Angular - Use pipes in services and components
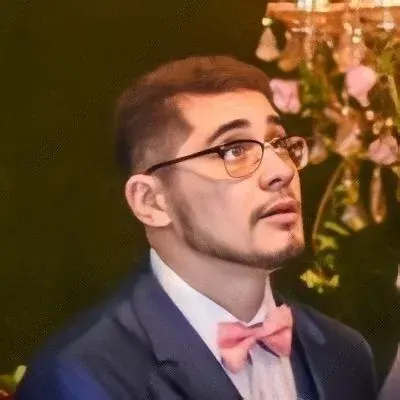
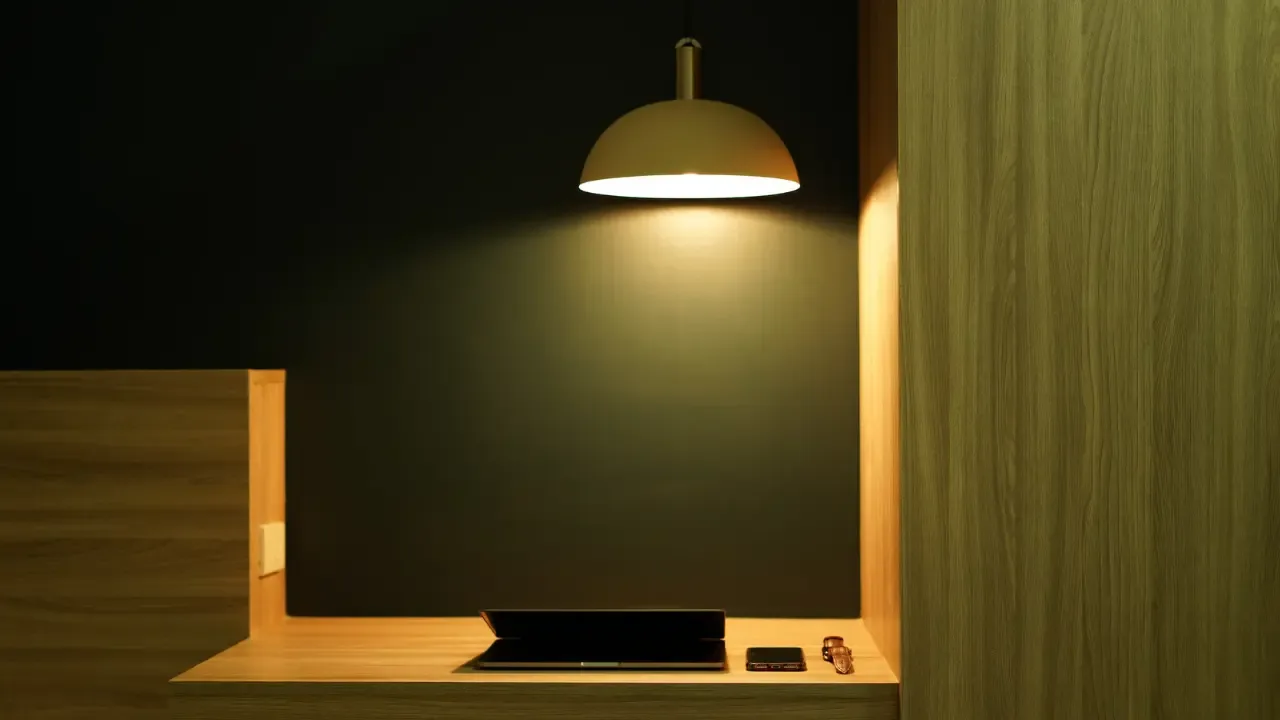
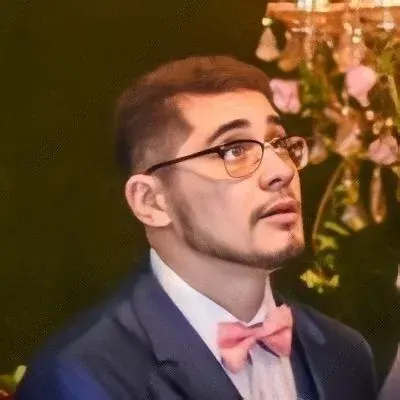
💡 Angular - Using Pipes in Services and Components
Are you an Angular enthusiast looking to use pipes, also known as filters, in your services and components? Well, buckle up, because we're about to dive into the world of Angular pipes and explore some handy tricks to make your code more efficient and readable.
🤔 The Question
The question at hand: Is it possible to use pipes in services and components, just like we did in AngularJS? Let's find out!
💡 The Solution
The good news is that Angular has an elegant way of achieving similar functionality using dependency injection. By injecting the PipeTransform
interface, we can leverage pipes in our services and components effortlessly.
To begin, let's say we have a pipe called date
that formats a given date object into a specific format:
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'date'
})
export class DatePipe implements PipeTransform {
transform(value: Date, format: string): string {
// Your date formatting logic here
return formattedDate;
}
}
Now that we have our pipe, let's see how we can use it in our services and components.
🛠️ Using Pipes in Services
To use a pipe in a service, we need to inject the PipeTransform
and the Injector
from @angular/core
. Here's an example of how we can achieve this:
import { Injectable, Injector } from '@angular/core';
import { DatePipe } from './date.pipe';
@Injectable({
providedIn: 'root'
})
export class MyService {
private datePipe: DatePipe;
constructor(private injector: Injector) {
// Inject the DatePipe using the Injector
this.datePipe = this.injector.get(DatePipe);
}
formatDate(date: Date, format: string): string {
return this.datePipe.transform(date, format);
}
}
By injecting the DatePipe
using the Injector
, we have access to all the functionalities provided by the pipe within our service. We can then use the formatDate
method to transform any given date using the pipe.
🚀 Using Pipes in Components
Using pipes in components is even simpler. All we need to do is declare the pipe within the component's providers
array:
import { Component } from '@angular/core';
import { DatePipe } from './date.pipe';
@Component({
selector: 'app-my-component',
templateUrl: './my-component.component.html',
providers: [DatePipe]
})
export class MyComponent {
constructor(private datePipe: DatePipe) {}
formatCurrentDate(format: string): string {
const currentDate = new Date();
return this.datePipe.transform(currentDate, format);
}
}
By declaring the DatePipe
within the component's providers
array, Angular will make it available for use throughout the component.
👏 Final Thoughts
Using pipes in services and components can be a powerful way to maintain code consistency and improve readability. By injecting the PipeTransform
in services and declaring the pipe in the providers
array of components, we can easily leverage the functionalities of pipes wherever needed.
So, whether you're formatting dates, numbers, or customizing your pipe, Angular has got you covered!
Now it's your turn! Have you used pipes in services or components before? Share your experiences and any cool tips you've discovered in the comments below. Let's learn together! 👇