What is the difference between IQueryable<T> and IEnumerable<T>?
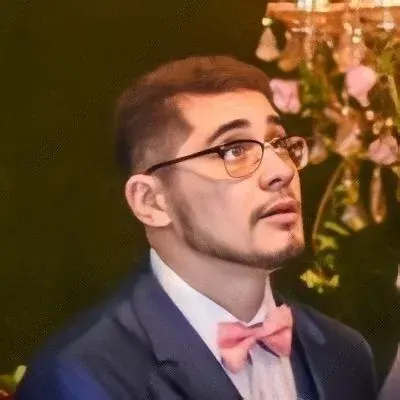
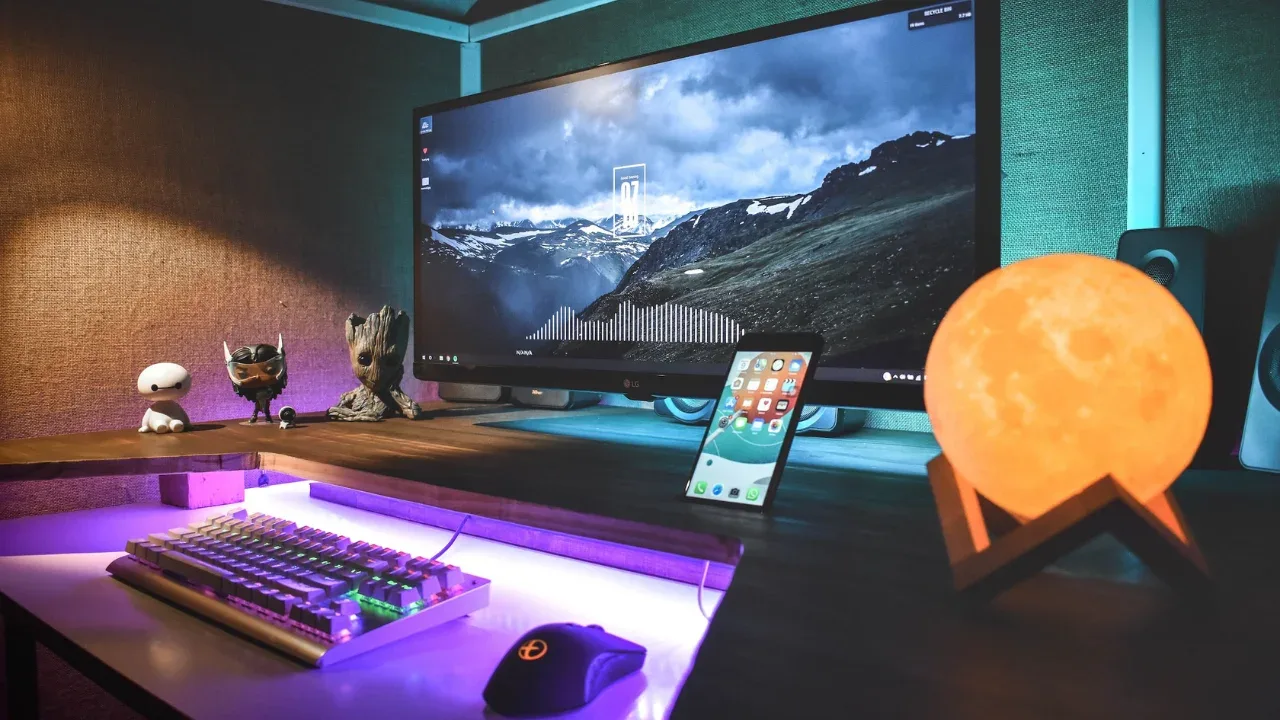
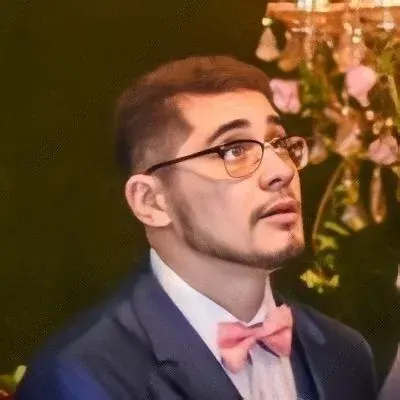
IQueryable vs. IEnumerable: Understanding the Crucial Difference
š¢ Hey tech enthusiasts! Are you puzzled by the difference between IQueryable<T>
and IEnumerable<T>
? Don't worry, you're not alone! š¤ These two guys may seem similar at first glance, but trust me, they have their own tricks up their sleeves. In this blog post, we'll break down their differences, address common issues, and provide easy solutions that will make your coding life a walk in the park. Grab your coffee āļø, sit back, and let's dive in! šŖ
What's the Context?
