How to apply filters to *ngFor?
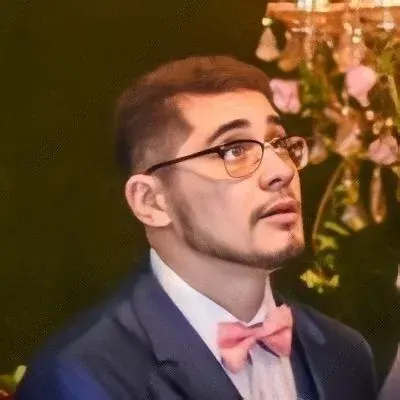
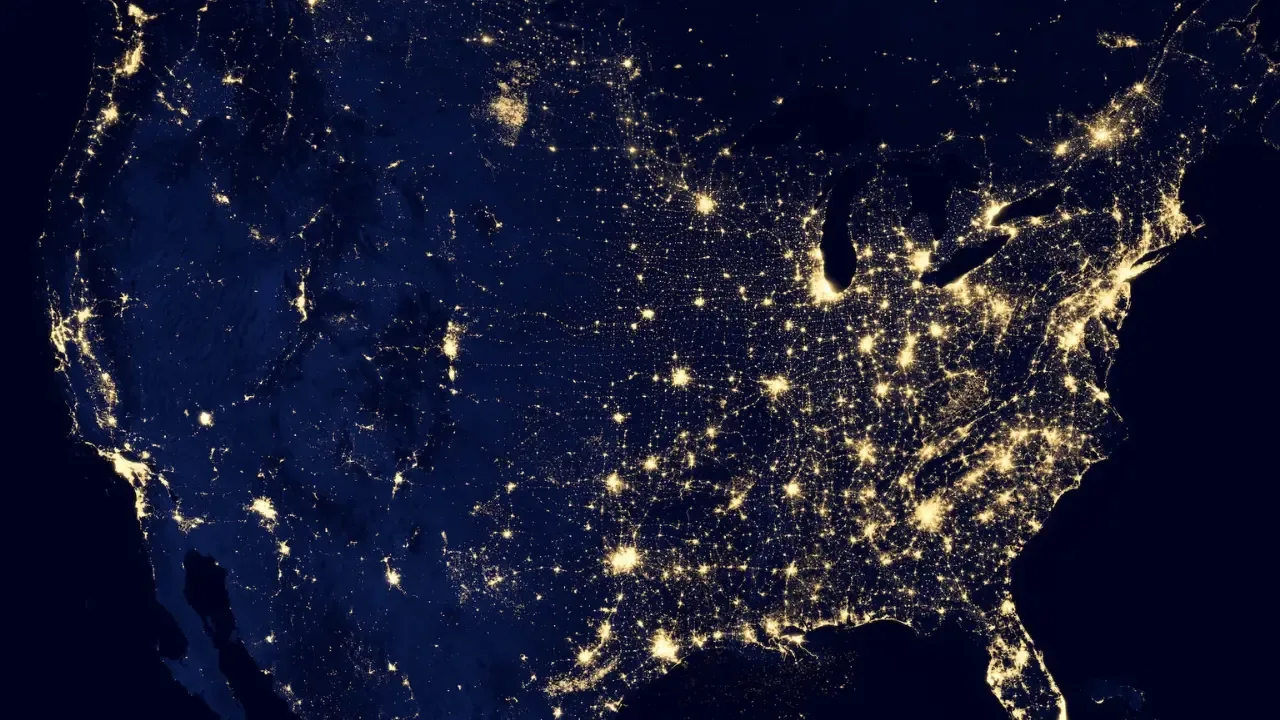
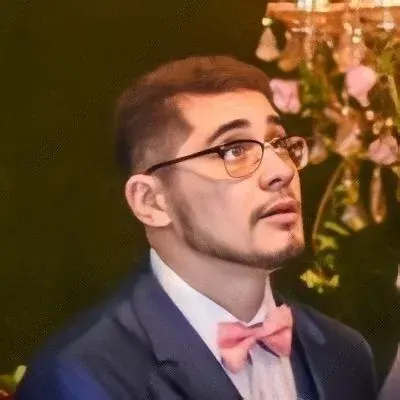
How to Apply Filters to *ngFor? ๐กโจ
So you want to apply filters to your *ngFor loop in Angular 2? You're in the right place! In Angular 2, instead of using filters like in Angular 1, pipes are used in conjunction with *ngFor to filter the results. Although the documentation may be a bit unclear, don't worry โ I've got you covered! In this guide, I'll walk you through the process of applying filters to *ngFor and provide easy solutions to common issues you may face. Let's dive in! ๐
The Context ๐
Before we jump into the solutions, let's quickly understand the context:
<div *ng-for="let item of itemsList" *ng-if="condition(item)"></div>
In the above example, we want to iterate over itemsList
using *ngFor, but we also want to apply a condition to filter the results. To achieve this, we need to use pipes. Let's see how! ๐ช
Solution 1: Using the Built-in Filter Pipe ๐งฐ
Angular 2 provides a built-in pipe called filter
that you can use to apply filters to *ngFor. Here's how you can do it:
<div *ng-for="let item of itemsList | filter: condition"></div>
In the above code snippet, we've added | filter: condition
after itemsList
. This tells Angular 2 to apply the filter
pipe with the condition
as the filter criteria. Pretty neat, right?
Solution 2: Creating a Custom Filter Pipe ๐ ๏ธ
If the built-in filter
pipe doesn't fulfill your needs, you can create a custom filter pipe. Creating a custom pipe allows you to have full control over the filtering logic. Let me show you how it's done:
Create a new file called
filter.pipe.ts
and add the following code:
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'filter'
})
export class FilterPipe implements PipeTransform {
transform(items: any[], condition: any): any {
// Filtering logic goes here
}
}
In your component file, import and declare the custom pipe:
import { FilterPipe } from './filter.pipe';
@Component({
// Component metadata goes here
})
export class YourComponent {
constructor(private filterPipe: FilterPipe) {}
// Rest of your component code
}
Finally, implement the filtering logic in the
transform
method infilter.pipe.ts
:
transform(items: any[], condition: any): any {
// Filtering logic goes here
return items.filter(item => condition(item));
}
Now you can use your custom filter pipe in the template:
<div *ng-for="let item of itemsList | filter: condition"></div>
Conclusion ๐
Congratulations! You've learned two ways to apply filters to *ngFor in Angular 2. You can either use the built-in filter
pipe or create a custom filter pipe to have full control over the filtering logic. Now it's your turn to give it a try! If you encounter any issues or have any questions, feel free to leave a comment below. Happy coding! ๐ปโจ
Liked this guide? Don't forget to share it with your fellow developers! If you found it helpful, be sure to leave a comment and let me know your thoughts. I'd love to hear your feedback and engage in a discussion with the community. Until next time, happy filtering! ๐