How can I avoid concurrency problems when using SQLite on Android?
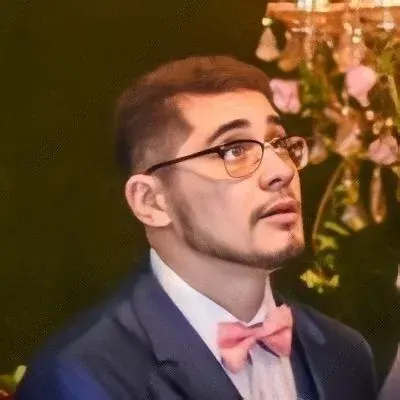
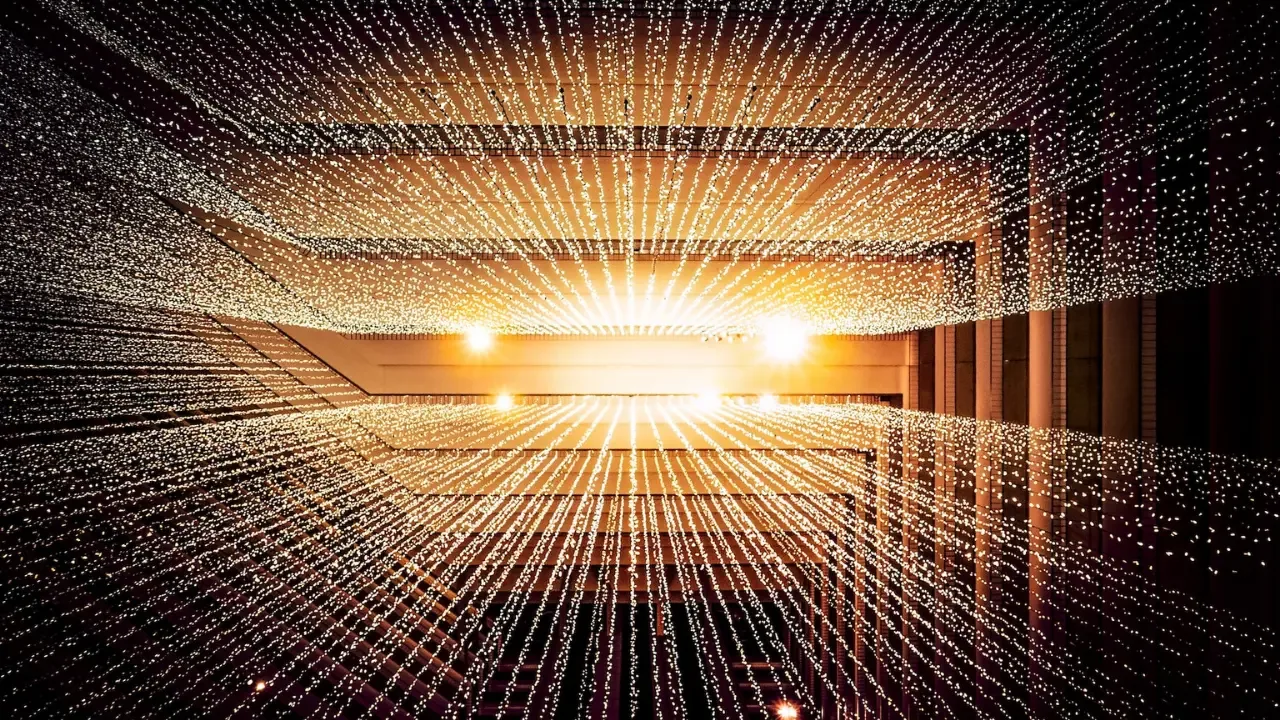
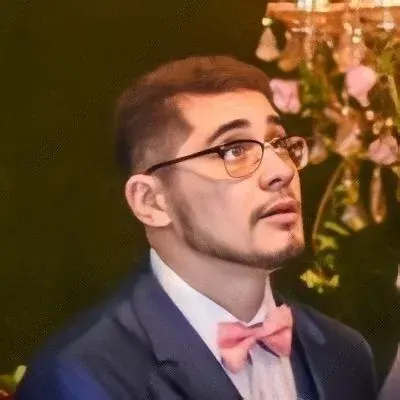
📝 Blog Post: How to Avoid Concurrency Problems with SQLite on Android
Are you developing an Android app and using SQLite as your database? If so, you might be facing some concurrency problems that can lead to slow performance or even crashes. But don't worry, we've got you covered! In this blog post, we will address common issues related to SQLite concurrency on Android and provide easy solutions to avoid them. Let's dive in! 💪📱
Issue: Running queries from the wrong thread
One common mistake when working with SQLite on Android is running database queries on the UI thread. This can cause your app to become unresponsive and result in an Application Not Responding (ANR) error. To avoid this, it's important to perform database operations on a separate thread.
✅ Solution: Use AsyncTask or Background Thread
To prevent concurrency problems, you can execute your SQLite queries within an AsyncTask
or on a background thread. This will ensure that your app remains responsive while performing database operations. Here's an example using an AsyncTask
:
private class QueryTask extends AsyncTask<Void, Void, Void> {
protected Void doInBackground(Void... params) {
// Perform your SQLite queries here
return null;
}
}
By running your queries on a separate thread, you eliminate the risk of blocking the UI thread and improve the overall performance of your app.
Issue: Multiple AsyncTasks and database connections
Another challenge arises when you have multiple AsyncTasks
that need to access the database simultaneously. Should they share a connection or open a new connection each?
🚫 Problem: Sharing a single connection can lead to concurrency issues
If multiple AsyncTasks
share a single SQLite database connection, you might encounter concurrency issues. This can result in data corruption, unexpected errors, or even crashes. It's generally not recommended to share a connection among different threads.
✅ Solution: Open a new connection for each AsyncTask
To avoid concurrency problems, it's best to open a new SQLite connection for each AsyncTask
. This ensures that each task operates independently and avoids any conflicts that might occur when sharing a connection. Here's an example:
private class QueryTask extends AsyncTask<Void, Void, Void> {
protected Void doInBackground(Void... params) {
SQLiteDatabase db = openOrCreateDatabase("your_database.db", SQLiteDatabase.OPEN_READWRITE, null);
// Perform your SQLite queries using this connection
db.close();
return null;
}
}
By opening a new connection for each AsyncTask
, you ensure that they can work concurrently without interfering with each other.
Best practices for SQLite concurrency on Android
To summarize, here are some best practices to avoid concurrency problems when using SQLite on Android:
Execute your SQLite queries on a separate thread using
AsyncTask
or a background thread.Avoid running queries on the UI thread to prevent ANR errors.
Open a new SQLite connection for each
AsyncTask
to avoid concurrency issues.Properly close the connections after executing queries to release system resources.
By following these best practices, you can ensure smooth and efficient database operations in your Android app. Now go ahead and implement these solutions in your code! 💻✨
If you found this guide helpful, make sure to share it with fellow Android developers who might be facing similar challenges. And if you have any questions or suggestions, feel free to leave a comment below. Let's optimize our SQLite concurrency together! 🚀😊