What is the best algorithm for overriding GetHashCode?
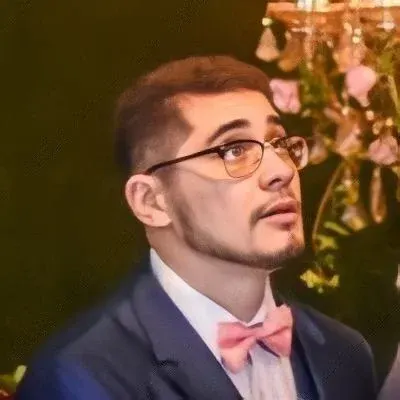

💡 The Best Algorithm for Overriding GetHashCode in .NET
So, you're wondering about the best algorithm for overriding GetHashCode
in your custom classes? You've come to the right place! 🙌
In .NET, the GetHashCode
method plays a crucial role in various parts of the framework. It helps in quickly finding items in collections and determining equality. Implementing it properly is essential to ensure optimal performance. 🏎️
The good news is that there isn't just one specific algorithm or standard approach for all scenarios. It depends on the nature of your custom class and the properties you want to consider for generating the hash code. Let's dive deeper and explore some common issues and easy solutions! 💡
The Problem 😓
When you create a custom class, you may face issues where multiple instances of the class end up with the same hash code. This phenomenon is called a "hash collision." And hash collisions can negatively impact the performance of hash-based collections like dictionaries and hash sets. 😕
Now, you might be wondering, "How can I override GetHashCode
to avoid such collisions?" 🤔
The Solution 🚀
Fortunately, you can follow a few best practices to minimize the chances of hash collisions and enhance the performance of your program:
Consider important properties
Think about the properties in your class that uniquely identify objects or significantly influence equality comparisons. For example, if you have a Person
class with FirstName
, LastName
, and Age
properties, you probably want to include all of them in your hash code calculation.
Use prime numbers
Generally, it's a good practice to multiply the current hash code by a prime number and then combine it with the hash code of the next property. This helps to distribute the hash codes more evenly and reduce collisions. 🔄
public override int GetHashCode()
{
int hash = 17; // Start with a prime number
hash = hash * 23 + FirstName.GetHashCode();
hash = hash * 23 + LastName.GetHashCode();
hash = hash * 23 + Age.GetHashCode();
return hash;
}
Be consistent with Equals
Remember, GetHashCode
and Equals
should go hand in hand. If two objects are equal, they must have the same hash code. Hence, ensure that you override the Equals
method accordingly. ♻️
Use hash-agnostic libraries
If you don't want to worry about implementing GetHashCode
and Equals
for each custom class, you can rely on hash-agnostic libraries like C# record types or third-party libraries like FastDeepCloner. These tools handle the hash code generation for you. 🛠️
Your Turn! ✍️
Now that you have a good understanding of the considerations for overriding GetHashCode
, it's time to put this knowledge into practice! Take a look at your custom classes and ensure that you've implemented the method correctly. Improve the performance of your collections and prevent nasty hash collisions. 💪
If you have any questions or want to share your experience, drop a comment below. Let's have an engaging discussion! ⬇️
Happy coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
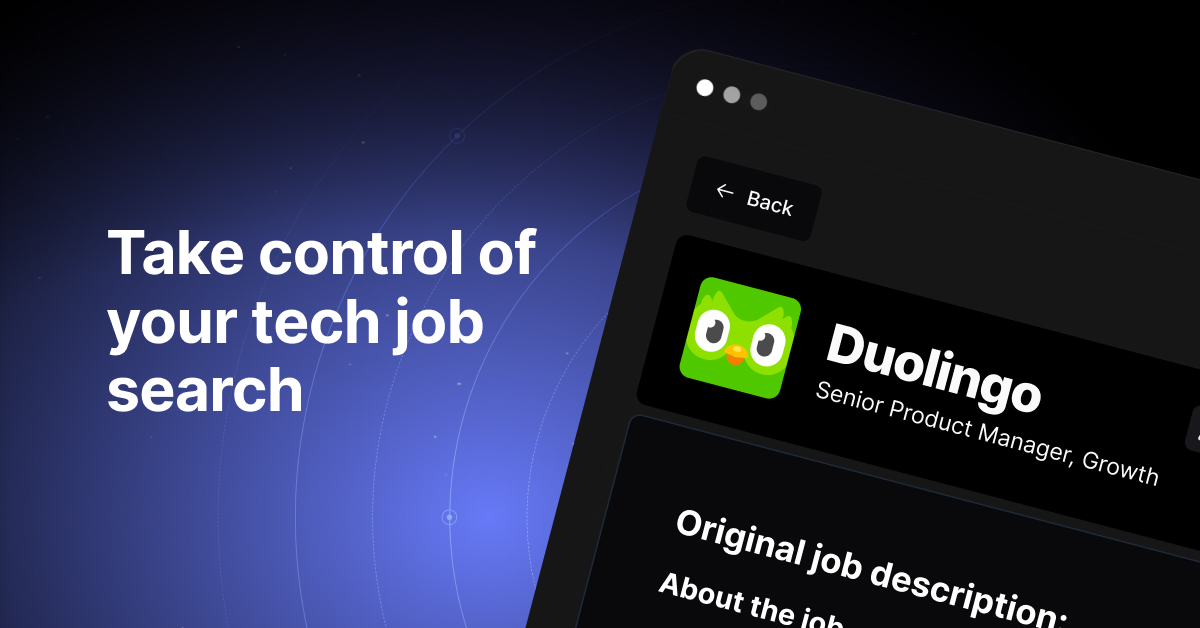