Call child component method from parent class - Angular
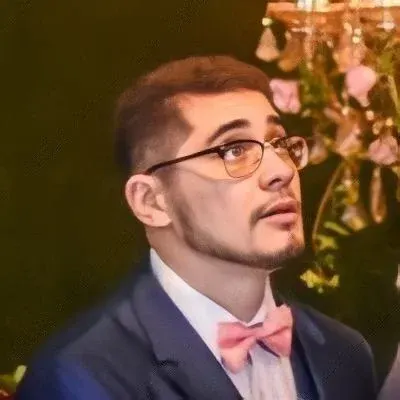
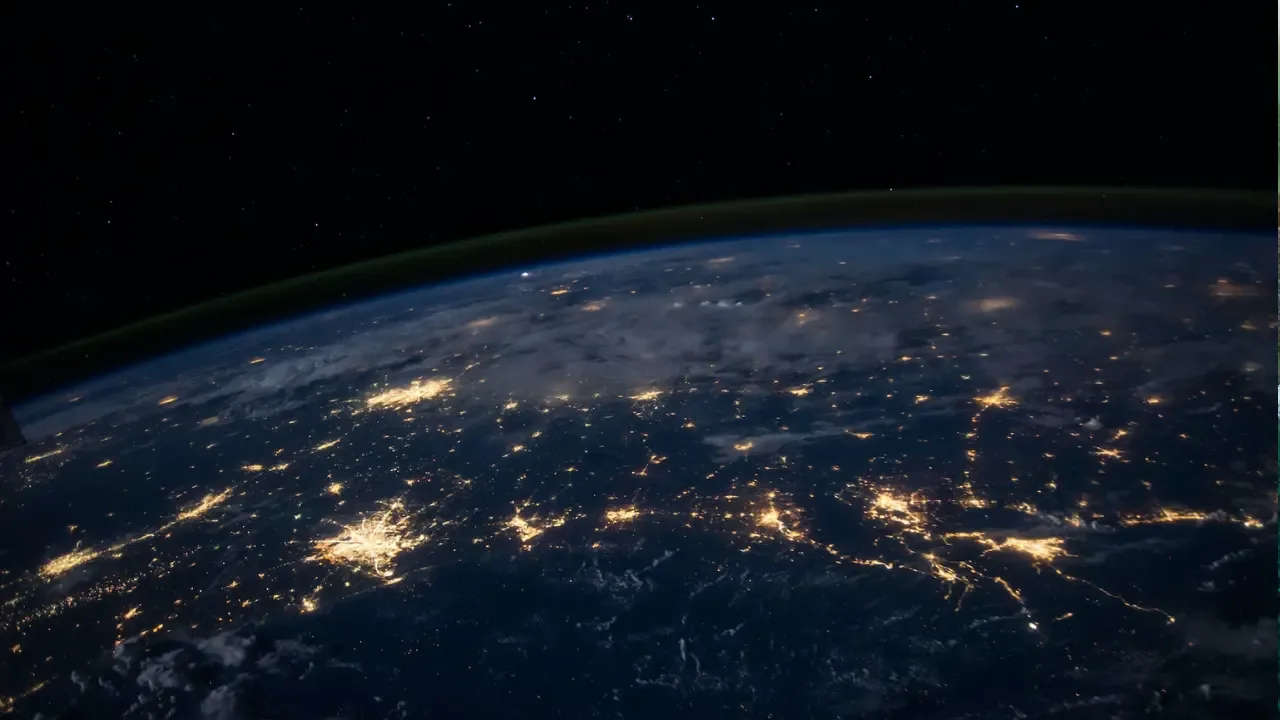
Call child component method from parent class - Angular
Are you struggling with calling a child component method from the parent class in Angular? Don't worry, you're not alone! This situation can be a bit tricky, but once you understand the concept, it becomes much easier. 😊
Let's dive into the code provided to understand the problem and find a solution.
The Problem
In the code snippet you provided, you have a parent component, AppComponent
, and a child component, NotifyComponent
. The issue is that when you invoke the callMethod()
in the parent component, it only fires the console.log()
line and doesn't set the test
property.
Understanding the Issue
The problem here is that you're creating a new instance of the NotifyComponent
in the parent component using this.notify = new NotifyComponent();
. This means that the child component instance in the parent component is not the same as the one being rendered in the template.
