How to detect when an @Input() value changes in Angular?
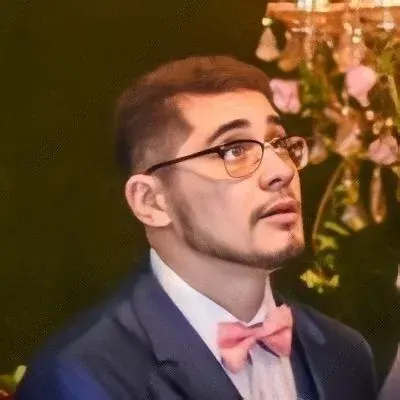
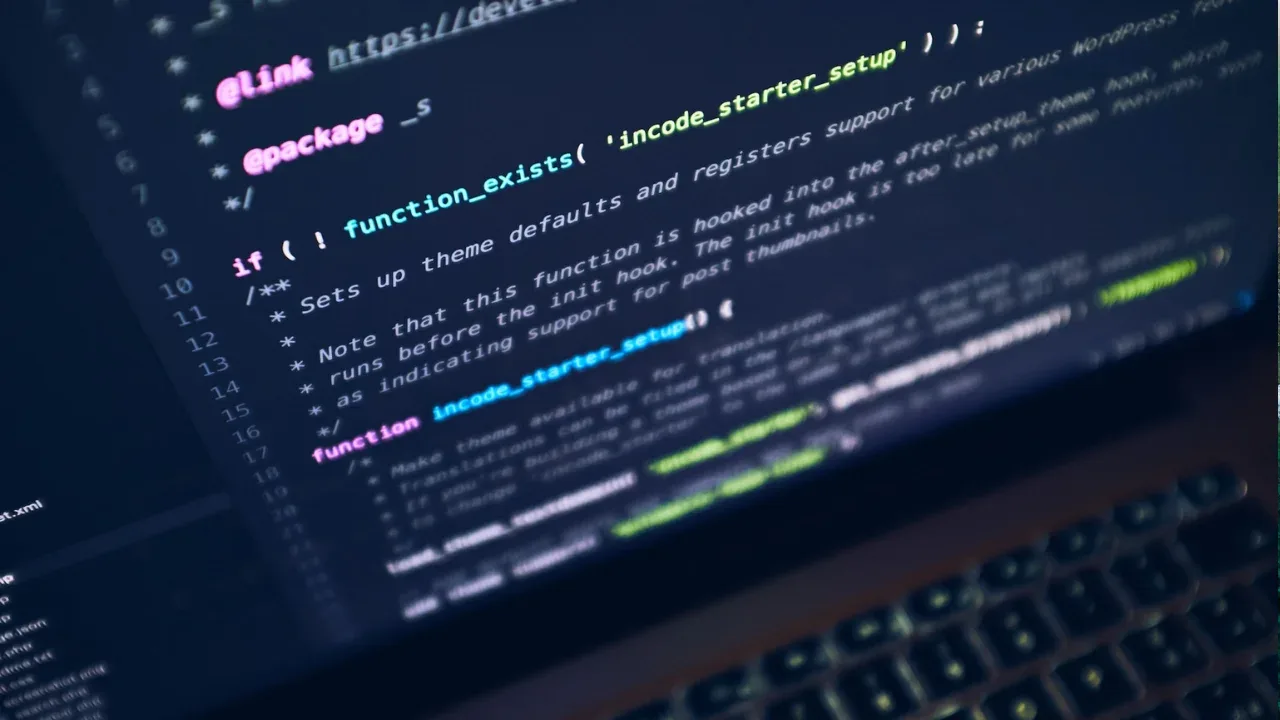
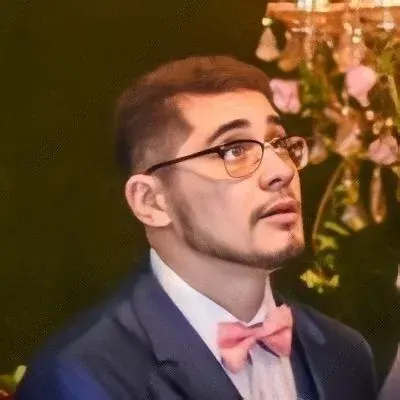
📝 Detecting @Input() value changes in Angular: The Easy Way 🚀
Do you find yourself struggling to detect when an @Input() value changes in Angular? Don't worry, you're not alone! Many Angular developers face this issue when working with parent and child components. But fret not, we've got you covered with some easy solutions. Let's dive in! 💪
The scenario is as follows: you have a parent component called CategoryComponent
, a child component named VideoListComponent
, and an ApiService
. Currently, the VideoListComponent
retrieves all videos, but you want to filter them based on a specific category. To achieve this, you pass the categoryId
from the parent component to the child using the @Input()
decorator.
In CategoryComponent.html
, you have the following code:
<video-list *ngIf="category" [categoryId]="category.id"></video-list>
This setup allows the categoryId
value to be passed to VideoListComponent
whenever the CategoryComponent
's category changes. But now, you need to detect this change in the VideoListComponent
and update the videos array using the new categoryId
. So, how can we achieve that? 🤔
In AngularJS, you might have used $watch
on the variable. However, in Angular, we have a better solution! You can leverage Angular's lifecycle hooks to detect when the @Input()
value changes. Let's use the ngOnChanges
hook to achieve this. Here's how you can do it:
import { Component, Input, OnChanges, SimpleChanges } from '@angular/core';
@Component({
selector: 'video-list',
// ...
})
export class VideoListComponent implements OnChanges {
@Input() categoryId: string; // Assuming categoryId is a string
ngOnChanges(changes: SimpleChanges): void {
if (changes.categoryId && !changes.categoryId.firstChange) {
// Do something when the categoryId value changes
this.fetchVideosByCategoryId(this.categoryId);
}
}
fetchVideosByCategoryId(categoryId: string): void {
// Use the ApiService to fetch videos based on the new categoryId
// Update the videos array
}
// ...
}
By implementing the OnChanges
interface and using the ngOnChanges
hook, you can easily detect when the value of categoryId
changes. The ngOnChanges
method receives a SimpleChanges
object that contains information about the changed properties. In our case, we check if categoryId
is one of the changed properties and if it's not the first change (to avoid unnecessary initializations).
Inside the ngOnChanges
method, you can perform any logic or actions you need when the categoryId
value changes. In our example, we call the fetchVideosByCategoryId
method, passing the new categoryId
value, and update the videos
array using the ApiService
.
With this simple approach, you can effortlessly detect @Input() value changes and take the necessary actions. Happy coding! 💻✨
If you found this guide helpful, don't hesitate to share it with your fellow Angular developers! And don't forget to leave a comment below sharing your thoughts or any other solutions you've found. Let's continue the conversation! 🎉
🚀 Take your Angular skills to the next level! 🚀
Are you hungry to learn more about Angular and become a pro? Check out our latest e-book: "Mastering Angular: Advanced Techniques for Building Amazing Web Applications." 📚🔥
In this comprehensive guide, you'll uncover expert tips, hidden gems, and advanced concepts that will skyrocket your Angular skills. From component communication to state management, we've got you covered! Don't miss out on this valuable resource. Get your copy today and level up your Angular game! 📖💪