Why does Math.Round(2.5) return 2 instead of 3?
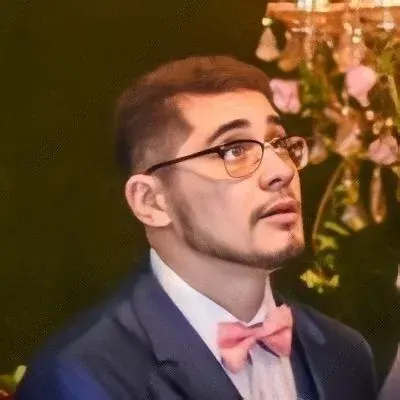
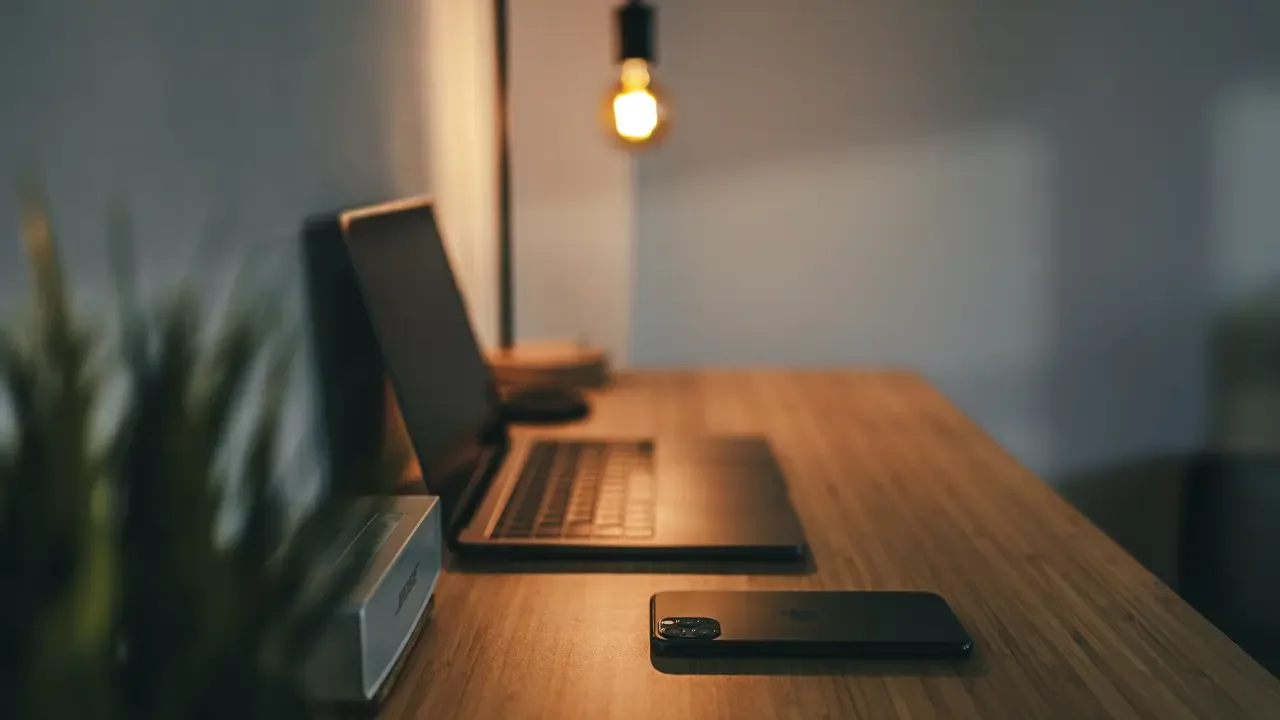
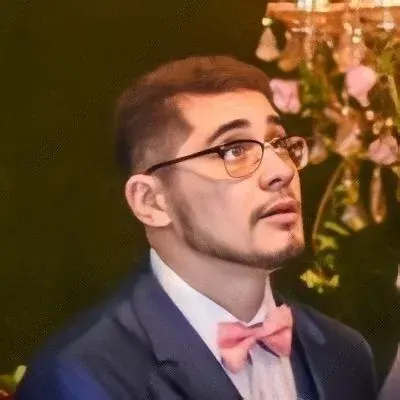
Why does Math.Round(2.5)
return 2 instead of 3? 🤔
You're not alone if you've been puzzled by the result of Math.Round(2.5)
in C#, expecting it to be 3 but getting 2 instead. Let's dive into the mysterious world of rounding numbers and understand why this happens, plus how to address this common issue. 💡
Understanding the rounding algorithm
To comprehend why Math.Round(2.5)
returns 2, we need to understand how the rounding algorithm works. When a number is exactly halfway between two other numbers, the rounding rule depends on the number's "evenness" or "oddness." If the number is even, it's rounded down; if it's odd, it's rounded up.
In our case, 2.5 is not an even number, so it gets rounded up to the nearest whole number, which is 3. However, C# (and several other programming languages) use a different rounding strategy called "round half to even" or "round half to nearest even." 🔄
The round half to even strategy
The round half to even strategy takes into account both the number being rounded and the digit to its left. Specifically, if the digit to the left of the rounding position is even, the number is rounded down. But if it's odd, the number is rounded up. This technique is used to ensure that rounding is statistically fair, preventing any bias caused by consistently rounding up or down.
In our scenario, 2 is an even number (digit to the left of the rounding position), so the result becomes 2 instead of 3. 😮
Getting the expected result
If you want to obtain 3 as the result of Math.Round(2.5)
, there are a few solutions available. Here are some options you can consider:
Option 1: Use Math.Ceiling()
Math.Ceiling()
is a method that always rounds numbers up to the nearest whole number, regardless of the decimal portion. By simply replacing Math.Round()
with Math.Ceiling()
, the code Math.Ceiling(2.5)
will indeed return 3.
int result = (int)Math.Ceiling(2.5); // result = 3
Option 2: Implement custom rounding logic
If you need more control over the rounding process, you can create a custom method that fits your specific requirements. This can be as simple as adding 0.5 to the number and then applying Math.Floor()
to round it down. Here's an example:
int CustomRound(double number)
{
return (int)(number + 0.5);
}
int result = CustomRound(2.5); // result = 3
Join the discussion! 💬
Have you ever been confused by rounding algorithms in your programming journey? Share your thoughts, experiences, and any additional tips or tricks you've discovered with our community in the comments below. Let's learn from each other! 👥
Remember, understanding how rounding works is crucial for preventing unexpected results and ensuring your code behaves as intended. Now that you're aware of the intricacies behind Math.Round(2.5)
and how to work around it, you can confidently tackle rounding challenges in C# and beyond. Happy coding! 😄🚀