Angular 2 - How do I navigate to another route using this.router.parent.navigate("/about")?
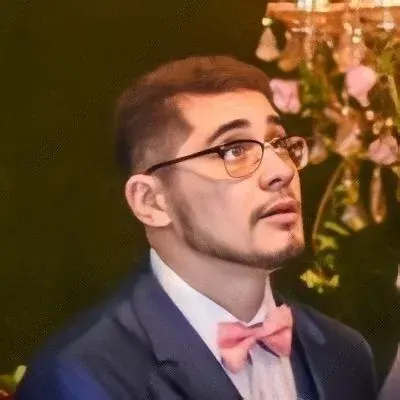
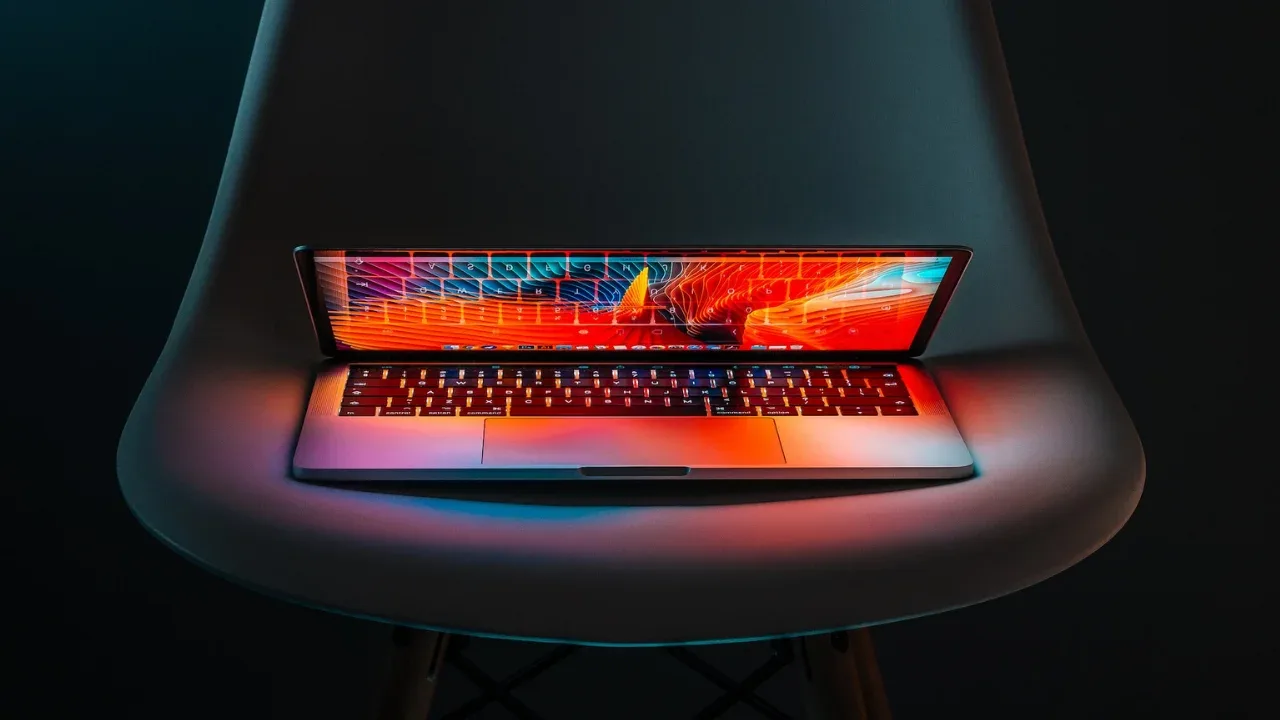
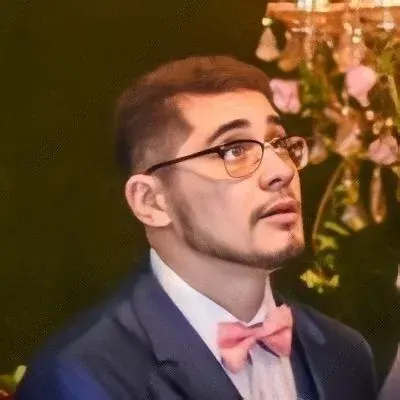
How to Navigate to Another Route in Angular 2 using this.router.parent.navigate('/about')
Are you having trouble navigating to another route in Angular 2 using this.router.parent.navigate('/about')
? Don't worry, you're not alone! Many developers encounter this issue and find it difficult to resolve. But fear not, we're here to help you navigate through this problem and find a solution that works for you.
The Problem
Based on the context you provided, it seems that you have written code to navigate to the '/about' route after a user has logged in. However, the navigation doesn't seem to be working as expected. You tried using location.go("/about")
, but that also didn't work. So what could be the issue here?
The Solution
To understand the solution, we need to dig a little deeper into the code snippet you provided. Let's take a closer look at the relevant parts:
import { Component } from 'angular2/angular2';
import { CORE_DIRECTIVES, FORM_DIRECTIVES } from 'angular2/angular2';
import { Router } from 'angular2/router';
import { AuthService } from '../../authService';
// Model
class User {
constructor(public email: string, public password: string) {}
}
@Component({
templateUrl: 'src/app/components/todo/todo.html',
directives: [CORE_DIRECTIVES, FORM_DIRECTIVES]
})
export class Todo {
model = new User('Mark@gmail.com', 'Password');
authService: AuthService;
router: Router;
constructor(_router: Router, _authService: AuthService) {
this.authService = _authService;
this.router = _router;
}
onLogin = () => {
this.authService.logUserIn(this.model).then((success) => {
// This is where it's broken - below:
this.router.parent.navigate('/about');
});
}
}
The issue here is that you are trying to navigate to another route using this.router.parent.navigate('/about')
. The router
property in your component is correctly injected by Angular's dependency injection system, but the parent
property might not be what you expect it to be.
In Angular 2, the Router
class has a navigateByUrl
method that is used to navigate to a specific URL. So, instead of using this.router.parent.navigate('/about')
, you should use this.router.navigateByUrl('/about')
to navigate to the '/about' route.
Here's how your code should look with the correct navigation method:
onLogin = () => {
this.authService.logUserIn(this.model).then((success) => {
this.router.navigateByUrl('/about');
});
}
With this simple change, you should be able to navigate to the '/about' route successfully.
Still having issues?
If you're still facing issues with navigation, it is worth checking if you have properly configured your routes and if the '/about' route is defined in your routing configuration.
Additionally, ensure that you have imported the correct classes and modules related to routing in your application.
Conclusion
Navigating to another route in Angular 2 can be a bit tricky, especially if you're using the this.router.parent.navigate('/about')
method. However, by using the this.router.navigateByUrl('/about')
method, you can easily resolve this issue and successfully navigate to your desired route.
We hope this guide has helped you understand the problem and provided a clear solution. If you still have any questions or facing any issues, feel free to leave a comment below. Happy coding! 😊🚀
Did you find this guide helpful? Share it with your fellow Angular 2 developers and spread the knowledge! 📢💻
Subscribe to our newsletter 📧👉 Newsletter Subscription
Follow us on Twitter 🐦👉 Twitter Handle
Join our community 💬👉 Community Name