AsyncTask Android example
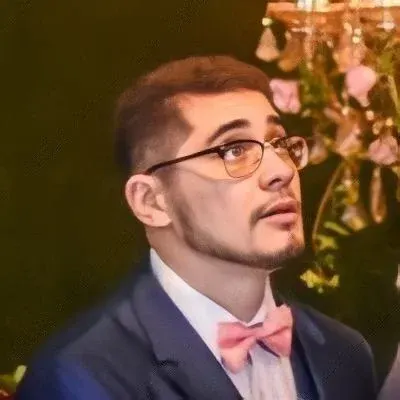
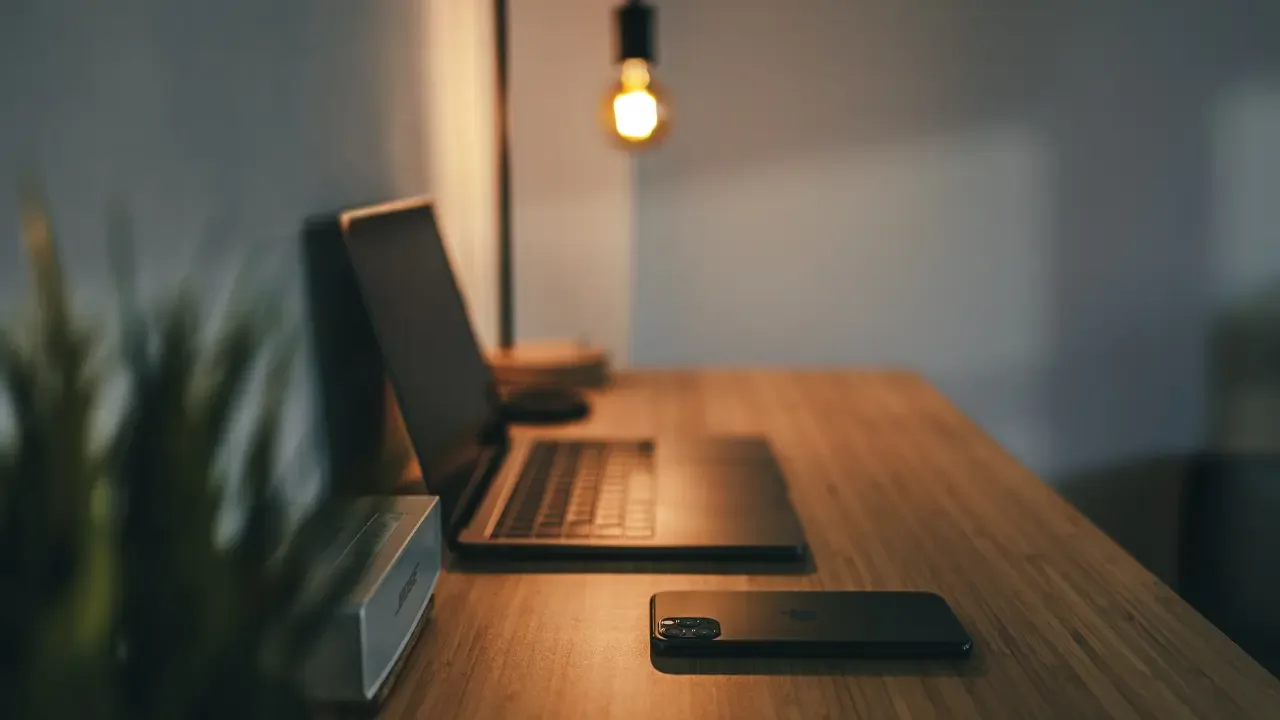
Title: Mastering AsyncTask in Android: A Simplified Guide with Examples 👨💻
Introduction: Are you struggling to make your AsyncTask work in your Android app? Don't worry, I've got you covered! In this blog post, I'll explain the common issues developers face when implementing AsyncTask and provide easy solutions to help you make it work smoothly. 🚀
The Problem: One of our readers encountered an issue with their AsyncTask implementation. They wanted to change a label after a background process completed, but it wasn't working as expected. Let's take a look at their code snippet to understand the problem better. 💡
The Code:
public class AsyncTaskActivity extends Activity {
Button btn;
// onCreate method and other code...
public void onClick(View view){
new LongOperation().execute("");
}
private class LongOperation extends AsyncTask<String, Void, String> {
@Override
protected String doInBackground(String... params) {
// Background operation code to sleep for 5 seconds
// and update the label
return null;
}
// Other methods...
