Delegation: EventEmitter or Observable in Angular
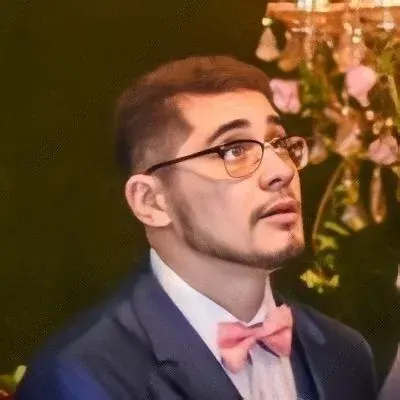
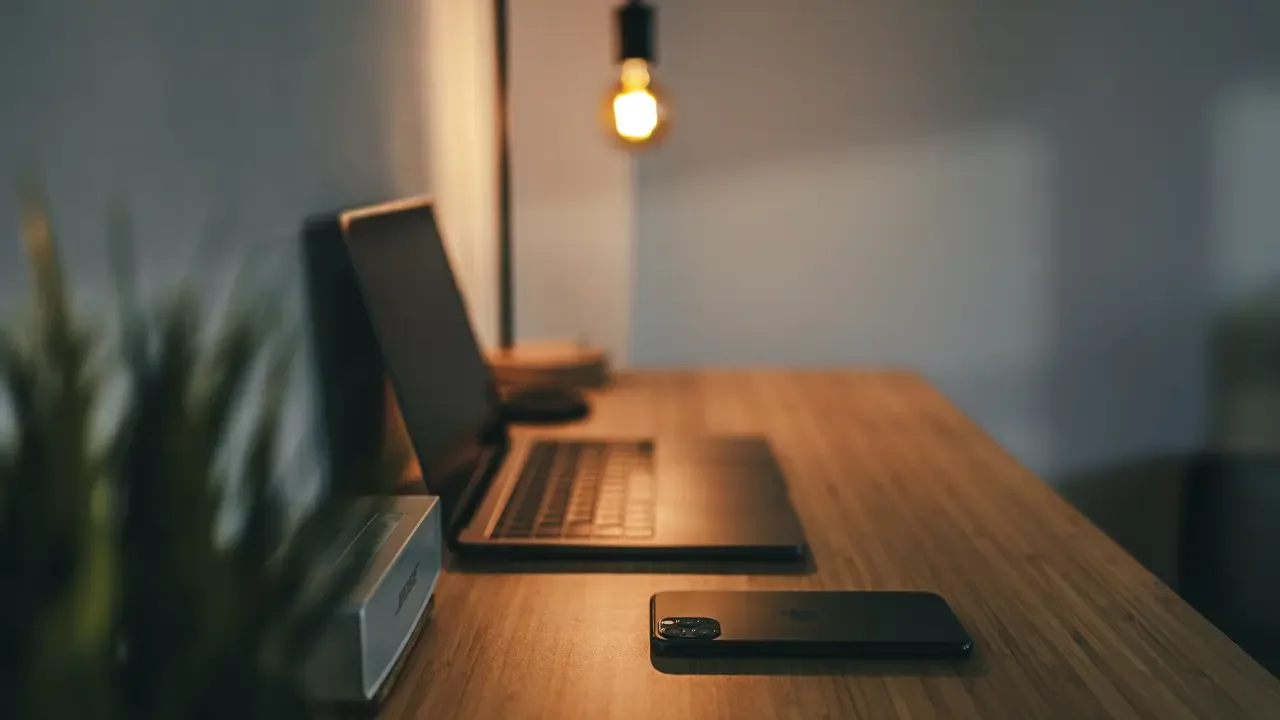
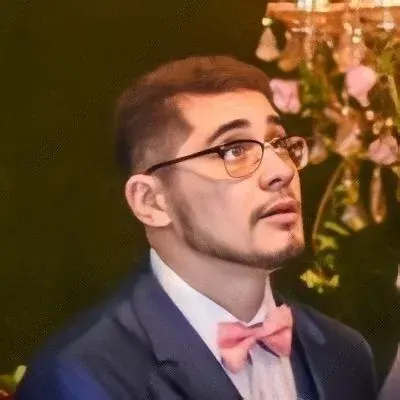
Delegation: EventEmitter or Observable in Angular? 🤔
Are you trying to implement a delegation pattern in your Angular project? Do you want to emit an event from one component and handle it in another component? 🚀🔗
Well, you've come to the right place! In this blog post, we'll explore two common approaches for achieving this: using EventEmitter
or Observable
. We'll address the common issues people encounter and provide easy solutions for them. 💡🛠️
The Setup 🖥️
Let's set the context: you have a Navigation
component and an ObservingComponent
. When the user clicks on a nav-item
in the Navigation
component, you want to emit an event and handle it in the ObservingComponent
. Easy enough, right?
