How to add bootstrap to an angular-cli project
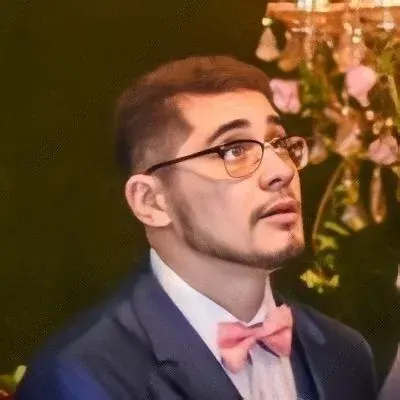
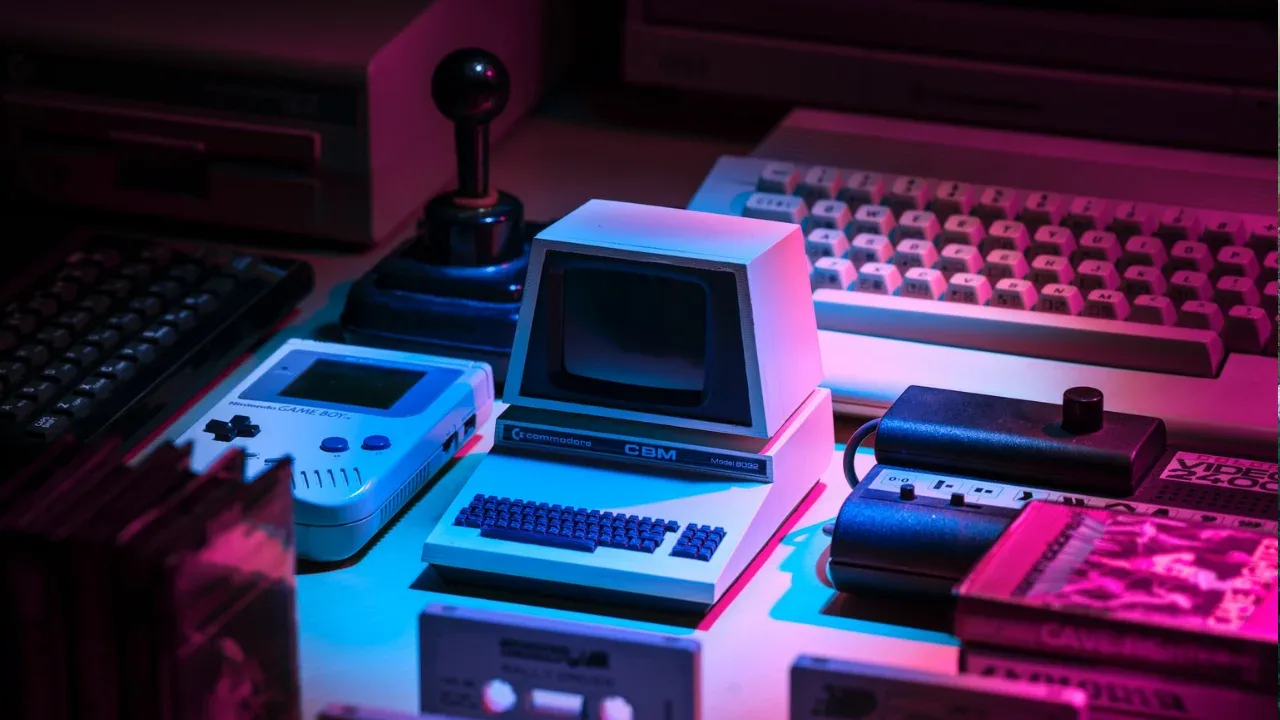
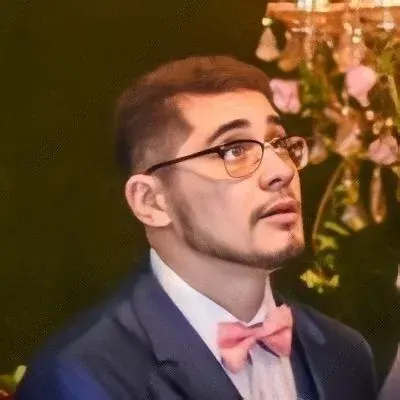
How to Add Bootstrap to an Angular-CLI Project
So, you want to add Bootstrap 4 to your Angular-CLI project? Great choice! Bootstrap is a popular CSS framework that can make your web app look sleek and professional. However, adding Bootstrap to an Angular-CLI project might not be as straightforward as you initially thought. But fear not, I'm here to guide you through the process. Let's get started!
