Flutter give container rounded border
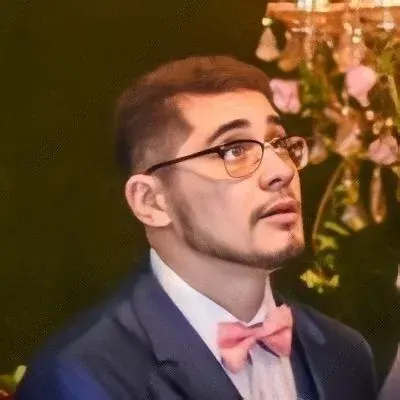
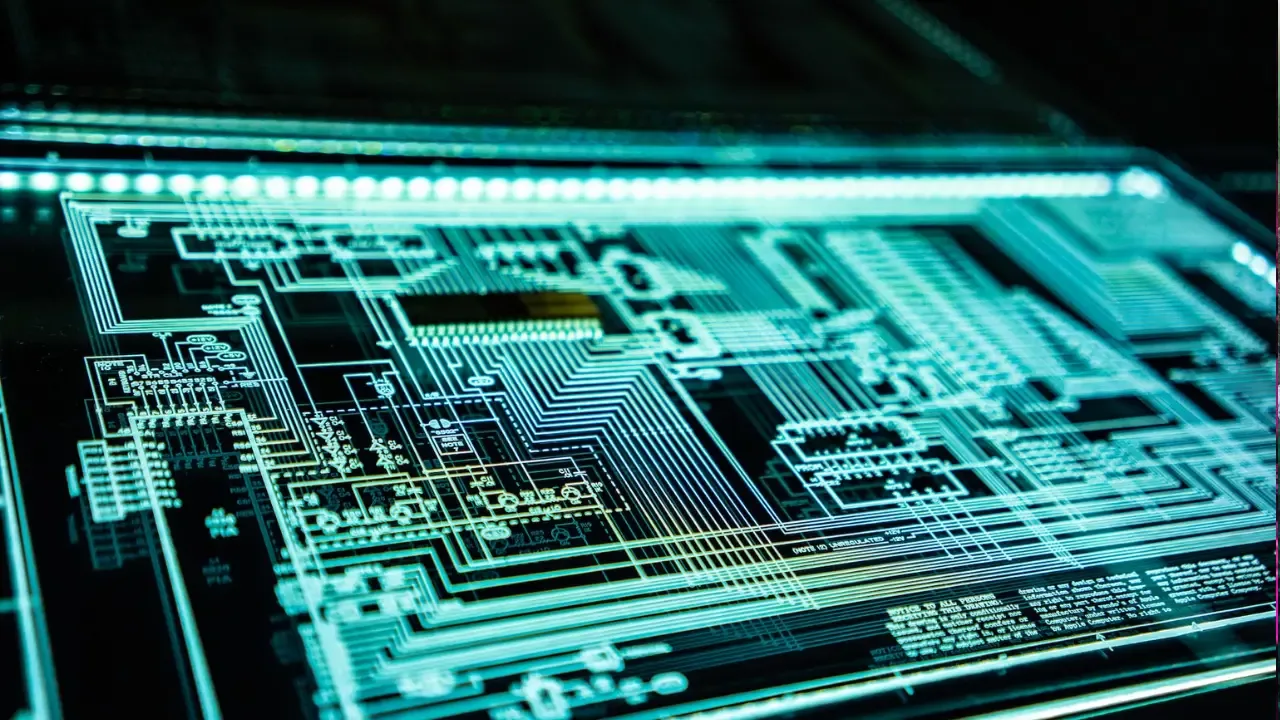
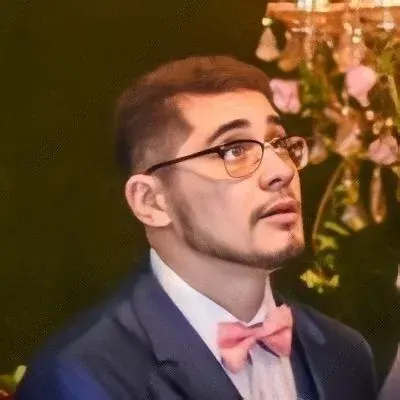
How to Give a Container Rounded Border in Flutter ๐
So you're making a Container()
in your Flutter app and you've added a border. But wouldn't it be nice if the border had rounded corners? ๐ค
Well, you're in luck! In this guide, we'll address this common issue and provide you with easy solutions to give your container a rounded border. Let's dive in! ๐ช
The Problem ๐คจ
The code you currently have sets a border for your Container
, but it lacks rounded corners. Here's a snippet of your existing code:
Container(
width: screenWidth / 7,
decoration: BoxDecoration(
border: Border.all(
color: Colors.red[500],
),
),
...
);
You've tried using ClipRRect
to achieve rounded corners, but unfortunately, that crops the border away. ๐
โโ๏ธ
The Solution ๐
To give your Container
a rounded border, you can utilize the borderRadius
property in the BoxDecoration
class. By specifying a BorderRadius
value, you'll be able to achieve those beautiful rounded corners! ๐
Here's an updated version of your code with the rounded border applied:
Container(
width: screenWidth / 7,
decoration: BoxDecoration(
border: Border.all(
color: Colors.red[500],
),
borderRadius: BorderRadius.circular(15.0),
),
...
);
In the code above, we added borderRadius: BorderRadius.circular(15.0)
to the decoration
property of your Container
. You can adjust the 15.0
value to fit the amount of curvature you desire for your rounded corners. Play around with different values until you find the perfect fit! ๐จ
Conclusion ๐
And that's it! With just a simple addition to your code, you can now have a Container
with rounded borders in your Flutter app. ๐
Remember, the borderRadius
property in the BoxDecoration
class is your secret weapon for achieving those smooth and stylish corners. Don't be afraid to experiment with different values to make your UI truly shine!
Have fun coding and enjoy your new rounded borders! ๐
If you have any questions or suggestions, let me know in the comments below. Happy Fluttering! ๐