The Solution
To fix this issue and properly call the child component method from the parent class, you need to establish a communication channel between the parent and child components. There are several ways to achieve this, and I'll show you one of the simplest and most commonly used methods: using ViewChild
.
Import the
ViewChild
decorator from@angular/core
in the parent component.
import { Component, ViewChild } from '@angular/core';
import { NotifyComponent } from './notify.component';
Use
ViewChild
to bind the child component to a property in the parent component.
@ViewChild(NotifyComponent)
private notify: NotifyComponent;
Update the
submit()
method in the parent component to call the child component method using thenotify
property.
submit(): void {
// execute child component method
this.notify.callMethod();
}
With these changes, you'll be able to properly invoke the callMethod()
function and set the test
property in the child component.
Final Code
Here's the modified parent component code:
import { Component, ViewChild } from '@angular/core';
import { NotifyComponent } from './notify.component';
@Component({
selector: 'my-app',
template:
`
<button (click)="submit()">Call Child Component Method</button>
`
})
export class AppComponent {
@ViewChild(NotifyComponent)
private notify: NotifyComponent;
constructor() { }
submit(): void {
// execute child component method
this.notify.callMethod();
}
}
And the child component code remains the same:
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'notify',
template: '<h3>Notify {{test}}</h3>'
})
export class NotifyComponent implements OnInit {
test: string;
constructor() { }
ngOnInit() { }
callMethod(): void {
console.log('successfully executed.');
this.test = 'Me';
}
}
The Call-to-Action
Congratulations! You've learned how to properly call a child component method from the parent class in Angular. Now it's time to put your newfound knowledge into action. Go ahead and try implementing this solution in your own Angular app. If you have any questions or run into any issues, feel free to leave a comment below. Happy coding! 👩💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
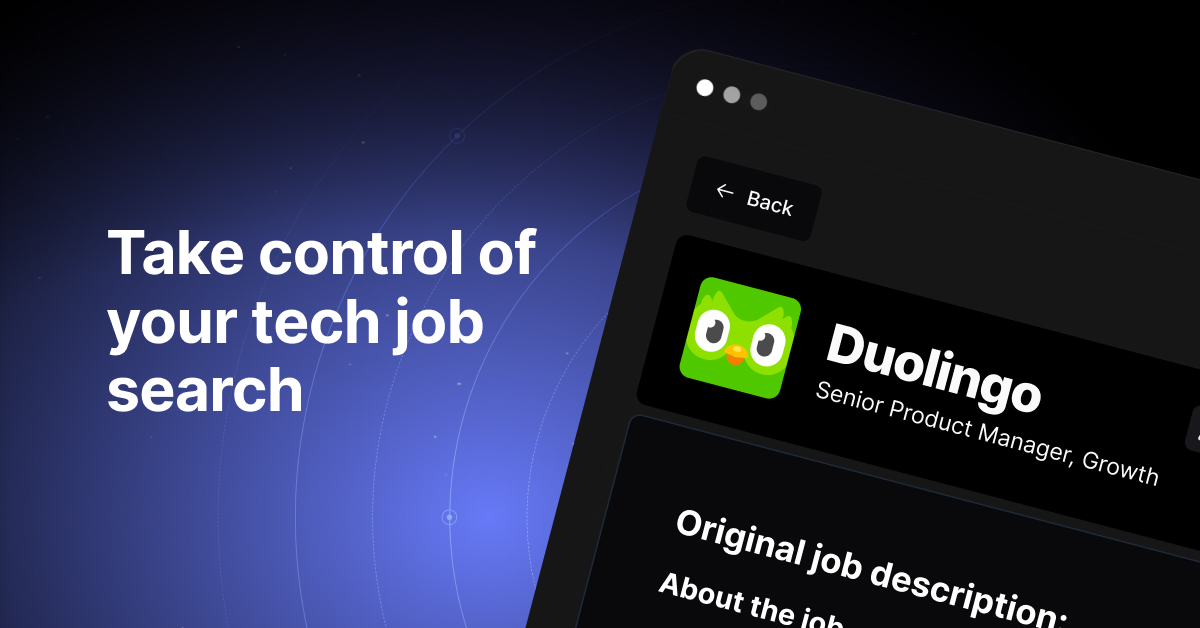