Angular 2 - innerHTML styling
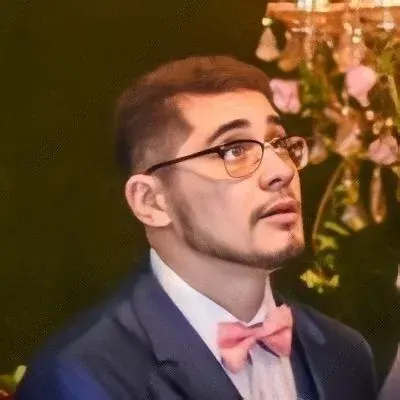
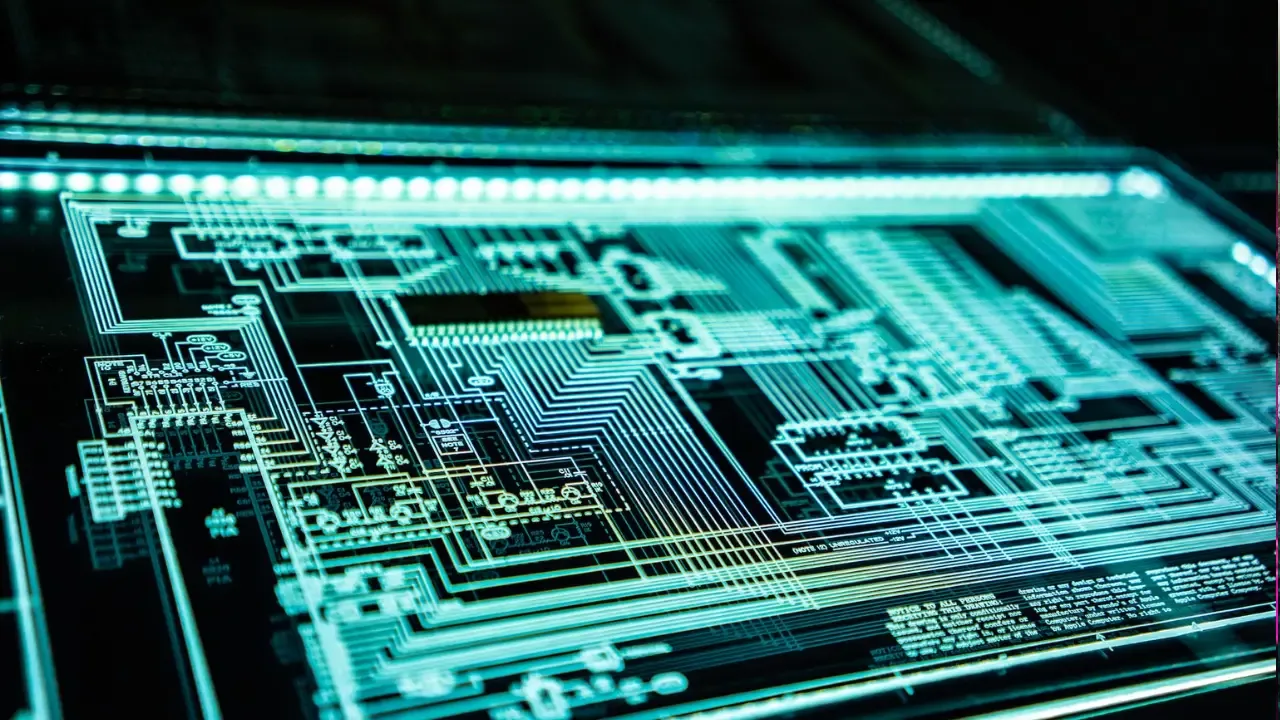
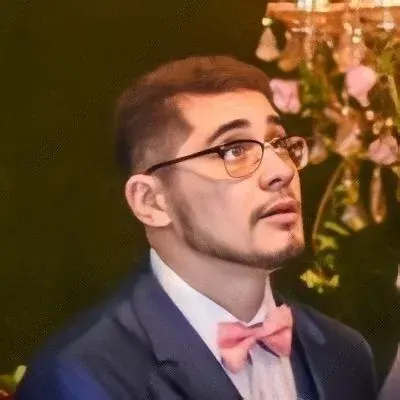
Angular 2 - Styling innerHTML: A Guide to Taming the HTML Beast 🎨
Are you struggling to style the content inserted using [innerHTML]
in your Angular 2 application? 🙄 Don't worry, you're not alone! Many developers face this issue, but luckily, there are easy solutions that can help you style the inserted HTML block with ease. Let's dive in! 💪
The Problem 🤔
The problem arises when you are getting chunks of HTML code from HTTP calls and inserting them into your page using [innerHTML]
. Although the HTML is being inserted successfully, the applied styles are not being reflected on the inserted content. This can be quite frustrating, as you want the inserted content to look consistent with the rest of your application.
The Solution 🔧
To style the inserted HTML block, you need to make use of Angular's dynamic styling capabilities. There are two primary approaches you can take to achieve this:
1. Using a Wrapper Element 👥
One way to apply styles to the inserted HTML block is by wrapping it in a wrapper element and dynamically adding a class or styles to that wrapper. Let's look at an example:
@Component({
selector: 'calendar',
template: '<div class="calendar-wrapper" [innerHTML]="calendar"></div>',
styles: [
`.calendar-wrapper {
/* Your styles go here */
color: red;
}`
]
})
In this example, we added a calendar-wrapper
class to the wrapper div element and applied styles specifically to that class in the component's styles array. This way, the inserted HTML block will inherit the styles applied to its wrapper element, giving you the desired styling result. 😎
2. Using Host Binding 🏠
Another approach is to use Angular's HostBinding
decorator to dynamically bind inline styles to the host element. Here's how you can achieve this:
import { Component, HostBinding } from '@angular/core';
@Component({
selector: 'calendar',
template: '<div [innerHTML]="calendar"></div>',
})
export class CalendarComponent {
@HostBinding('style.color')
calendarStyles = 'red';
}
In this example, we are using the @HostBinding
decorator along with style.propertyName
notation to bind the color
property of the host element to a variable called calendarStyles
. By assigning a value to calendarStyles
, we can dynamically apply styles to the inserted HTML block.
The Call to Action 💥
Now that you know how to style the inserted HTML block in Angular 2, why not give it a try? Implement these solutions in your code and see the magic happen. Also, don't hesitate to share your experience and any other cool tips you've discovered along the way in the comments section below! Let's unlock the beauty of HTML styling together! 💅🌈
References 🔍
That's it, folks! Now go forth and conquer the HTML beast with your newfound styling powers! 🦁✨