Open directory dialog
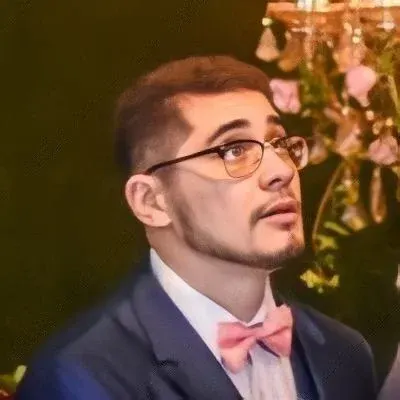
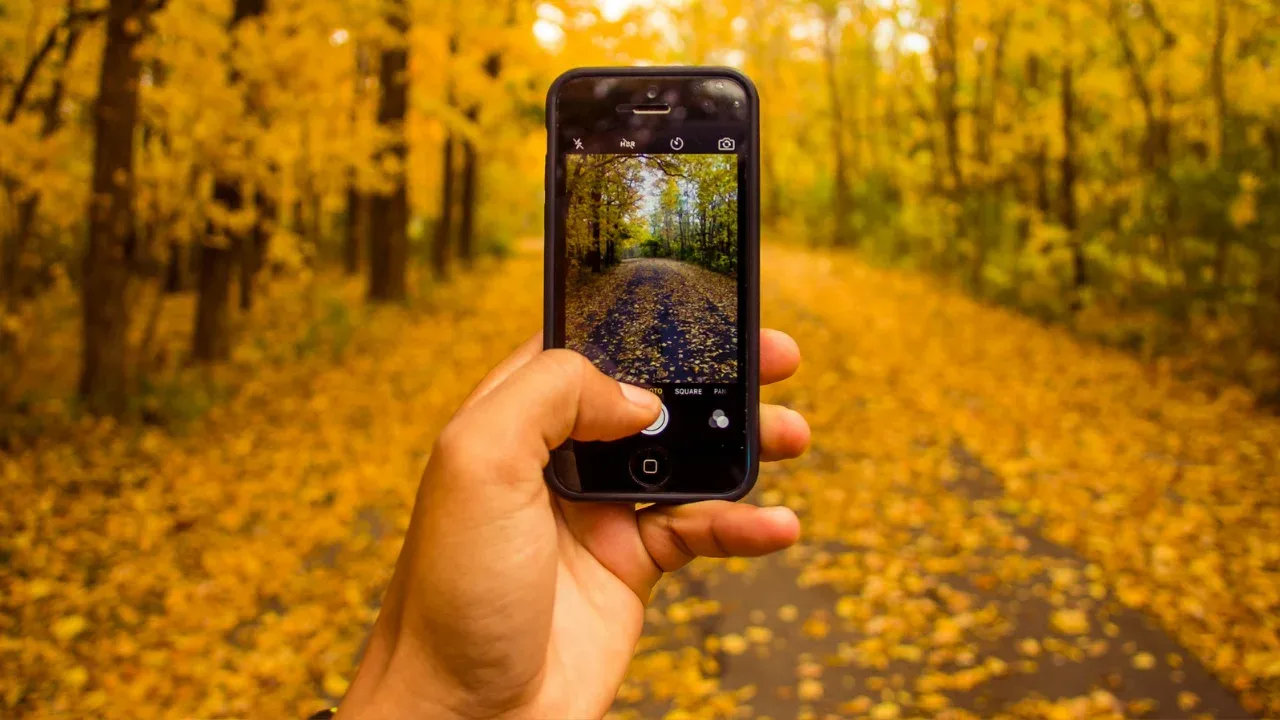
Open Directory Dialog: How to Select a Directory in WPF?
š As developers, we often encounter situations where we need the user to select a directory for various file operations. In WPF, the widely-used OpenFileDialog
from Win32 is a popular choice. However, it poses a common challenge - it requires the selection of a file, which can be confusing and counterintuitive for users who only want to choose a directory. But worry not, we've got you covered with some easy solutions!
The Problem: OpenFileDialog and the Requirement for File Selection
The OpenFileDialog
is a useful tool for selecting files, but it's not specifically designed for choosing directories alone. By default, it demands at least one file selection before it allows the user to proceed. This creates a roadblock for developers who want to minimize complexity and provide a straightforward directory selection experience.