}
}
Explaining the Code:
The code defines an AsyncTask called LongOperation
with a doInBackground
method that is responsible for performing background operations. Inside the doInBackground
method, there's a loop that sleeps for 5 seconds and then tries to update the label. However, this code won't work as expected. 😫
The Solution:
To make this code work and update the label after the background process completes, we need to modify the doInBackground
method and the related UI update code. Instead of updating the label directly in doInBackground
, we'll use the onPostExecute
method, which runs on the main UI thread, to update the label. Let's make the required changes: 🛠️
private class LongOperation extends AsyncTask<String, Void, String> {
@Override
protected String doInBackground(String... params) {
// Background operation code to sleep for 5 seconds
return "Executed";
}
@Override
protected void onPostExecute(String result) {
TextView txt = (TextView) findViewById(R.id.output);
txt.setText(result);
}
// Other methods...
}
In the modified code, we return the string "Executed" from the doInBackground
method and update the label in the onPostExecute
method using this returned string. By updating the UI element in onPostExecute
, we ensure that the label is changed on the main UI thread, avoiding any conflicts. 🎉
Explanation of onPostExecute:
The onPostExecute
method is automatically called after the doInBackground
method completes its execution. It receives the result from the background operation, allowing us to perform any necessary UI updates based on that result. In our example, we set the updated label in the TextView
with the ID output
using the setText
method. 🖍️
Additional Tips and Tricks:
Make sure you call
.execute()
on your AsyncTask instance to start its execution.Use the
onPreExecute
method to perform any necessary setup before the background operation starts.Utilize
onProgressUpdate
to update the UI with progress if you have any intermediate updates during your background operation.
Remember, AsyncTask is a convenient tool to handle background operations in Android, but it's important to use it correctly to avoid unexpected glitches and ensure a smooth user experience. 📱
Conclusion: By understanding the common issues and following the easy solutions provided in this guide, you can now confidently implement AsyncTask in your Android apps. Make your apps more responsive and keep your users engaged while background operations are running. Share your success stories and any other tips you have in the comments below! Let's master AsyncTask together! 💪
Don't forget to share this blog post with your fellow Android developers who might benefit from it. Happy coding, everyone! 🤩🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
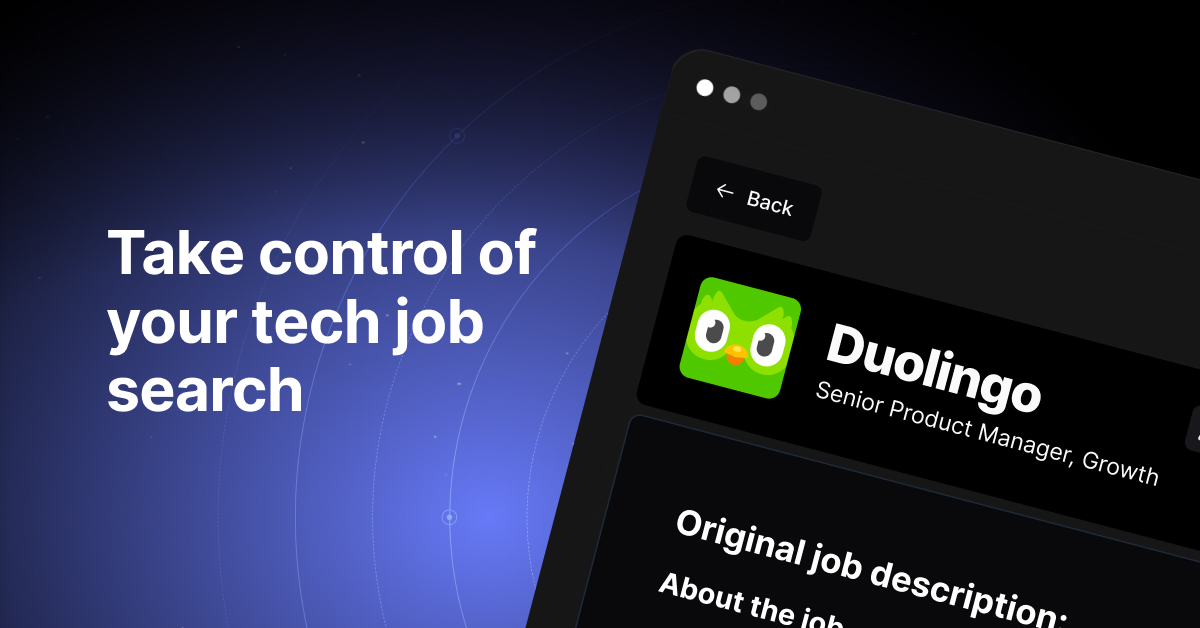