components: A Complete Guide 🚀
So you want to create extensions for existing components in Angular 2? You're in luck! In this guide, we'll explore how to extend or inherit components without the need to rewrite them completely. We'll address common issues and provide easy solutions that allow you to make changes to the base component while keeping those changes reflected in the derived components. Let's dive in!
Understanding the Problem
To better understand the problem, let's take a look at a simple example. We have a base component called BasePanelComponent
which has some desired functionality. Now, we want to create a derivative component, MyPanelComponent
, that only alters a basic behavior of the base component (in this case, the color).
import { Component } from 'angular2/core';
import { BasePanelComponent } from './base-panel.component';
@Component({
selector: 'my-panel',
template: '<div class="panel" [style.background-color]="color" (click)="onClick($event)">{{content}}</div>',
styles: [`
.panel {
padding: 50px;
}
`]
})
export class MyPanelComponent extends BasePanelComponent {
constructor() {
super();
this.color = 'blue';
}
}
The issue with this approach is that much of the implementation is repeated in the derivative component. The only part that is truly inherited is the class
definition of BasePanelComponent
. The @Component
decorator has to be completely repeated, even for the unchanged portions.
The Solution
While there is no built-in way to achieve literal full inheritance of components in Angular 2, we can still accomplish our goal by following a few steps:
Create a base component with the desired functionality.
Extend the base component in the derivative component using the
extends
keyword.In the derivative component, use the
super()
method within the constructor to invoke the constructor of the base component.Make the necessary modifications to the derivative component as needed.
Let's modify our example to follow these steps:
Step 1: Create the Base Component
import { Component, Input } from 'angular2/core';
@Component({
selector: 'base-panel',
template: '<div class="panel" [style.background-color]="color" (click)="onClick($event)">{{content}}</div>',
styles: [`
.panel {
padding: 50px;
}
`]
})
export class BasePanelComponent {
@Input() content: string;
color: string = 'red';
onClick(event: any) {
console.log('Click color: ' + this.color);
}
}
Step 2: Extend the Base Component
import { Component } from 'angular2/core';
import { BasePanelComponent } from './base-panel.component';
@Component({
selector: 'my-panel',
template: '<div class="panel" [style.background-color]="color" (click)="onClick($event)">{{content}}</div>',
styles: [`
.panel {
padding: 50px;
}
`]
})
export class MyPanelComponent extends BasePanelComponent {
constructor() {
super();
this.color = 'blue';
}
}
Step 3: Use the Components
Now, you can use both the base component (BasePanelComponent
) and the derivative component (MyPanelComponent
) in your Angular 2 application. For example:
<base-panel [content]="'Base Panel Content'"></base-panel>
<my-panel [content]="'My Panel Content'"></my-panel>
The base-panel
component will have the original functionality, while the my-panel
component will inherit the functionality and have the modified color.
Conclusion
Although Angular 2 doesn't provide native support for literal full inheritance of components, we can still extend or inherit components by following the steps outlined in this guide. By leveraging the extends
keyword, we can reuse functionality while making specific modifications where necessary.
So go ahead and give it a try! Start extending and inheriting components in your Angular 2 applications, and let us know how it goes. And remember, if you encounter any issues or have any questions, feel free to leave a comment below or contact us directly.
Happy coding! 😄✨👩💻
Like this article? Don't forget to share it and let your friends know about this cool feature in Angular 2! If you have any questions or want to share your experiences with extending or inheriting components, leave a comment below. We would love to hear from you! 🗣️💬
Follow us on Twitter for more tech tips and updates: @yourtwitterhandle
😊🙏 Thanks for reading and happy coding! 🎉🚀