The Unintuitive Approach: "Hacking Up" Functionality
One possible solution, as mentioned in the context above, is to ask the user to pick a file and then extract the directory path from it. While this approach technically works, it can be confusing and unintuitive for the end-user. Therefore, we should explore alternative methods that provide a better user experience.
Easy Solutions to Select a Directory in WPF
Solution 1: Using FolderBrowserDialog
One of the simplest and most straightforward ways to accomplish directory selection in WPF is by using the FolderBrowserDialog
, which is available in the System.Windows.Forms
namespace. Here's a step-by-step guide to implementing it:
Add the reference to the
System.Windows.Forms
assembly if it's not already present in your project.Import the namespace using the following directive:
using System.Windows.Forms;
Create an instance of the
FolderBrowserDialog
:
var folderBrowserDialog = new FolderBrowserDialog();
Open the dialog and retrieve the selected directory:
if (folderBrowserDialog.ShowDialog() == DialogResult.OK)
{
string selectedDirectory = folderBrowserDialog.SelectedPath;
// Use the selected directory as needed
}
Solution 2: Using Third-Party Libraries
If you prefer a more customizable and aesthetically-pleasing solution, you can explore third-party libraries specifically designed to address this challenge. Some popular choices include:
These libraries provide pre-built components or controls that simplify directory selection and enhance the overall user experience.
Your Turn: Engage and Share!
Now that you've learned some easy solutions for selecting a directory in WPF, it's time to put them into practice. Choose the method that best suits your needs and give it a try. If you encounter any issues or have additional insights to share, feel free to leave a comment below. Don't forget to spread the knowledge among your fellow developers by sharing this post on your favorite platforms!
š©āš» Happy coding and swift directory selection!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
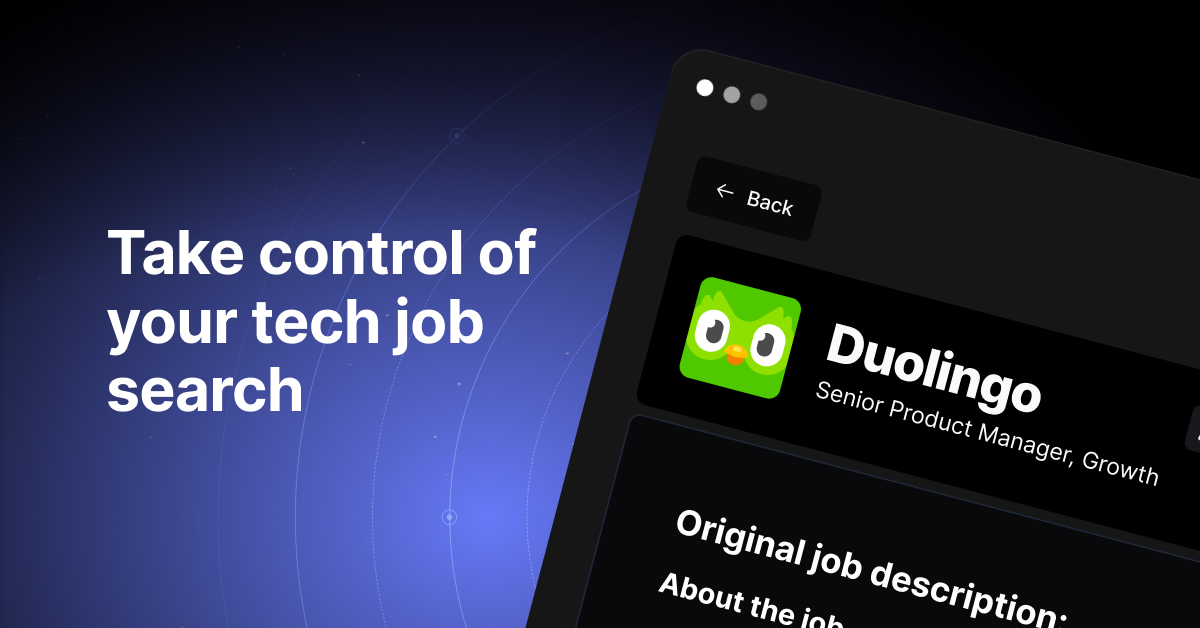