How can I use/create dynamic template to compile dynamic Component with Angular 2.0?
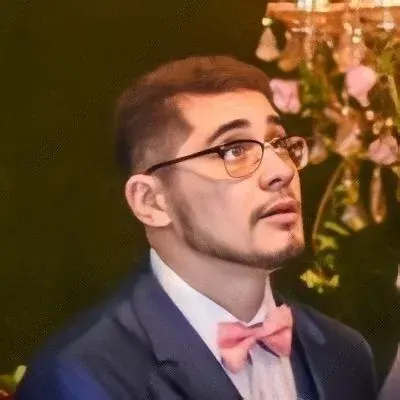
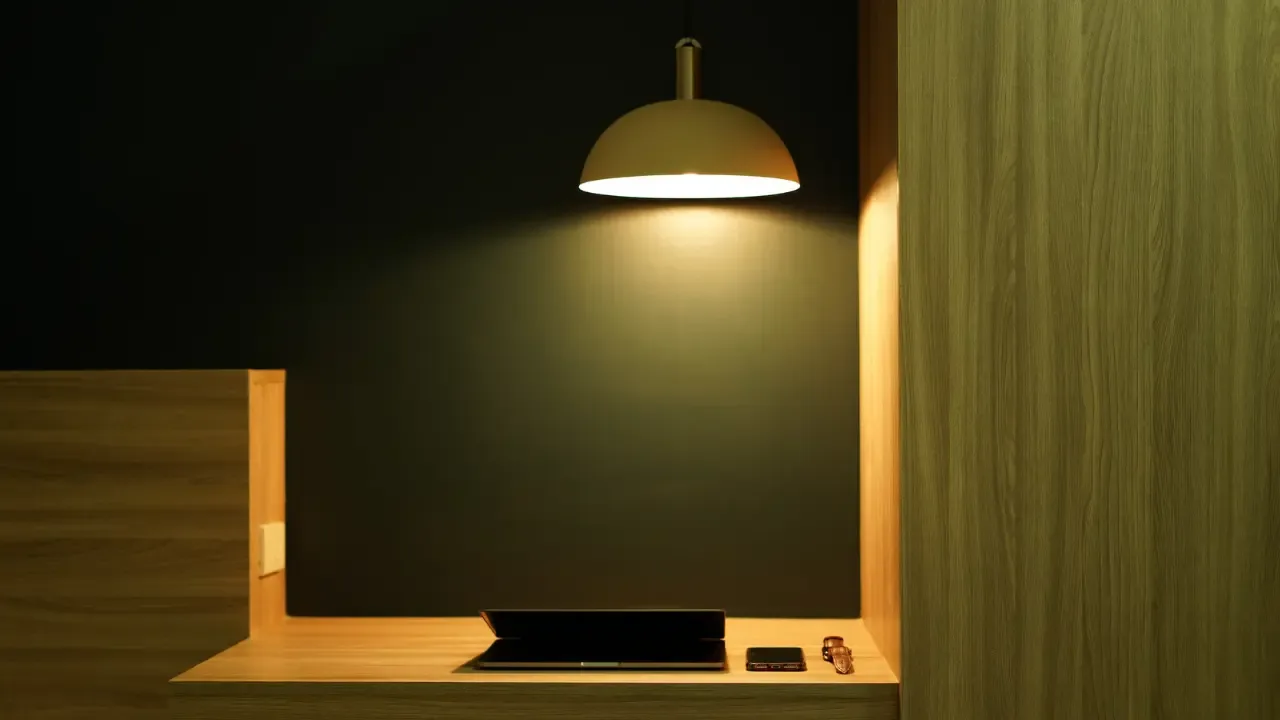
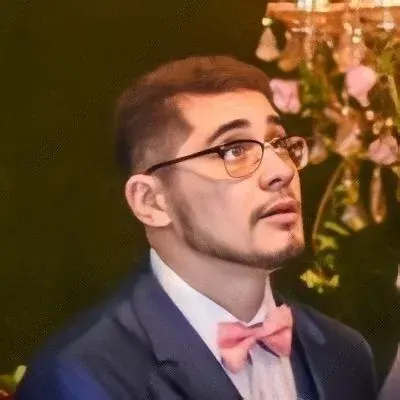
How to Use/Create Dynamic Templates with Angular 2.0
So you want to create dynamic templates with Angular 2.0, huh? 🤔 That's a cool challenge! But don't worry, I'm here to help you out! 😎 In this blog post, I will guide you through the process of using and creating dynamic templates using Angular 2.0.
The Problem: Dynamic Template Creation
First things first, let's address the problem at hand. You want to dynamically create a template that can be used to build a ComponentType
at runtime. This template should also be able to be placed or even replaced within the hosting Component. 📝
However, you may be facing issues with your previous approach, as the ComponentResolver
you were using has been deprecated since RC5. 😱 But fear not, my friend! I have a solution for you!
The Solution: ComponentFactoryResolver and Compiler
With the deprecation of ComponentResolver
, Angular 2.0 now provides two alternatives: ComponentFactoryResolver
and Compiler
. Let's dive into each of these options. 💪
Option 1: ComponentFactoryResolver
👉 If you want to dynamically create components based on known components, you can use ComponentFactoryResolver
alongside entryComponents
configuration within the @Component
decorator.
First, define your known components in the
entryComponents
array:
@Component({
entryComponents: [comp1, comp2],
...
})
Now, you can create components dynamically using
ComponentFactoryResolver
like this:
const componentFactory = componentFactoryResolver.resolveComponentFactory(componentToRender);
const componentRef = componentFactory.create(injector);
This way, you can programmatically create and render components based on your configuration. 🎉
Option 2: Compiler
👉 If you need runtime compilation and want more flexibility, you can use Compiler
to dynamically compile components. Here's how to do it:
First, import the
Compiler
module:
import { Compiler } from '@angular/core';
Next, you can compile components synchronously or asynchronously using the
compileComponentSync
orcompileComponentAsync
methods. For example:
const compiledComponent = compiler.compileComponentSync(template);
template
is an instance of ComponentMetadata
or ComponentFactory
. You can specify your desired template configuration based on your conditions.
Once the compilation is done, you can use the compiledComponent
to create and render your dynamic component. 🚀
Example: Creating Dynamic Templates
Now, let's see an example to make things clearer. Say you want to create different templates based on some configuration conditions.
<!-- Template 1 -->
<form>
<string-editor
[propertyName]="'code'"
[entity]="entity"
></string-editor>
<string-editor
[propertyName]="'description'"
[entity]="entity"
></string-editor>
...
</form>
<!-- Template 2 -->
<form>
<text-editor
[propertyName]="'code'"
[entity]="entity"
></text-editor>
...
</form>
In this example, the template changes based on conditions. You have different editors
(e.g., string-editor
, text-editor
) depending on the property types. Some properties might be skipped for certain users. 😮
To address this dynamic template creation, you can leverage the power of Compiler
. With the appropriate configuration, you can generate various complex templates based on your needs. 🎨
Wrapping Up and Taking Action
Creating dynamic templates with Angular 2.0 can be both challenging and exciting. But with the help of ComponentFactoryResolver
and Compiler
, you can overcome these challenges and create amazing dynamic templates for your application. 🌟
So, what are you waiting for? Start experimenting with these techniques and unleash your creativity! If you have any questions or need further guidance, feel free to reach out to me in the comments below. Let's build some awesome dynamic templates together! 💪😊