Android layout replacing a view with another view on run time
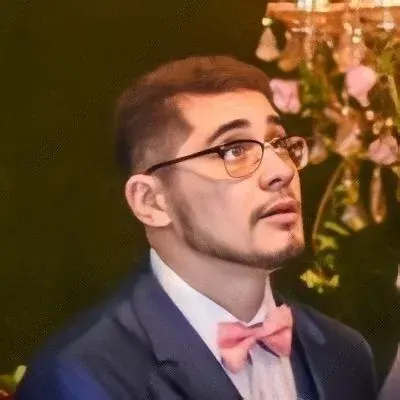
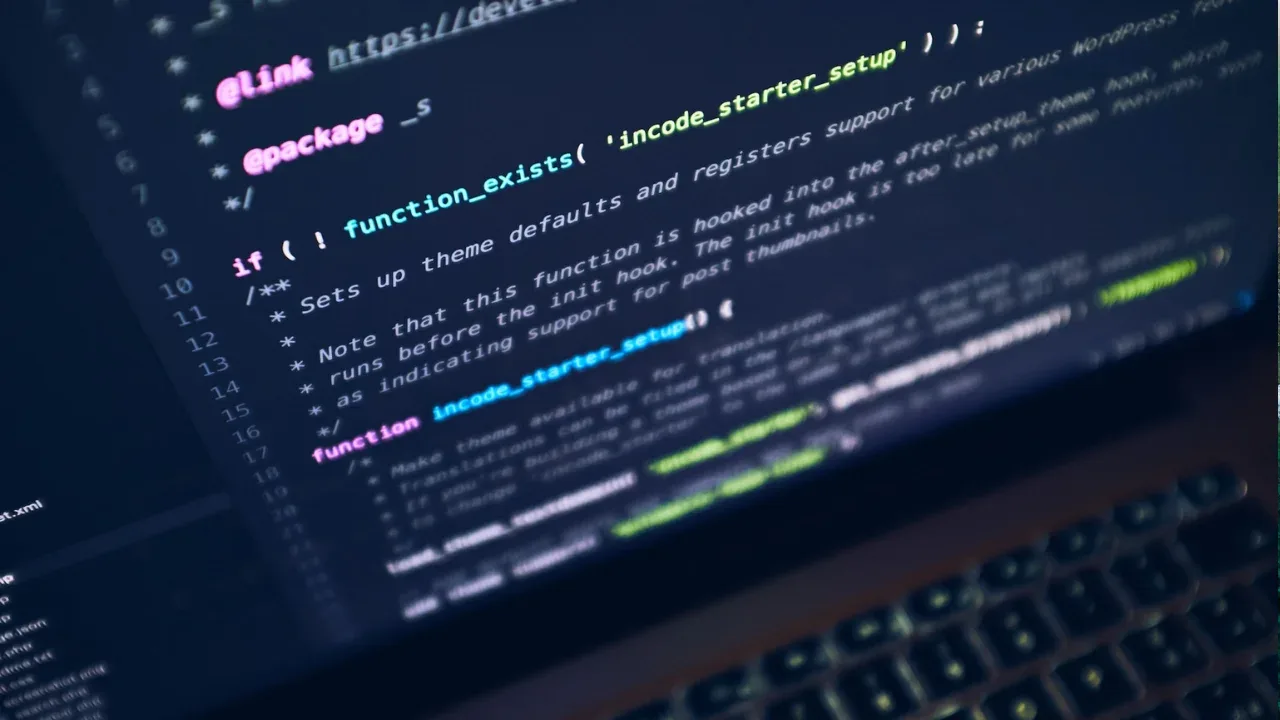
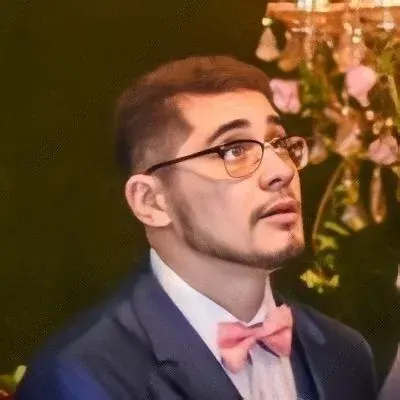
📱 How to Replace an Android View with Another View at Runtime 🔄
Are you looking to dynamically switch between different views in your Android layout at runtime? 🤔
Say you have a layout file called main.xml
, which contains two TextViews, A and B, as well as a placeholder view C. And you have two other layout files, option1.xml
and option2.xml
, each representing a different view you want to load into C.
The question is: Can you load either option1.xml
or option2.xml
into C programmatically using Java? And if so, what function do you need to use? 🤔
Hang tight, my tech-savvy friend! I've got you covered with easy solutions and helpful tips! 💪
1. Using the LayoutInflater 🏗️
To load a layout file dynamically, you can utilize the LayoutInflater
class provided by Android. This handy class allows you to create instances of XML layouts in your code.
Let's dive into the step-by-step process to achieve our goal. 🏊♀️
Step 1: Instantiate the LayoutInflater
First, you need to create an instance of the LayoutInflater
class. You can use the getLayoutInflater()
method, available from any activity or fragment.
LayoutInflater inflater = getLayoutInflater();
Step 2: Inflate the desired layout
Using the inflate()
method of the LayoutInflater
instance, you can inflate the XML layout of your choice.
View newView = inflater.inflate(R.layout.option1, null);
Make sure to replace R.layout.option1
with the proper layout resource identifier for option1.xml
or option2.xml
, depending on your needs.
Step 3: Replace the placeholder view
Finally, you need to replace the existing view C with the newly inflated view.
ViewGroup container = findViewById(R.id.viewCContainer);
container.removeAllViews();
container.addView(newView);
Here, R.id.viewCContainer
refers to the ID of the view container where you want to place the newly inflated view. Replace it with the correct ID from your layout file.
2. Putting it All Together 🎉
To wrap it up, let's see the complete code snippet that brings everything together:
LayoutInflater inflater = getLayoutInflater();
View newView = inflater.inflate(R.layout.option1, null);
ViewGroup container = findViewById(R.id.viewCContainer);
container.removeAllViews();
container.addView(newView);
That's it! You can now switch between different views in real-time using the above code. It's as simple as that! 🎈
🌟 Your Turn to Shine! 🌟
Now that you know how to replace an Android view with another view at runtime, why not give it a try in your own projects? Get creative and experiment with different layouts and dynamic views!
If you have any questions or want to share your experience, feel free to leave a comment below. I'd love to hear from you! 😄
So go ahead, code and conquer! 🚀