How do I pass data to Angular routed components?
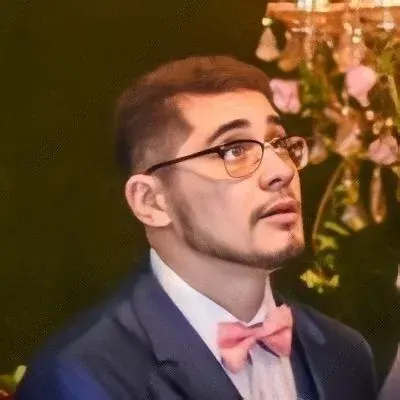
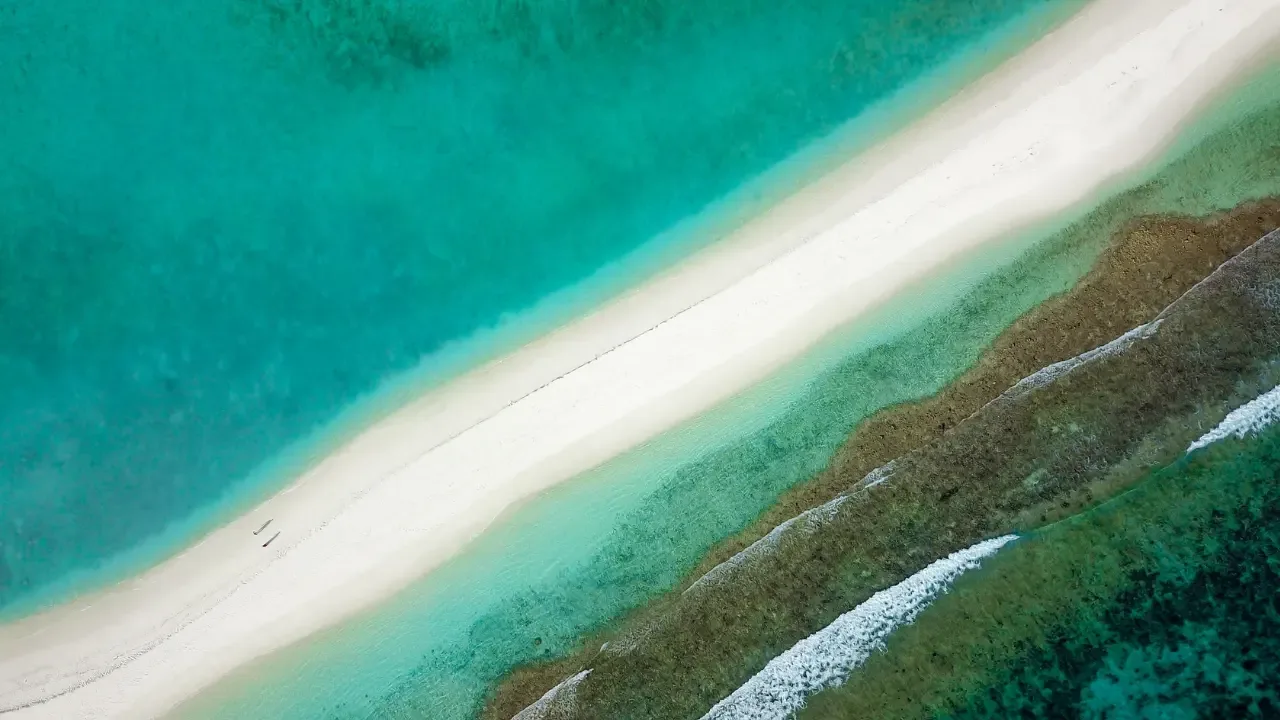
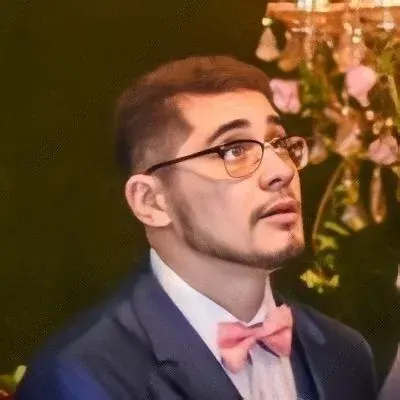
How to Pass Data to Angular Routed Components
Are you struggling with passing data between Angular routed components? 😫 Don't worry, we've got you covered! In this guide, we'll walk you through common issues and provide easy solutions.
The Problem
Let's start with a common scenario. You have a button in your FirstComponent
template, and when clicked, you want to route to another component (SecondComponent
) while preserving data. But how can you achieve this without using the other component as a directive? 🤔
Approach 1 - URL Parameters
One approach is to pass the data through URL parameters. You can use the Angular Router
service to navigate to SecondComponent
with the data. Here's how you can do it:
Define the link with parameters in your route configuration:
@RouteConfig([
// ...
{ path: '/SecondComponent/:p1:p2', name: 'SecondComponent', component: SecondComponent}
)]
In your
FirstComponent
, store the data in properties:
export class FirstComponent {
constructor(private _router: Router) { }
property1: number;
property2: string;
property3: TypeXY;
routeWithData(){
this._router.navigate(['SecondComponent', {p1: this.property1, p2: this.property2 }]);
}
}
However, this approach has limitations when it comes to complex data objects like property3
. URL parameters are designed for simple data types, not objects. 😕
Approach 2 - Using a Directive
Another alternative is to include SecondComponent
as a directive in FirstComponent
. Although this approach allows you to pass complex data objects, it doesn't fulfill your requirement of routing to the component.
Approach 3 - The Elegant Solution
The most elegant solution to this problem is to use a service to store and retrieve the data between components. Let's call this service FirstComponentService
. Here's how you can implement it:
Create
FirstComponentService
to store and retrieve the data:
@Injectable()
export class FirstComponentService {
private data: any;
storeData(data: any) {
this.data = data;
}
retrieveData() {
return this.data;
}
}
In your
FirstComponent
, import and inject the service. Store the data usingstoreData()
method:
export class FirstComponent {
constructor(private _router: Router, private _firstComponentService: FirstComponentService) { }
routeWithData(){
// Store the data
this._firstComponentService.storeData({p1: this.property1, p2: this.property2});
// Navigate to SecondComponent
this._router.navigate(['SecondComponent']);
}
}
In your
SecondComponent
, import and inject the service. Retrieve the data in thengOnInit()
method:
export class SecondComponent {
constructor(private _firstComponentService: FirstComponentService) { }
ngOnInit() {
// Retrieve the data
const data = this._firstComponentService.retrieveData();
// Use the data as needed
console.log(data.p1, data.p2);
}
}
By using this approach, you can easily pass complex data between components without relying on URL parameters or including components as directives. Isn't it cool? 😎
Have More Ideas?
If you have any other potential approaches to pass data between components with the least amount of code, we would love to hear from you! Share your ideas by leaving a comment below.
Now that you've learned how to pass data between Angular routed components, give it a try in your own projects! Happy coding! 🚀