Angular + Material - How to refresh a data source (mat-table)
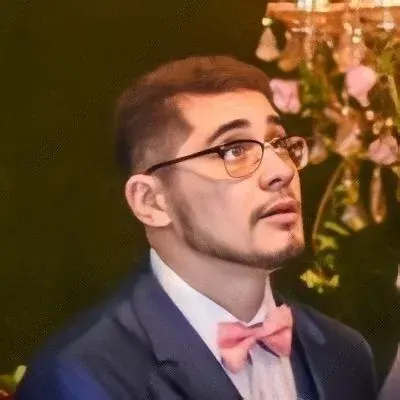
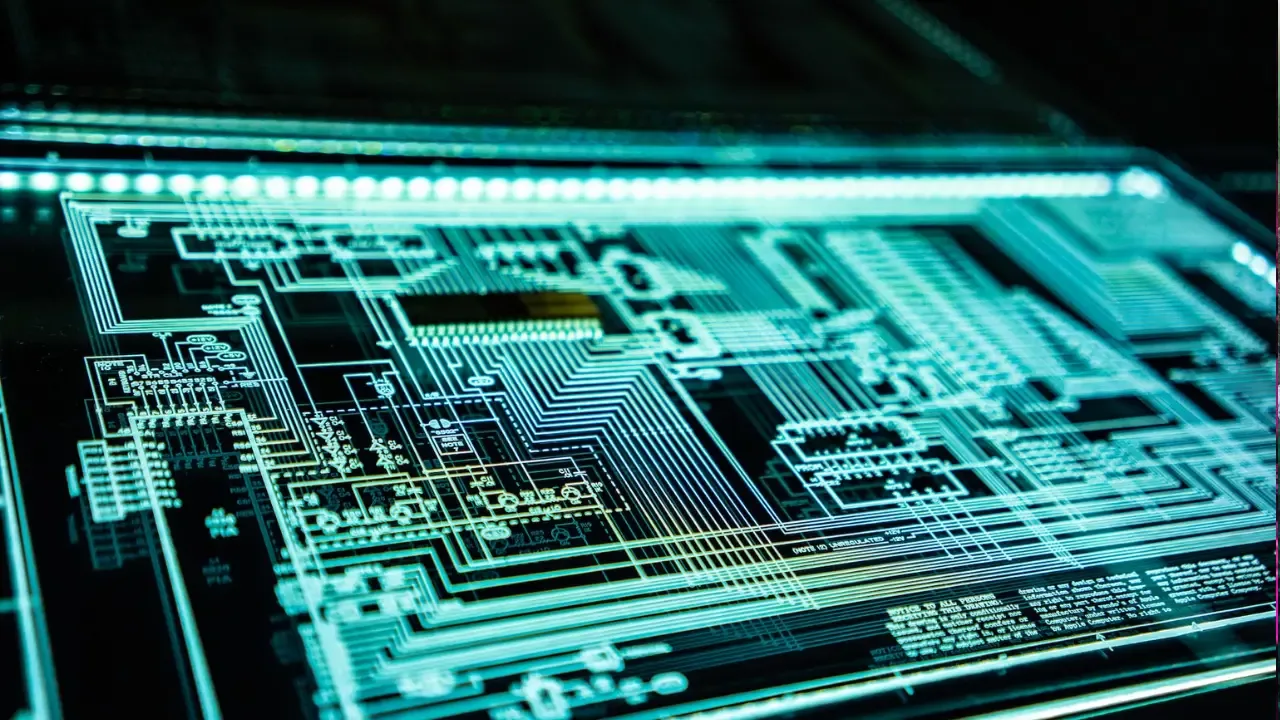
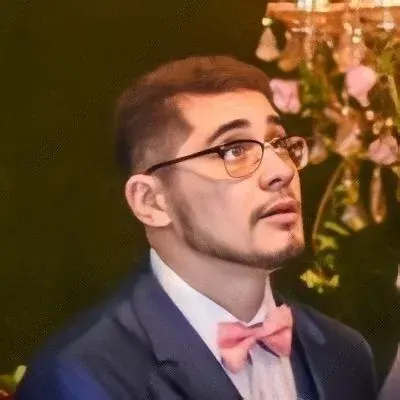
📝 Angular + Material - How to refresh a data source (mat-table) 🔄
Are you having trouble refreshing the data source in your Angular application when using the Material mat-table component? You're not alone! Many developers face this issue when implementing dynamic data updates. But worry not, because I'm here to guide you through the process. Let's dive in!
🔍 The Problem: Refreshing the Data Source
In the given context, the objective is to refresh the data source (mat-table) after adding a new language using a dialog panel. However, despite calling the refresh method and reinitializing the data source, no changes are applied.
💡 The Solution: The Correct Method
To resolve this issue, there are a few steps we need to follow. Let's take a closer look at the code and make necessary adjustments.
1️⃣ In LanguageDataSource
class:
Import the
BehaviorSubject
from RxJS usingimport { BehaviorSubject } from 'rxjs';
.Create a
private
BehaviorSubject
variable, let's name itdataSubject
, and initialize it with an empty array. This will act as our data source.Replace the
connect()
method with the following code:connect(): Observable<any[]> { return this.dataSubject.asObservable(); }
In your
refresh()
method, after receiving the updated data from the backend, update thedataSubject
with the new data:refresh() { this.authService.getAuthenticatedUser().subscribe((res) => { this.user = res; this.dataSubject.next(this.user.profile.languages.teach); }); }
2️⃣ In LanguageComponent
class:
Remove the
teachDS
property and theLanguageDataSource
import. We will make use of theMatTableDataSource
provided by Angular Material instead.Import the
MatTableDataSource
from Angular Material usingimport { MatTableDataSource } from '@angular/material';
.Replace the
refresh()
method code with the following:refresh() { this.authService.getAuthenticatedUser().subscribe((res) => { this.user = res; this.teachDS = new MatTableDataSource(this.user.profile.languages.teach); }); }
In the template, bind the
mat-table
to theteachDS
property:<table mat-table [dataSource]="teachDS"> <!-- column definitions --> </table>
3️⃣ Lastly, don't forget to call the refresh()
method after the add language dialog is closed:
add() {
this.dialog.open(LanguageAddComponent, {
data: { user: this.user },
}).afterClosed().subscribe(result => {
this.refresh();
});
}
🎉 You Did It!
Congrats! You've successfully implemented the necessary changes to refresh the data source (mat-table) when adding a new language. Now, when the user adds a new language using the dialog panel and returns back, the changes will be reflected in the table.
👉 Engage with us
Have you encountered other challenges while working with Angular + Material? Let us know in the comments below! We're here to help you solve your coding mysteries.
🎯 Call-to-Action: Share and Engage
If you found this blog post helpful, share it with your fellow Angular enthusiasts! Let's spread the word and help each other create amazing projects 👍 Don't forget to comment and share your thoughts, experiences, and any additional questions you may have. We love engaging with our readers!
Thanks for reading! Happy coding! 💻✨