Angular 2: 404 error occur when I refresh through the browser
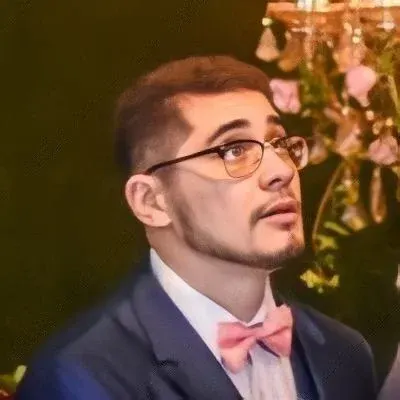
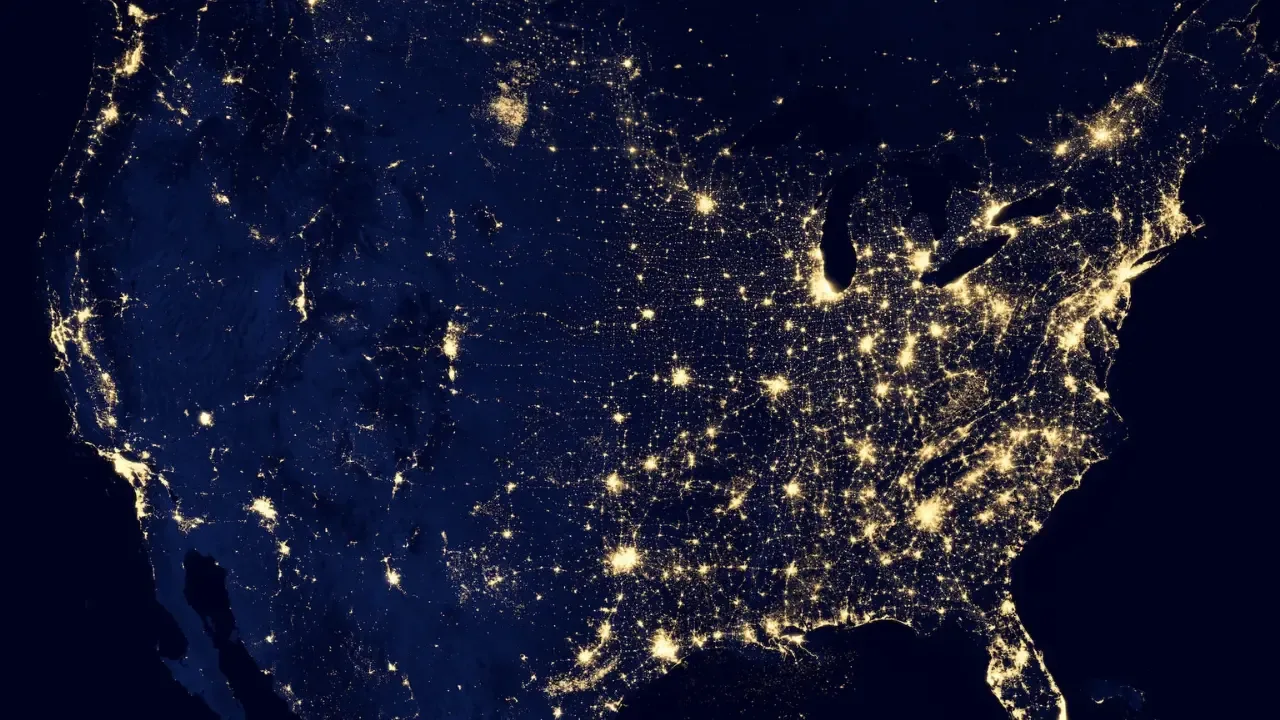
Angular 2: Solving the 404 Refresh Error on Browser
So, you're building a cool Angular 2 single-page application, and you encounter a pesky 404 error when you try to refresh the page in your browser. Don't worry, you're not alone. This is a common issue that many developers face when setting up their Angular 2 projects.
Understanding the Problem
Let's break it down. The problem arises when you try to refresh a page in your Angular 2 application that has routing enabled. By default, Angular 2 uses hash-based routing, which means that your URLs would look something like this: http://example.com/#/login
. However, you mentioned that you changed the base URL to http://example.com/myapp/
, and this is where the trouble starts.
When you refresh the page, the browser sends a request to the server for the URL you're currently on (http://example.com/myapp/login
). Since Angular routing is client-side, the server doesn't recognize this URL and responds with a 404 error because it can't find a matching route on the server.
Solving the Issue
Now that we understand the problem, let's dive into the solutions. There are a few different approaches you can take to fix the 404 refresh error in Angular 2. Let's explore two common solutions:
1. Using a server-side redirect
One way to solve this issue is by configuring your server to redirect all routes to the index.html
file. This way, the server will always serve the same HTML file regardless of the route requested, and your Angular routing will take care of displaying the correct content based on the URL.
