Angular EXCEPTION: No provider for Http
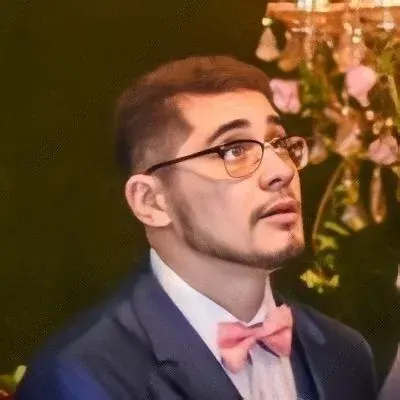
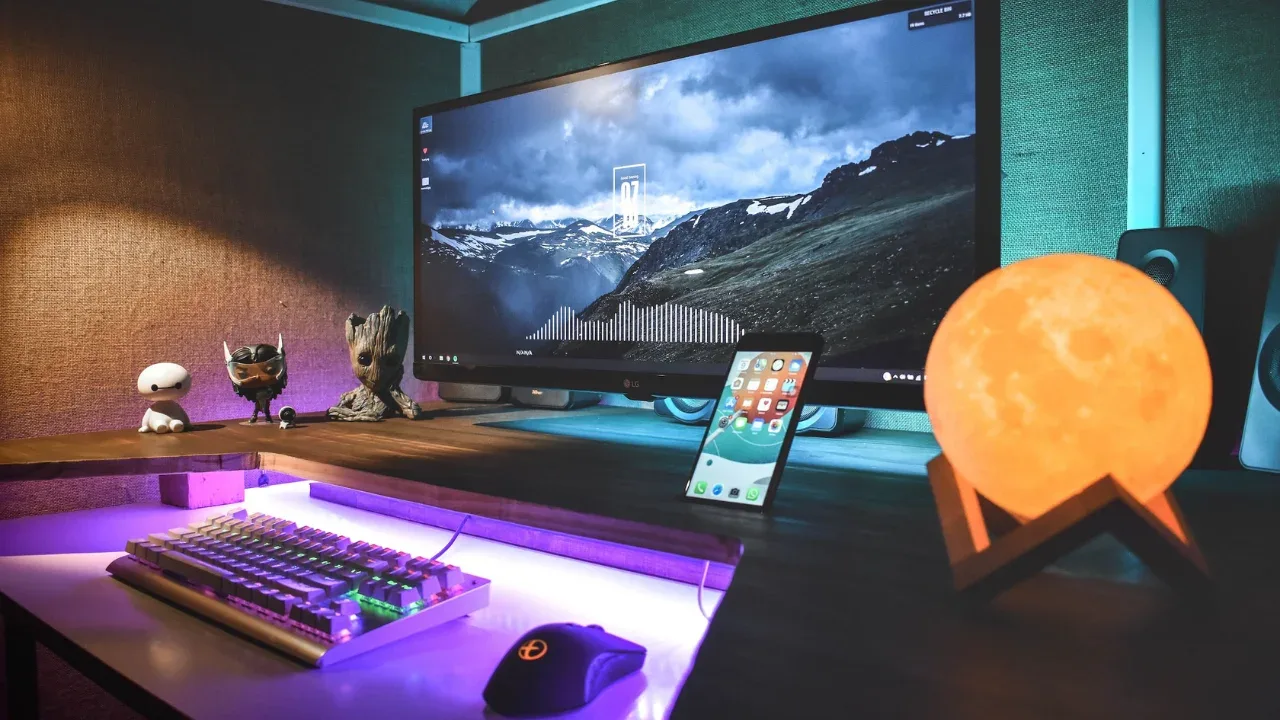
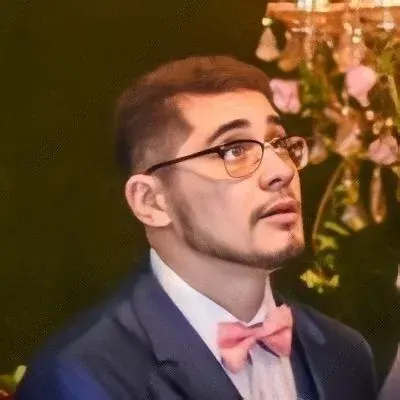
Angular EXCEPTION: No provider for Http
If you are encountering the error message "EXCEPTION: No provider for Http!" in your Angular app, don't worry! You're not alone. This error typically occurs when you are trying to use the Http service in your Angular app, but haven't properly configured the necessary dependencies.
Understanding the Problem
In order to use the Http service in Angular, you need to make sure you have the proper imports and providers set up. Looking at the code snippet provided, it seems that the Http service is being used in the constructor
of the GreetingsAcApp2
component.
import {Http, Headers} from 'angular2/http';
import {Injectable} from 'angular2/core'
@Component({
selector: 'greetings-ac-app2',
providers: [],
templateUrl: 'app/greetings-ac2.html',
directives: [NgFor, NgModel, NgIf, FORM_DIRECTIVES],
pipes: []
})
export class GreetingsAcApp2 {
private str:any;
constructor(http: Http) {
this.str = {str:'test'};
http.post('http://localhost:18937/account/registeruiduser/',
JSON.stringify(this.str),
{
headers: new Headers({
'Content-Type': 'application/json'
})
});
}
}
Easy Solutions
To resolve the "EXCEPTION: No provider for Http!" error, you need to make sure you have the necessary imports and providers added correctly.
Step 1: Import the Http module
Import the Http module from the correct location in your Angular project. Starting from Angular 2, the Http module is located at '@angular/http'
. Update your import statement to reflect this change.
import {Http, Headers} from '@angular/http';
Step 2: Add Http to the providers
Add the Http provider to the providers
array in the @Component
decorator's configuration, so that it can be injected into the component's constructor.
@Component({
selector: 'greetings-ac-app2',
providers: [Http], // Add Http to the providers array
templateUrl: 'app/greetings-ac2.html',
directives: [NgFor, NgModel, NgIf, FORM_DIRECTIVES],
pipes: []
})
Step 3: Use Http as a dependency in the constructor
In the constructor of your component, add private http: Http
as a parameter, and assign it to a class property.
constructor(private http: Http) {
// Rest of your code
}
Call-to-Action
Now that you know how to resolve the "EXCEPTION: No provider for Http!" error in your Angular app, go ahead and give it a try. Make sure to double-check your imports, add the Http provider to the providers array, and update the constructor to include Http as a dependency.
If you found this guide helpful, let me know in the comments below. Also, if you have any questions or other issues you'd like me to cover, feel free to reach out. Happy coding! 🚀