Convert Promise to Observable
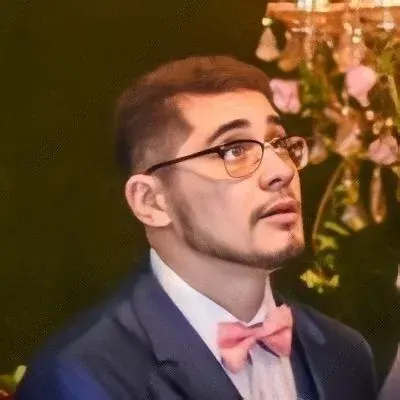
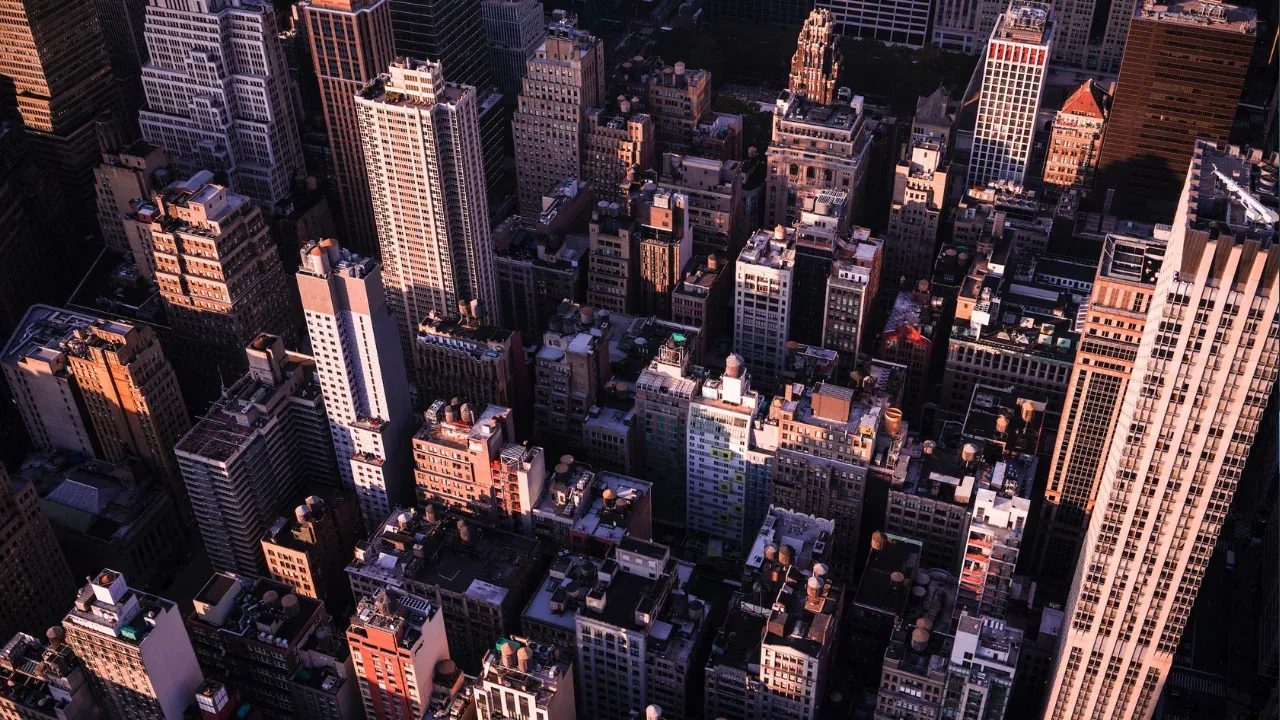
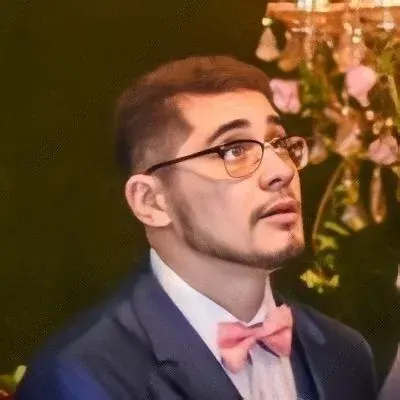
Converting a Promise to an Observable in Angular 🔄💡
Are you looking to convert a Promise to an Observable in Angular? You're in the right place! Observables are a powerful tool in Angular that help with development and increase readability. In this blog post, we'll explore how to convert a Promise to an Observable and provide easy solutions to common issues. Let's dive in! 🌊
Understanding the Challenge 🔍
Before we jump into the solution, let's understand the challenge at hand. The code snippet you provided is using the Firebase Authentication API to create a user with an email and password. The API returns a Promise, which you want to convert to an Observable pattern.
firebase.auth().createUserWithEmailAndPassword(email, password)
.then(function(firebaseUser) {
// do something to update your UI component
// pass user object to UI component
})
.catch(function(error) {
// Handle Errors here.
var errorCode = error.code;
var errorMessage = error.message;
// ...
});
Your goal is to convert this Promise-based code to use Observables, allowing you to leverage the benefits of observables such as data, error, and completion handlers. Let's see how we can achieve this! 🚀
Converting Promises to Observables 🔄
To convert a Promise to an Observable in Angular, we can utilize the from
function from the rxjs
library. This function allows us to create an Observable from a variety of sources, including Promises. Here's an easy solution for your specific case:
import { from } from 'rxjs';
// ...
const createUserWithEmailAndPassword$ = from(firebase.auth().createUserWithEmailAndPassword(email, password));
createUserWithEmailAndPassword$.subscribe(
(firebaseUser) => {
// do something to update your UI component
// pass user object to UI component
},
(error) => {
// Handle Errors here.
const errorCode = error.code;
const errorMessage = error.message;
// ...
}
);
In the code snippet above, we use the from
function to convert the Promise returned by createUserWithEmailAndPassword
into an Observable called createUserWithEmailAndPassword$
. We then subscribe to this Observable and provide two callback functions - one for handling the data (when the Promise resolves) and another for handling errors.
This solution enables you to take advantage of the Observable pattern in Angular, providing a consistent way to handle data, errors, and completion in your services. 🙌
Embracing the Power of Observables 🌟
By converting Promises to Observables, you benefit from the power and flexibility of the Observable pattern. Observables offer a wide range of operators and allow you to transform, combine, and manipulate data streams easily. You can also handle multiple events over time, making them suitable for real-time applications and UI updates.
🔥 Call to Action: Engage with our Community! 🔥
Have you converted Promises to Observables in your projects? Share your experiences, tips, and code snippets in the comments below! Let's learn from each other and make the most out of Angular's amazing development capabilities. 🤝😄
Remember, Observables are your secret weapon for managing complex asynchronous operations, so don't hesitate to explore them further and discover their true potential! Let's level up our Angular game together! 💪💻
Happy coding! 🎉🚀