Error when trying to inject a service into an angular component "EXCEPTION: Can"t resolve all parameters for component", why?
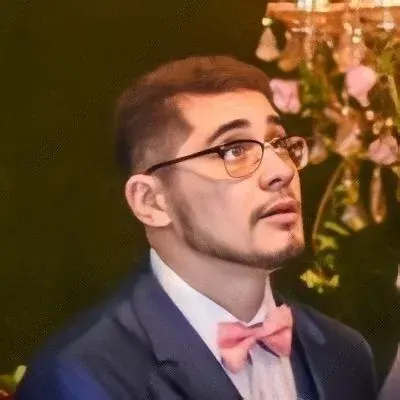
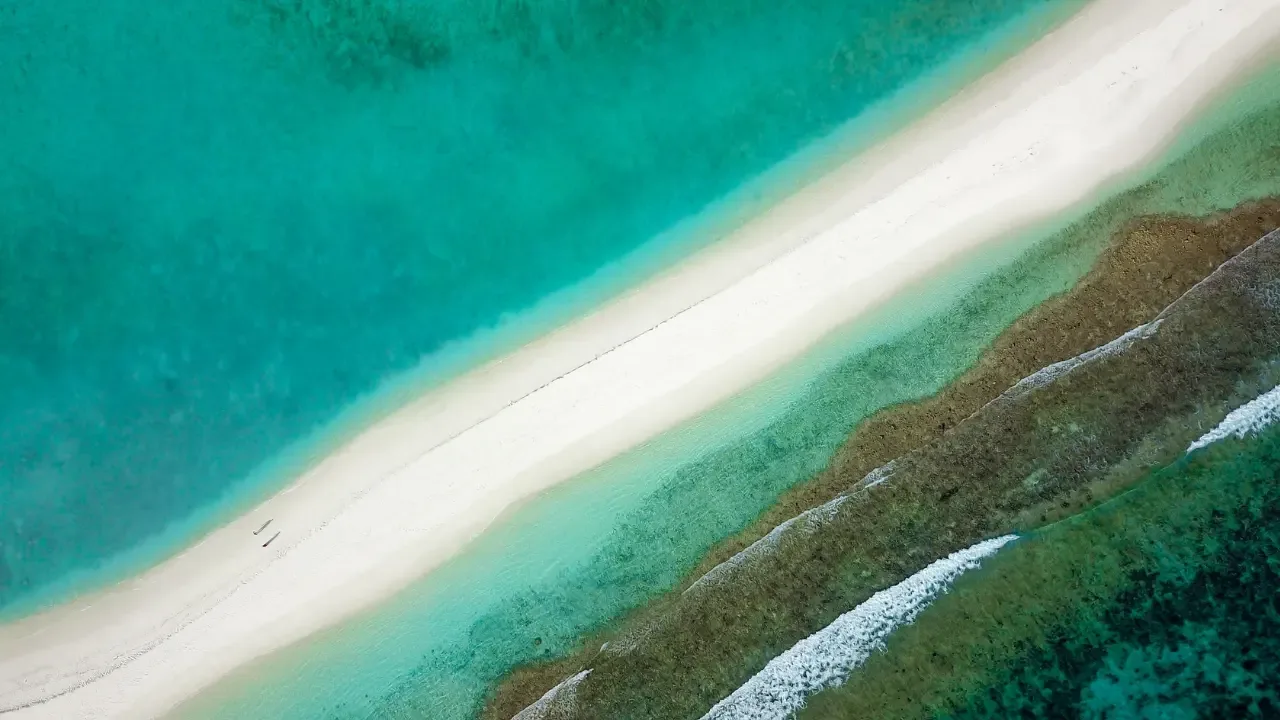
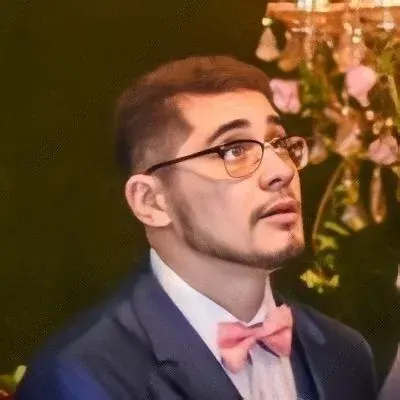
Error when trying to inject a service into an angular component "EXCEPTION: Can't resolve all parameters for component", why?
š Have you encountered this strange issue where you cannot inject a service into one of your Angular components? But it injects fine into your other components? š± Don't worry, you're not alone! Many developers have faced this issue, but luckily, there are easy solutions to tackle it. Let's dive in and resolve this problem together! šŖ
First, let's take a look at the service that you're trying to inject into your component:
import { Injectable } from '@angular/core';
@Injectable()
export class MobileService {
screenWidth: number;
screenHeight: number;
constructor() {
this.screenWidth = window.outerWidth;
this.screenHeight = window.outerHeight;
window.addEventListener("resize", this.onWindowResize.bind(this));
}
onWindowResize(ev: Event) {
var win = ev.currentTarget as Window;
this.screenWidth = win.outerWidth;
this.screenHeight = win.outerHeight;
}
}
This service looks fine, so let's move on to the component that's giving you the trouble:
import { Component } from '@angular/core';
import { NgClass } from '@angular/common';
import { ROUTER_DIRECTIVES } from '@angular/router';
import { MobileService } from '../';
@Component({
moduleId: module.id,
selector: 'pm-header',
templateUrl: 'header.component.html',
styleUrls: ['header.component.css'],
directives: [ROUTER_DIRECTIVES, NgClass],
})
export class HeaderComponent {
mobileNav: boolean = false;
constructor(public ms: MobileService) {
console.log(ms);
}
}
When trying to inject the MobileService
into the HeaderComponent
, you encounter the following error in the browser console:
EXCEPTION: Can't resolve all parameters for HeaderComponent: (?).
This error usually occurs when Angular can't figure out how to provide the necessary dependencies for your component. In this case, it's having trouble resolving the MobileService
parameter in the HeaderComponent
constructor.
To fix this, make sure to check the following:
1. Ensure proper import
Double-check if you have imported the MobileService
correctly in your component file. Ensure that the relative path is correct, and the file extension matches the actual file.
2. Provider configuration
Make sure that you have provided the MobileService
properly in your Angular module or component provider configuration. For example, in your app.module.ts
file:
import { MobileService } from './path-to-mobile-service';
@NgModule({
// ...
providers: [MobileService],
// ...
})
export class AppModule { }
3. Multiple instances of the service
Check if you are unintentionally providing multiple instances of the MobileService
in your application. Having multiple instances can sometimes confuse Angular and lead to unresolved parameter errors.
4. Circular dependency
In rare cases, a circular dependency between your components and services may cause this error. Analyze your code and make sure there are no circular dependencies present.
After checking these possible issues, give your application another try. Hopefully, one of these solutions will resolve the problem for you. š¤
Remember, it's always a good practice to keep an eye on your browser console for any other error messages or warnings that might give you further insights into the issue.
If you're still facing trouble or have any other Angular-related questions, feel free to reach out! Let's tackle Angular problems together. š
Thank you for reading! š Consider sharing this post with other Angular developers who might be facing similar issues. And don't forget to leave a comment below if you found this post helpful or have any suggestions. Let's keep learning and growing together! š