How to handle button clicks using the XML onClick within Fragments
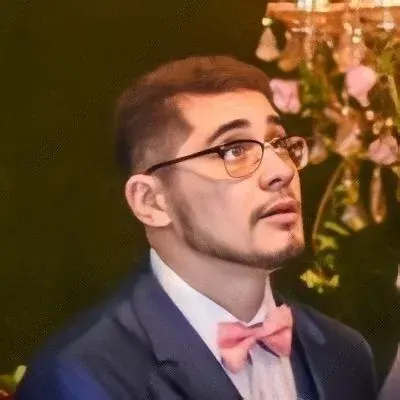
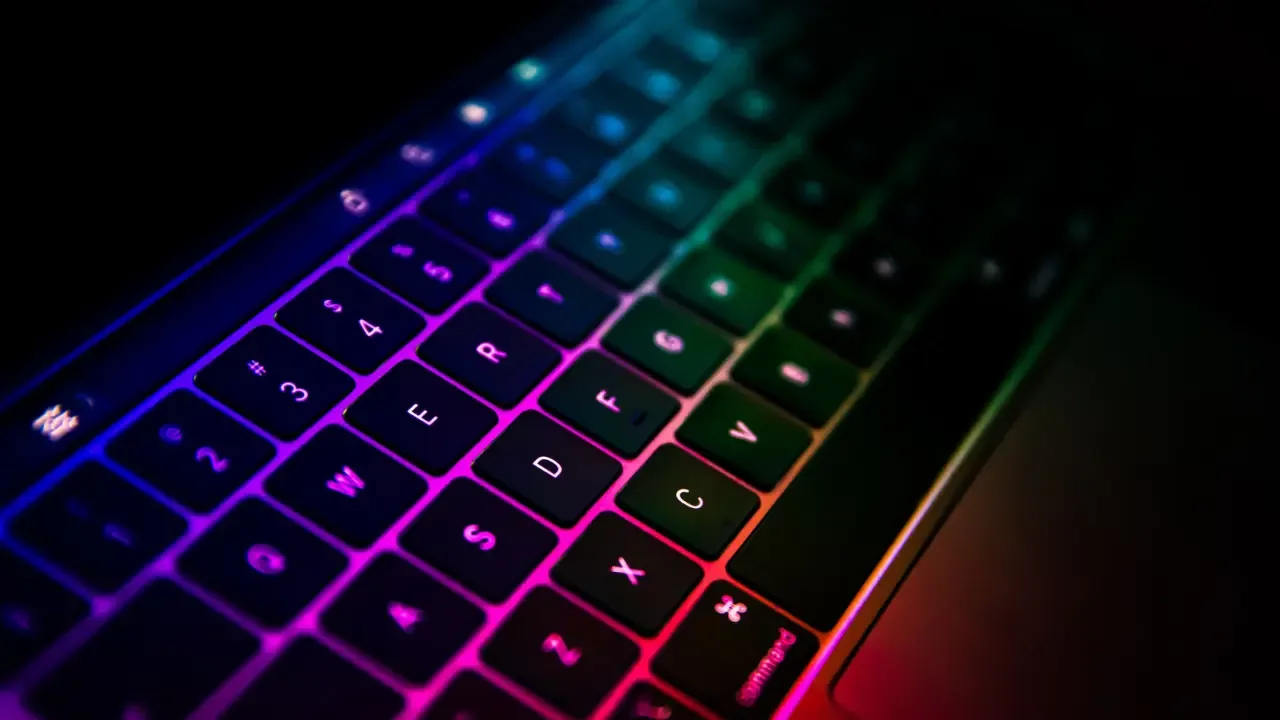
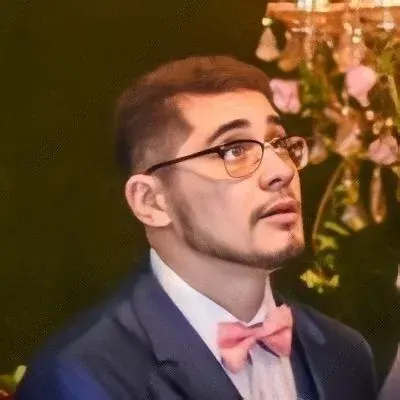
Handling Button Clicks in Fragments Using XML onClick
π‘Are you facing issues with handling button clicks in your Fragments using XML onClick? π€ Don't worry, we've got you covered! In this blog post, we'll address common issues and provide easy solutions to efficiently handle button clicks in Fragments. Let's dive right in! πββοΈ
The Traditional Approach
Before Honeycomb (Android 3), button clicks were handled within Activities using the onClick
attribute in XML layouts. It looked something like this:
android:onClick="myClickMethod"
Inside the corresponding Activity, you could use view.getId()
and a switch statement to perform the button logic. ππ
Fragments and Resuability
With the introduction of Fragments, the need for reusability became paramount. When breaking Activities into Fragments, the code for button logic should reside within the Fragments themselves, rather than the hosting Activity. Thankfully, we have a simple solution! π
Solution: Registering Fragments to Receive Button Clicks
If you want the Fragments to directly receive button clicks, you can register them by implementing the View.OnClickListener
interface in your Fragment class. Here's how you can do it:
public class MyFragment extends Fragment implements View.OnClickListener {
// ...
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_layout, container, false);
Button button = view.findViewById(R.id.button_id);
button.setOnClickListener(this);
return view;
}
@Override
public void onClick(View v) {
// Handle button click here
switch (v.getId()) {
case R.id.button_id:
// Perform action on button click
break;
// Handle other button clicks if needed
}
}
// ...
}
By implementing the View.OnClickListener
interface, the Fragment can directly handle button clicks through the onClick
method. No need to involve the hosting Activity anymore! ππ
Solution: Passing Click Events from Activity to Fragments
If you prefer to handle button clicks in the hosting Activity but still want Fragments to execute specific actions, you can pass the click events from the Activity to the appropriate Fragments. Here's how you can achieve this:
In your Fragment class, create a method to handle the specific action:
public void performButtonAction() {
// Perform the button action here
}
In the hosting Activity, find the relevant Fragment using FragmentManager and call the method created in step 1:
public class MyActivity extends AppCompatActivity {
// ...
public void handleClickEvent(View view) {
FragmentManager fragmentManager = getSupportFragmentManager();
MyFragment myFragment = (MyFragment) fragmentManager.findFragmentById(R.id.fragment_container);
myFragment.performButtonAction();
}
// ...
}
In this approach, the button clicks are still handled by the Activity, but the relevant action is executed within the Fragment. π
Conclusion
Handling button clicks in Fragments using XML onClick may seem tricky at first, but with the solutions provided above, you can effectively manage button logic within your Fragments. Whether you choose to directly register Fragments or pass click events from the Activity, these approaches will ensure smooth functionality and reusability.
Got more questions or facing other challenges? Let us know in the comments below! We'd love to help you out. ππ
Now go ahead and implement these strategies in your Fragment-based Android projects! πͺπ± Don't forget to share your experience with us and stay tuned for more tech tips and insights. Happy coding! πβ¨