How to declare global variables in Android?
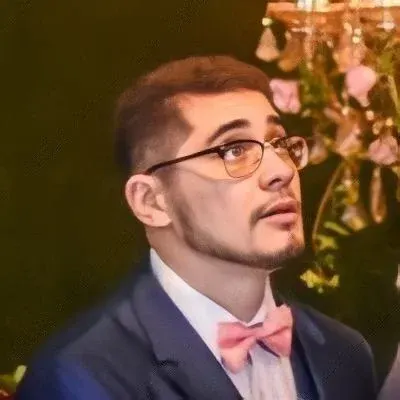
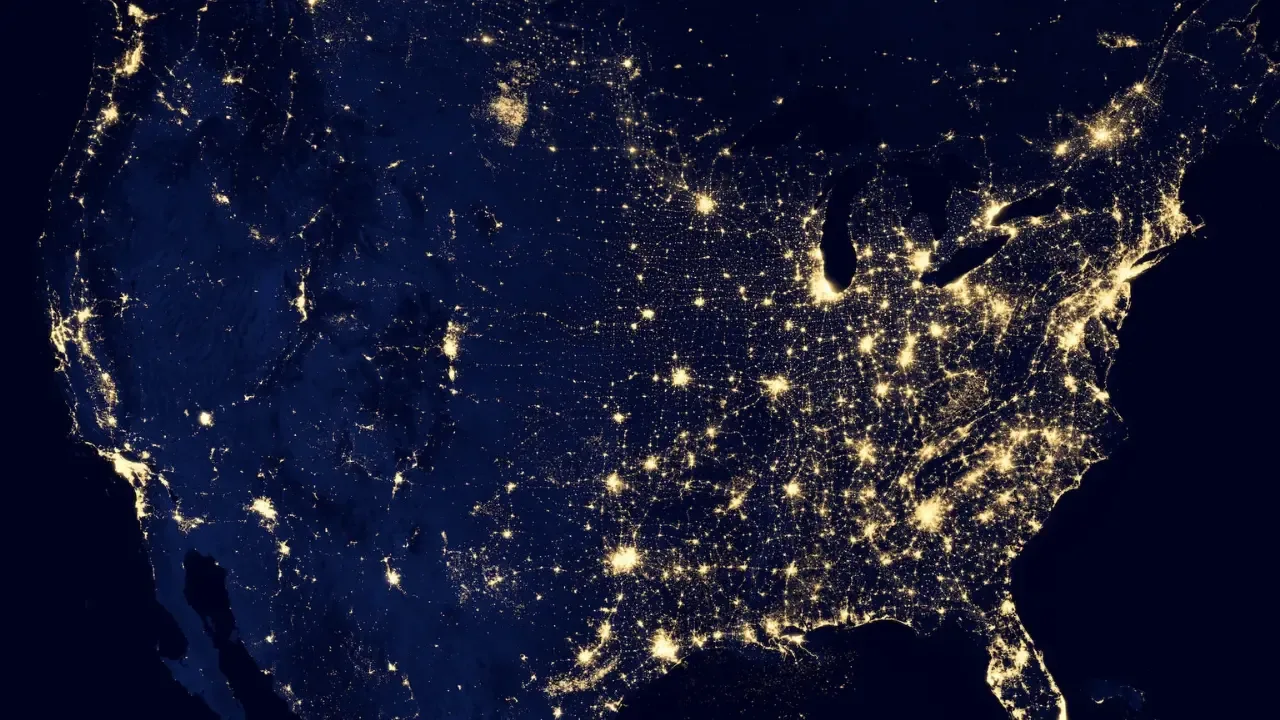
How to Declare Global Variables in Android? 🌐
Are you struggling with declaring global variables in your Android application? 📱 Don't worry, you're not alone! Many developers face issues when it comes to setting variables as global in order to avoid unwanted behaviors like duplicate pop-ups or repeated login forms. In this blog post, we'll walk you through the common problems and provide easy-to-implement solutions. Let's dive in! 💡
The Context: Creating an Application with Login Functionality 🔐
Imagine you're creating an Android application that requires users to log in before accessing its main features. You've already created the main activity and the login activity, and in the main activity's onCreate
method, you've added a condition to check if the user's session is already valid. Here's an example snippet:
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
...
loadSettings();
if(strSessionString == null)
{
login();
}
...
}
You're also using the onActivityResult
method, which gets triggered when the login form finishes. Here's how it looks:
@Override
public void onActivityResult(int requestCode,
int resultCode,
Intent data)
{
super.onActivityResult(requestCode, resultCode, data);
switch(requestCode)
{
case(SHOW_SUBACTICITY_LOGIN):
{
if(resultCode == Activity.RESULT_OK)
{
strSessionString = data.getStringExtra(Login.SESSIONSTRING);
connectionAvailable = true;
strUsername = data.getStringExtra(Login.USERNAME);
}
}
}
}
However, you're experiencing an issue where the login form sometimes appears twice, and it also appears when the phone's keyboard slides. You suspect that the problem lies with the variable strSessionString
not being set correctly.