Here's how your code might look:
import { Component, Output, EventEmitter } from 'angular/core';
@Component({
// other properties left out for brevity
template: `
<div class="nav-item" (click)="selectedNavItem(1)"></div>
`
})
export class Navigation {
@Output() navchange: EventEmitter<number> = new EventEmitter();
selectedNavItem(item: number) {
console.log(`selected nav item ${item}`);
this.navchange.emit(item);
}
}
export class ObservingComponent {
// How do I observe the event?
// <----------Observe/Register Event?-------->
selectedNavItem(item: number) {
console.log('item index changed!');
}
}
Approach 1: Using EventEmitter ⚡💡
In Angular, EventEmitter
is a powerful tool for communication between components. To make the ObservingComponent
listen for the event emitted by the Navigation
component, follow these steps:
Import the
EventEmitter
andOutput
decorators from@angular/core
in theObservingComponent
:
import { Component, EventEmitter, Output } from '@angular/core';
Decorate a method with the
@Output
decorator in theNavigation
component:
@Output() navchange: EventEmitter<number> = new EventEmitter();
Emit the event in the
selectedNavItem
method of theNavigation
component:
this.navchange.emit(item);
In the
ObservingComponent
, create an instance of theNavigation
component and subscribe to thenavchange
event:
import { Navigation } from './navigation.component';
export class ObservingComponent {
navigationComponent = new Navigation();
ngOnInit() {
this.navigationComponent.navchange.subscribe((item: number) => {
this.selectedNavItem(item);
});
}
selectedNavItem(item: number) {
console.log('item index changed!');
}
}
With this approach, you can observe the event emitted by the Navigation
component in the ObservingComponent
using EventEmitter
. 🎉🙌
Approach 2: Using Observable 🌈🔭
Alternatively, you can use the power of RxJS Observables to handle the event delegation. Here's how to do it:
Import the necessary operators and the
Subject
class fromrxjs/Rx
in theNavigation
component:
import { Subject } from 'rxjs/Subject';
import 'rxjs/add/operator/filter';
import 'rxjs/add/operator/share';
Replace the
EventEmitter
with aSubject
in theNavigation
component:
export class Navigation {
private navchangeSubject: Subject<number> = new Subject();
navchange = this.navchangeSubject.asObservable();
selectedNavItem(item: number) {
console.log(`selected nav item ${item}`);
this.navchangeSubject.next(item);
}
}
In the
ObservingComponent
, create an instance of theNavigation
component and subscribe to thenavchange
observable:
import { Navigation } from './navigation.component';
export class ObservingComponent {
navigationComponent = new Navigation();
ngOnInit() {
this.navigationComponent.navchange.subscribe((item: number) => {
this.selectedNavItem(item);
});
}
selectedNavItem(item: number) {
console.log('item index changed!');
}
}
By using RxJS Observables, you have more flexibility and additional operators available for performing complex event handling. 🙌🔥
Choose Your Approach 👩💻👨💻
Now that you have two possible approaches for event delegation in Angular - using EventEmitter
or Observable
- it's time to choose the one that suits your project and requirements.
If you prefer a simpler, lightweight solution, go with EventEmitter
. On the other hand, if you need more flexibility and sophisticated event handling, give Observable
a try.
So, which approach are you going to use in your Angular project? Let us know in the comments below! 😄👇
Conclusion 📝✅
Delegation in Angular doesn't have to be complicated. By using either EventEmitter
or Observable
, you can easily emit events from one component and handle them in another component.
In this blog post, we covered both approaches, provided step-by-step instructions, and explained the benefits of each approach. Now it's time for you to implement delegation in your Angular project with confidence! 💪🚀
If you have any questions or additional tips, don't hesitate to leave a comment below. Happy delegating! 🎉🙌
📣 Did you find this blog post helpful? Share it with your fellow developers and spread the knowledge! 🤝🔗