How to emit an event from parent to child?
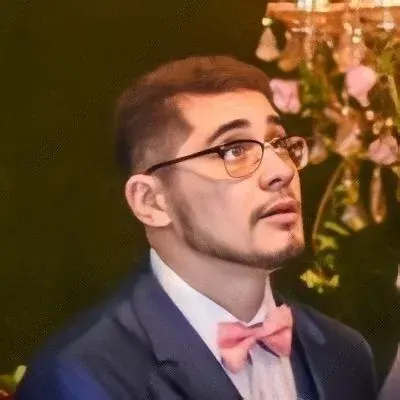
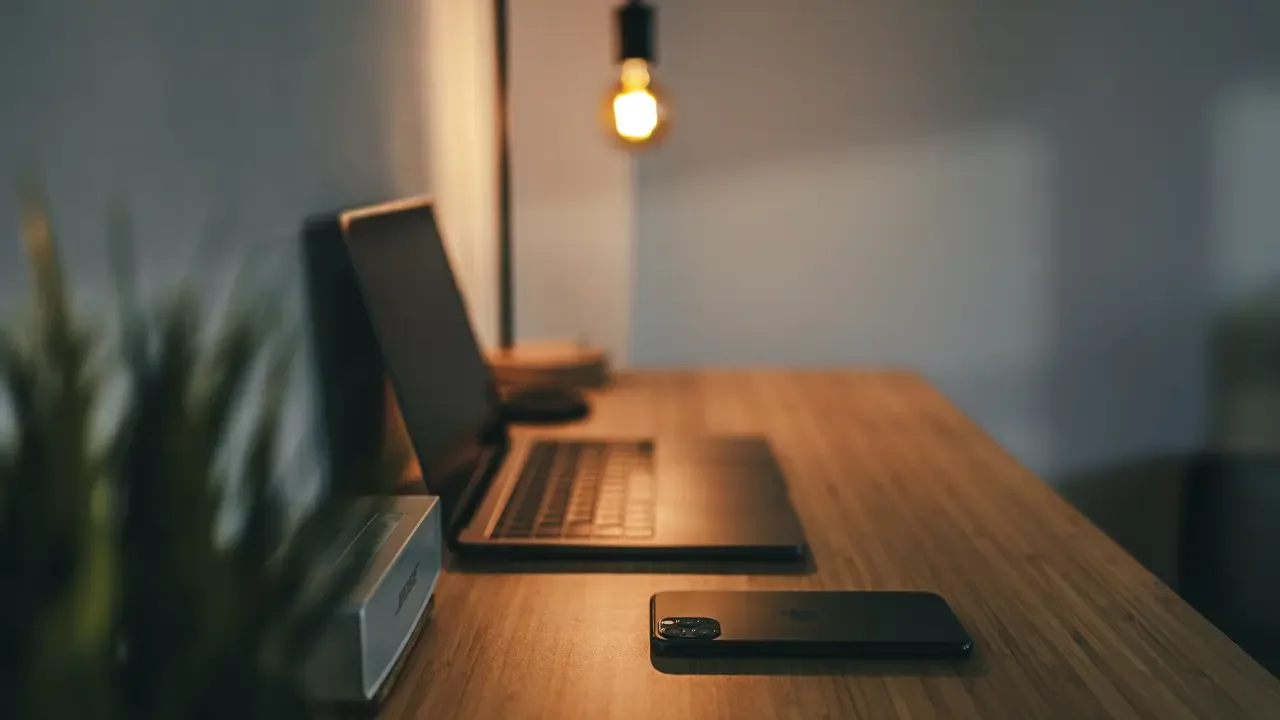
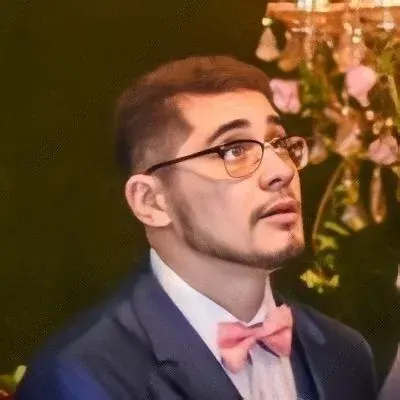
📢 Emitting an Event from Parent to Child in Angular 2 🏃♀️🏃♂️
So you've been using Angular 2 and you're familiar with emitting events from a child component to its parent using @Output()
. But now you find yourself in a situation where you need to emit an event from the parent component to its child. 🤔 Don't worry, we've got you covered! In this blog post, we'll explore how to achieve this reverse event emission in Angular 2. Let's dive right in! 💦
The Scenario 🎬
You have an Angular 2 application, and you're currently working with a parent component and a child component. In your child component, you already know how to emit events to the parent component using @Output()
and EventEmitter
. However, now you want to emit an event from the parent component to the child component. How can you achieve this? 🤷♀️🤷♂️
The Solution 🎉
To emit an event from the parent component to the child component, we can utilize the power of Angular's template reference variable. Here's how you can do it:
In the child component template, define a reference variable using the
#
symbol. For example, let's call itchildCmp
:
<child-component #childCmp></child-component>
In the parent component, use
ViewChild
to access the child component reference variable:
import { ViewChild } from '@angular/core';
// ...
@ViewChild('childCmp') childComponent: ChildComponent;
Now, you can emit an event from the parent component to the child component using the
EventEmitter
from thechildComponent
:
this.childComponent.someEvent.emit(data);
That's it! You've successfully emitted an event from the parent component to the child component using the template reference variable. 🎊
Example 💡
Here's a simple example to illustrate the process. Let's say we have a parent component called ParentComponent
and a child component called ChildComponent
. We want to emit an event from the parent to the child when a button is clicked. Here's how the code would look like:
In the parent component template:
<button (click)="emitEventToChild()">Click me!</button>
<child-component #childCmp></child-component>
In the parent component TypeScript:
import { Component, ViewChild } from '@angular/core';
import { ChildComponent } from './child.component';
@Component({
selector: 'app-parent',
template: `
<button (click)="emitEventToChild()">Click me!</button>
<child-component #childCmp></child-component>
`,
})
export class ParentComponent {
@ViewChild('childCmp') childComponent: ChildComponent;
emitEventToChild() {
this.childComponent.someEvent.emit(data);
}
}
In the child component TypeScript:
import { Component, Output, EventEmitter } from '@angular/core';
@Component({
selector: 'app-child',
template: `
<h3>Child Component</h3>
`,
})
export class ChildComponent {
@Output() someEvent = new EventEmitter<any>();
}
Conclusion ✨
Congratulations! You now know how to emit events from the parent component to the child component in Angular 2. By using template reference variables and ViewChild
, you have a powerful tool at your disposal. Feel free to experiment with this technique and unleash the full potential of your Angular 2 application. 🚀
Now it's up to you, what are you waiting for? Go ahead and apply this knowledge to your own projects. And don't forget to let us know in the comments below if you found this guide helpful or if you have any questions.
Keep coding, keep exploring! 🧑💻🌈
👉 Don't forget to subscribe to our newsletter to stay up-to-date with the latest Angular 2 tips and tricks! 💌
📣 Have you ever emitted an event from a parent component to a child component? Share your experience with us! 💬
✨ Join our thriving community on social media and connect with fellow Angular enthusiasts:
✉️ Do you have a burning question or need further clarification? Feel free to reach out to our friendly support team at support@angularcommunity.com
That's all for now! Stay tuned for more exciting Angular 2 tutorials, tips, and tricks coming your way. Happy coding! 🎉