The Problem
When you initially added Bootstrap and its dependencies to your project's angular-cli-build.js
file and imported them in your index.html
file, everything seemed to work fine when using ng serve
- your app looked beautiful. However, when you tried to produce a build using the ng build -prod
command, all those Bootstrap dependencies disappeared from the dist/vendor
folder. What's the deal?
The Solution
Option 1: Use a CDN
Using a Content Delivery Network (CDN) is a quick and easy way to include the Bootstrap CSS and JavaScript files in your project. Bootstrap is available on popular CDNs like BootstrapCDN, which hosts the latest versions of Bootstrap. Here's how you can use a CDN to include Bootstrap in your Angular-CLI project:
Open your
index.html
file.Remove the previous script and link tags that were pointing to the local Bootstrap files.
Add the following lines inside the
<head>
tag:
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.0.0-alpha.2/css/bootstrap.min.css">
<script src="https://code.jquery.com/jquery-3.2.1.slim.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/tether/1.4.0/js/tether.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.0.0-alpha.2/js/bootstrap.min.js"></script>
⚠️ Important Note:
Using a CDN means your app's availability depends on the availability of Bootstrap files from the CDN. If the CDN is down, your app won't look as expected. Moreover, it's always better to serve these files from your app's assets to ensure they are available even if the CDN is down.
Option 2: Copy Dependencies to dist/vendor
If you prefer serving the Bootstrap files from your app's assets and want to keep these files locally, you can manually copy the Bootstrap dependencies to the dist/vendor
folder after building your project. Here's how you can do it:
Open your
angular-cli.json
file.Add the following lines under the
apps
section:
"assets": [
"assets",
"vendor"
]
Open your terminal and navigate to your project's root folder.
Run the following command:
cp -R node_modules/bootstrap/dist/ dist/vendor/bootstrap
cp -R node_modules/jquery/dist/ dist/vendor/jquery
cp -R node_modules/tether/dist/ dist/vendor/tether
Option 3: Import Dependencies in src/system-config.ts
If you want to include Bootstrap in your project's bundle and avoid external dependencies, you can add the Bootstrap, jQuery, and Tether files to your src/system-config.ts
file. Although this might seem counterintuitive since you're not explicitly using Bootstrap in your application's code, it ensures that the required files are bundled. Here's how you can do it:
Open your
src/system-config.ts
file.Add the following lines at the beginning of the file:
declare var jQuery: any;
declare var Tether: any;
let map: any = {
// other mappings...
'bootstrap': 'vendor/bootstrap/dist/js/bootstrap.min.js',
'jquery': 'vendor/jquery/dist/jquery.min.js',
'tether': 'vendor/tether/dist/js/tether.min.js'
};
let packages: any = {
// other packages...
'bootstrap': { main: 'bootstrap.min.js', defaultExtension: 'js' },
'jquery': { main: 'jquery.min.js', defaultExtension: 'js' },
'tether': { main: 'js/tether.min.js', defaultExtension: 'js' }
};
let barrels: string[] = [
// other barrels...
'bootstrap',
'jquery',
'tether'
];
barrels.forEach((barrelName: string) => {
packages[barrelName] = { main: 'index.js', defaultExtension: 'js' };
});
let config: any = {
// your config...
map,
packages
};
System.config(config);
Open your
src/main.ts
file.Add the following lines at the top of the file:
import 'bootstrap';
import 'jquery';
import 'tether';
Conclusion
Adding Bootstrap to an Angular-CLI project requires a bit of configuration but is totally doable! In this article, we explored three different ways to add Bootstrap to your project: using a CDN, copying dependencies to the dist/vendor
folder, and importing the files in src/system-config.ts
. Choose the method that best fits your needs and give your app that stunning Bootstrap look!
Got any questions or other methods to share? Let me know in the comments below! And don't forget to share this article with your fellow Angular developers. Happy coding! 🚀