The specific steps to configure the server-side redirect depend on the server you're using. For example, if you're using Apache, you can achieve this by adding a .htaccess
file in the root directory with the following content:
<IfModule mod_rewrite.c>
RewriteEngine On
RewriteBase /myapp/
RewriteRule ^index\.html$ - [L]
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{REQUEST_FILENAME} !-d
RewriteRule . /myapp/index.html [L]
</IfModule>
This configuration tells Apache to always serve the index.html
file for any request that is not an existing file or directory. Make sure to adjust the RewriteBase
directive to match your desired base URL.
2. Using HashLocationStrategy
Another approach is to revert to using hash-based routing instead of the HTML5 push-state routing. This means that your URLs will contain a #
symbol, but the advantage is that it avoids the 404 refresh error.
To enable hash-based routing, you need to update your Angular 2 app's routing configuration. In your app.module.ts
file, import the HashLocationStrategy
and LocationStrategy
from the @angular/common
module, and specify HashLocationStrategy
as the LocationStrategy
provider:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { HashLocationStrategy, LocationStrategy } from '@angular/common';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, AppRoutingModule],
providers: [{ provide: LocationStrategy, useClass: HashLocationStrategy }],
bootstrap: [AppComponent]
})
export class AppModule {}
By using HashLocationStrategy
, Angular will append a #
symbol to your URLs, allowing the browser to handle them differently and avoid triggering the 404 error on refresh.
The Final Step
Now that you have a couple of solutions to choose from, it's time to put them into action and get rid of that pesky 404 error on refresh. Remember to test your application thoroughly after implementing any changes to ensure everything is working as expected.
And don't forget to share your experience! If you found this guide helpful or have any additional tips, let us know in the comments below. Happy coding! 💻🚀
📢 Your Turn: Share Your Experience!
Have you encountered the 404 refresh error in Angular 2 before? What solution did you use to fix it? Share your thoughts, experiences, and any additional tips you have in the comments below. Let's help each other build amazing apps!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
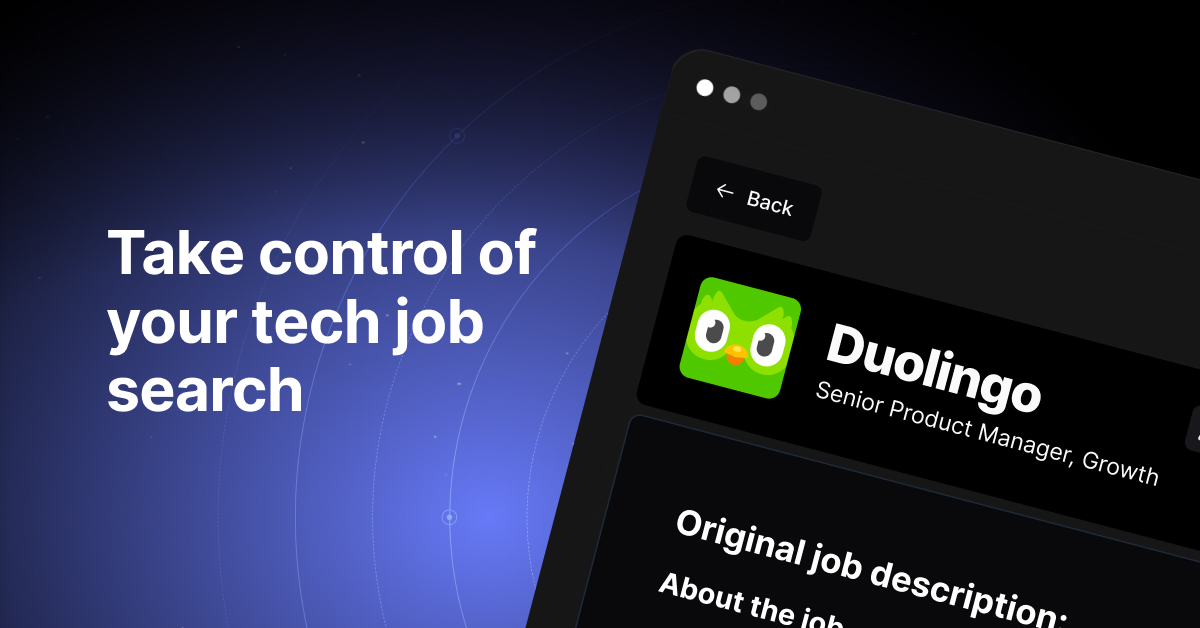