So, you stumbled upon a code snippet that contains this question: "What is the difference between IQueryable<T>
and IEnumerable<T>
?" Let's unravel this mystery together! šÆāāļø
IQueryable<T>
: The Queryable Powerhouse
Let's start with the player in the blue corner, IQueryable<T>
. š This interface is all about writing expressive queries that can be executed against a data source like a database š¾, using a query provider. š
IEnumerable<T>
: The Enumerable All-rounder
Now, let's meet the contender in the red corner, IEnumerable<T>
. ā¤ļø This interface is all about working with in-memory collections šļø, where you can perform common operations like filtering, sorting, and projecting, using LINQ or other methods.
Key Differences
1ļøā£ Query Execution: The main difference lies in when and where the query is executed. IQueryable<T>
represents a query that will be executed directly against a data source, like a database, when evaluated. On the other hand, IEnumerable<T>
operates on in-memory collections and executes the query immediately upon enumeration.
2ļøā£ Deferred Execution: IQueryable<T>
supports deferred execution, meaning the query is not executed until the results are enumerated. This allows you to compose and modify the query as needed before execution. Conversely, IEnumerable<T>
does not inherently support deferred execution, as it evaluates the query immediately upon enumeration.
3ļøā£ Expression Trees: IQueryable<T>
uses expression trees to represent queries. This allows query providers to inspect and transform the query before execution, enabling powerful optimizations. On the contrary, IEnumerable<T>
operates on objects, without the ability for query providers to analyze and optimize the query.
Let's Illustrate With Examples
Example 1: Querying a Database
// IQueryable<T> example
IQueryable<Customer> customersQuery = dbContext.Customers.Where(c => c.City == "New York");
// IEnumerable<T> example
IEnumerable<Customer> customers = dbContext.Customers.Where(c => c.City == "New York").ToList();
With the IQueryable<T>
approach, the query is transformed into SQL and executed directly against the database. This ensures that only the relevant data is retrieved, delivering better performance. On the other hand, the IEnumerable<T>
approach retrieves all the data from the database and performs the filtering in-memory, potentially causing unnecessary network traffic.
Example 2: Modifying a Query
// IQueryable<T> example
IQueryable<Customer> query = dbContext.Customers.Where(c => c.IsActive);
if (someCondition)
{
query = query.OrderBy(c => c.Name);
}
else
{
query = query.OrderBy(c => c.Age);
}
var result = query.ToList();
In this example, the IQueryable<T>
query allows us to conditionally modify the sorting behavior before execution. With IEnumerable<T>
, achieving the same effect would require materializing the results and performing the conditional operation on an in-memory collection.
Common Pitfalls and Easy Solutions
1ļøā£ Queries Not Executing as Expected: If your IQueryable<T>
query is not producing the desired results, ensure that you've enumerated the results using methods like .ToList()
, .First()
, or .Count()
. This forces the execution of the query and retrieves the desired data.
2ļøā£ Performance Bottlenecks: If you notice your IEnumerable<T>
queries become slow for large datasets, consider switching to IQueryable<T>
to leverage the optimizations provided by the query provider. This can significantly improve performance.
Conclusion: The Choice is Yours!
Now that you understand the difference between IQueryable<T>
and IEnumerable<T>
, it's time to put this knowledge into practice! Choose wisely based on your specific needs and maximize the potential of your queries. š”š
Feel free to share your experiences or ask any questions in the comments below. We'd love to hear your thoughts! Let's level up our coding skills together! š©āš»šØāš»
š Don't forget to check out this Stack Overflow post for more insights.
Call-to-Action: Join the Discussion!
Now it's your turn to join the conversation! Have you encountered any hurdles while dealing with IQueryable<T>
or IEnumerable<T>
? Share your experiences, questions, or even your favorite coding memes in the comments section below. Let's learn from each other and harness our collective expertise! šš¬
Keep coding and stay curious! šāØ