Angular/RxJS When should I unsubscribe from `Subscription`
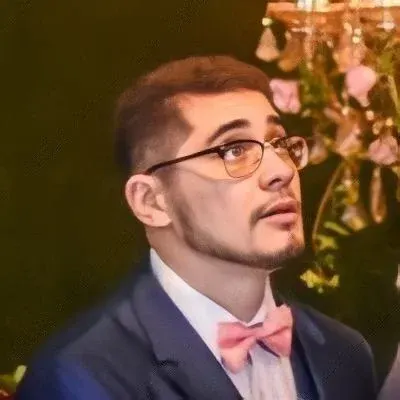
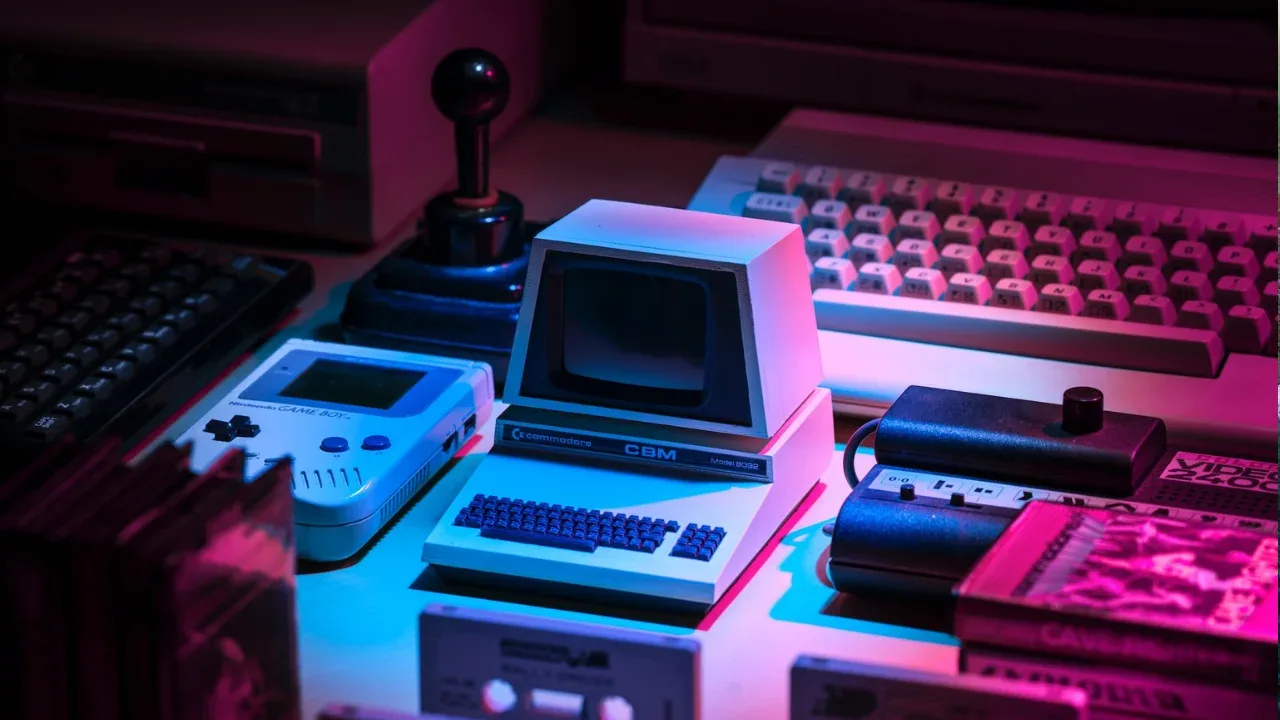
📝 When to Unsubscribe from Subscription
in Angular/RxJS
Have you ever wondered when you should unsubscribe from a Subscription
in Angular and RxJS? 🤔 It's a common question among developers who are concerned about avoiding memory leaks and keeping their code clean and efficient. In this blog post, we will explore the different scenarios where you should or shouldn't unsubscribe and provide easy solutions to help you effectively manage your subscriptions. Let's dive in! 💪
The Dilemma
The first thing you might be wondering is, "When should I store the Subscription
instances and invoke unsubscribe()
during the ngOnDestroy
life cycle, and when can I simply ignore them?" Good news! You don't always have to store and manually unsubscribe from every Subscription
you create. 🙌
Ignoring Subscriptions
The Angular HTTP Client Guide suggests ignoring subscriptions in certain scenarios. Here's an example from the guide:
getHeroes() {
this.heroService.getHeroes()
.subscribe(
heroes => this.heroes = heroes,
error => this.errorMessage = <any>error);
}
In this case, it's safe to ignore the subscription. Why? Because Angular's HttpClient
automatically completes the Observable returned by getHeroes()
after it emits the data or an error. So, you don't have to worry about unsubscribing manually. 😎
Cleaning Up Subscriptions
However, there are situations where you do need to clean up your subscriptions to avoid memory leaks. The Route & Navigation Guide provides a perfect example:
"Eventually, we'll navigate somewhere else. The router will remove this component from the DOM and destroy it. We need to clean up after ourselves before that happens. Specifically, we must unsubscribe before Angular destroys the component. Failure to do so could create a memory leak."
