How to detect a route change in Angular?
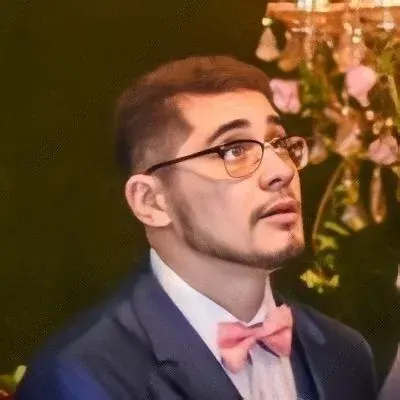
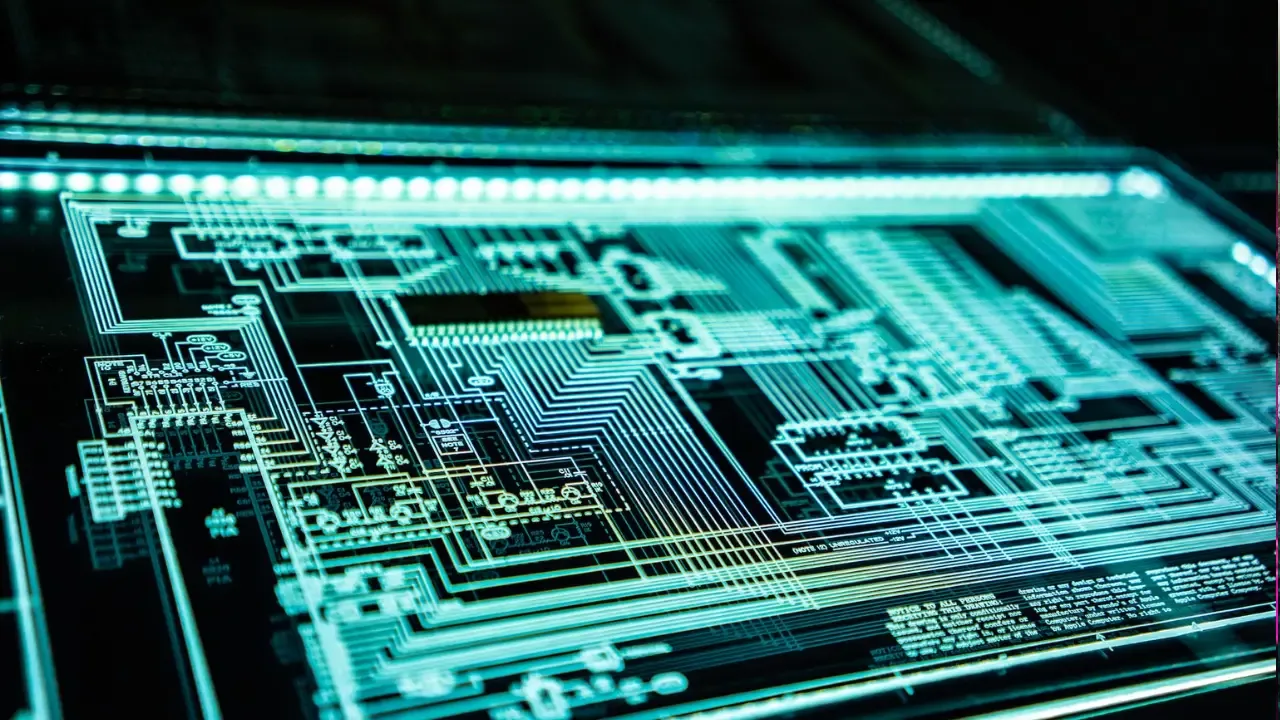
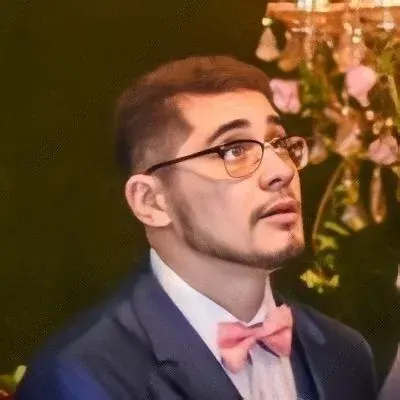
How to detect a route change in Angular?
So, you want to detect a route change in your Angular app's AppComponent
? No worries, I've got you covered! 🕵️♂️
It seems like you're looking to ensure that your user is redirected if they're not logged in. That's a common scenario, and luckily, Angular provides us with a straightforward solution. Let's explore it together! 💪
First things first, we need to import the Router
from @angular/router
in our AppComponent
:
import { Router, NavigationEnd } from '@angular/router';
Next, we'll inject the Router
into our AppComponent
constructor:
constructor(private router: Router) { }
Now, let's subscribe to the router.events
and listen for NavigationEnd
events. These events occur whenever the route changes:
ngOnInit() {
this.router.events.subscribe(event => {
if (event instanceof NavigationEnd) {
// Route has changed, do something here
}
});
}
Within the NavigationEnd
event callback, we can perform our desired actions. In your case, you mentioned redirecting the user if they're not logged in. Let's assume you have a AuthService
to handle authentication:
import { AuthService } from './auth.service';
constructor(private router: Router, private authService: AuthService) { }
ngOnInit() {
this.router.events.subscribe(event => {
if (event instanceof NavigationEnd) {
if (!this.authService.isLoggedIn()) {
// User is not logged in, redirect them
this.router.navigate(['/login']);
}
}
});
}
Here, we're checking if the user is not logged in using the isLoggedIn()
method of our AuthService
. If they're not logged in, we redirect them to the login page using router.navigate(['/login'])
.
And voila! 🎉 You've successfully detected a route change and handled the scenario of redirecting the user if they're not logged in.
Remember to import the necessary modules and services and ensure that the routes are properly configured in your Angular app. If you face any difficulties, feel free to drop a comment below or consult the Angular documentation.
Now, it's time for you to implement this solution in your app! Give it a shot and let me know how it goes. Happy coding! 💻
If you found this guide helpful, don't forget to share it with your fellow Angular developers! Sharing is caring, after all. 🤗
Have a different scenario in mind or any other Angular issues you'd like me to cover? Let me know in the comments below. Your feedback helps me create content that caters to your needs.
Keep exploring, keep learning, and keep building amazing Angular apps! 🚀