The Solution: Declaring Global Variables in Android 🚀
To avoid repetitive login forms and unexpected behaviors, you need to declare strSessionString
as a global variable. A global variable is accessible from all parts of your application.
To declare a global variable in Android, you have a few options:
Option 1: Application Class 📦
You can utilize the Application class to store global variables. This class is the base class for maintaining global application states. Here's how you can use it:
Create a new Java class file called
MyApplication
(or any name you prefer).Extend the
android.app.Application
class.
public class MyApplication extends Application {
// Declare your global variables here
public static String strSessionString;
public static boolean connectionAvailable;
public static String strUsername;
}
Update your
AndroidManifest.xml
file to specify your custom application class:
<application
android:name=".MyApplication"
...
>
...
</application>
Now, you can access the global variables anywhere in your application using MyApplication.strSessionString
, MyApplication.connectionAvailable
, and MyApplication.strUsername
.
Option 2: Singleton Class 🕴️
Another approach is to create a Singleton class to hold your global variables. Follow these steps to implement it:
Create a new Java class file called
GlobalVariables
(or any name you prefer).Implement the Singleton pattern by adding a private constructor and a static instance field.
public class GlobalVariables {
private static final GlobalVariables instance = new GlobalVariables();
// Declare your global variables here
public String strSessionString;
public boolean connectionAvailable;
public String strUsername;
private GlobalVariables() {
// Prevent instantiation from outside
}
public static GlobalVariables getInstance() {
return instance;
}
}
Now, you can access the global variables using
GlobalVariables.getInstance().strSessionString
,GlobalVariables.getInstance().connectionAvailable
, andGlobalVariables.getInstance().strUsername
.
Wrapping Up: Avoiding Duplicate Login Forms and Unwanted Pop-ups 🎁
By declaring global variables in your Android application, you can prevent the login form from appearing twice and eliminate unexpected behaviors triggered by keyboard slides. Whether you choose to use the Application class or a Singleton pattern, these solutions will help you maintain the state and accessibility of your variables throughout your application.
Give it a try, implement these solutions, and say goodbye to those annoying login form repetitions! 😊
Have you faced any other Android development hurdles or got any brilliant solutions? Share your experiences and thoughts in the comments below. Let's learn and grow together! 🌟🚀
Happy coding! 💻🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
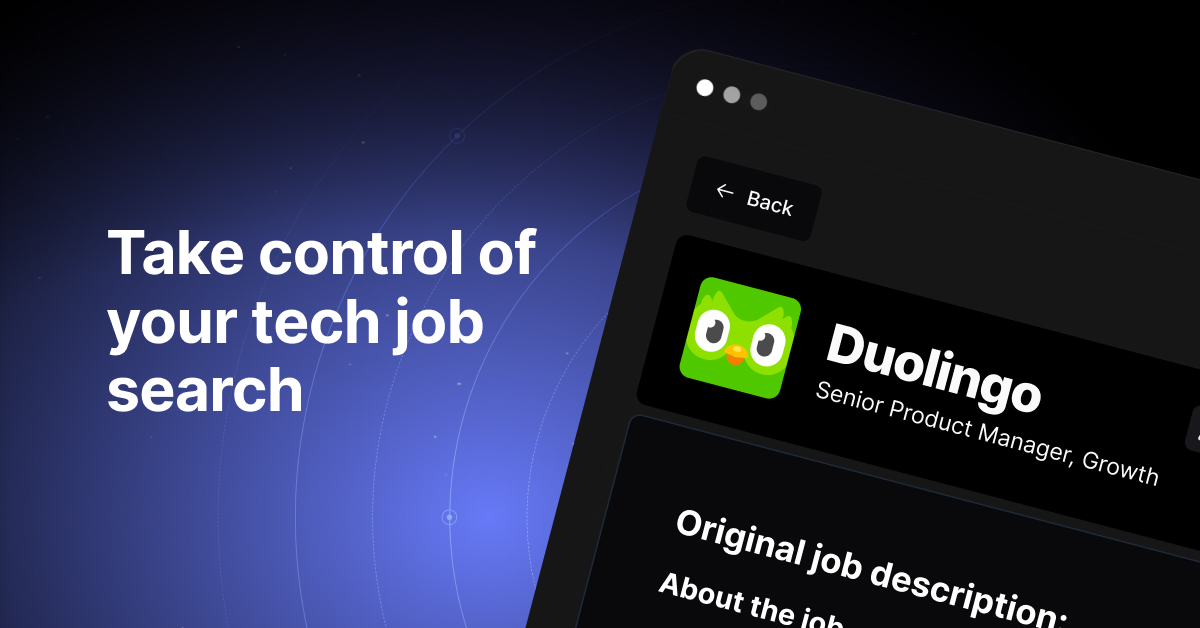