Here's an example from the guide that demonstrates the proper way to unsubscribe in the ngOnDestroy
method:
private sub: any;
ngOnInit() {
this.sub = this.route.params.subscribe(params => {
let id = +params['id']; // (+) converts string 'id' to a number
this.service.getHero(id).then(hero => this.hero = hero);
});
}
ngOnDestroy() {
this.sub.unsubscribe();
}
In this case, if you don't unsubscribe from the params
subscription, it will keep listening even after you navigate away from the component, potentially causing memory leaks. As a general rule, any subscriptions related to an ongoing task or asynchronous operation should be unsubscribed in the ngOnDestroy
method. 👍
Take Control of Your Subscriptions
To summarize, you should consider unsubscribing from subscriptions in the following scenarios:
When the Observable you subscribe to is not automatically completed (e.g., HTTP requests).
When you navigate away from a component and want to avoid memory leaks.
For all other cases, such as subscriptions that complete on their own, there's no need to worry about manual unsubscription.
Now that you understand when to unsubscribe from Subscription
, you can take control of your code and avoid memory leaks. It's important to strike a balance between cleanliness and efficiency in your Angular applications! 🚀
Your Turn! 📣
Have you encountered any challenges with managing subscriptions in Angular? How do you handle them in your projects? Share your experiences and insights in the comments below. Let's learn from each other and make our Angular code even better! 👇
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
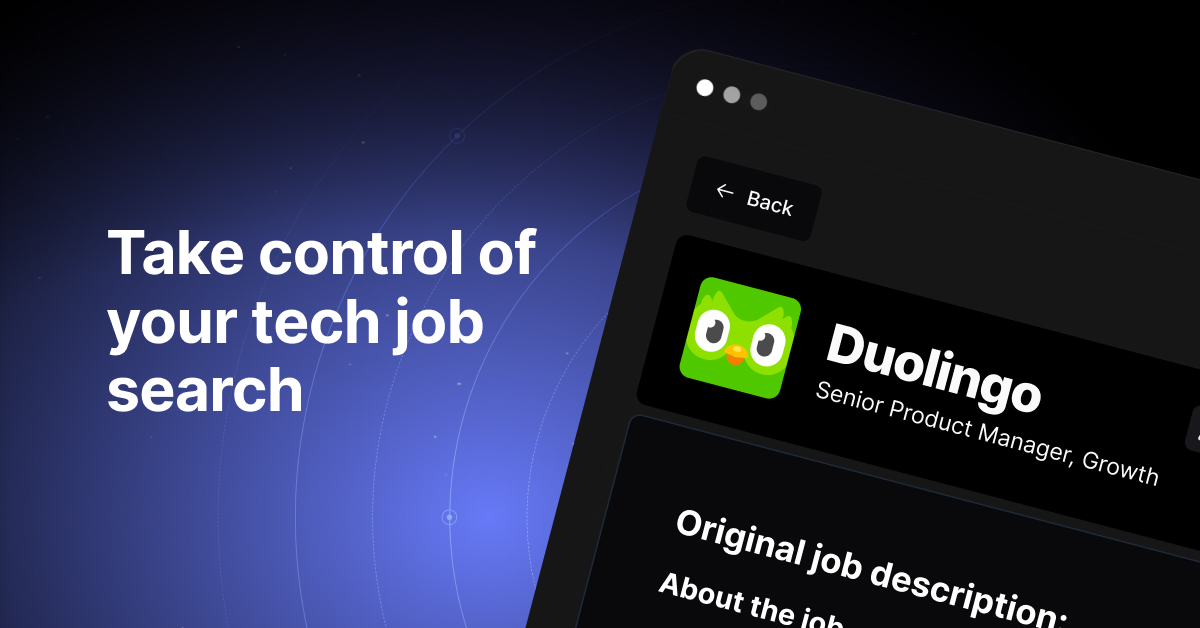