Is it better to call ToList() or ToArray() in LINQ queries?
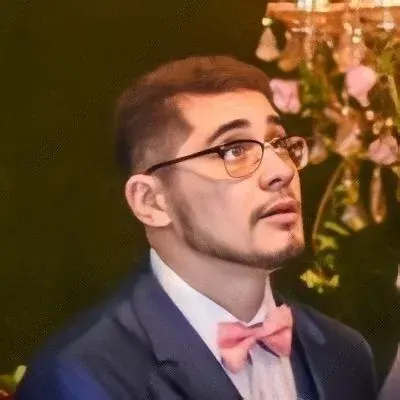
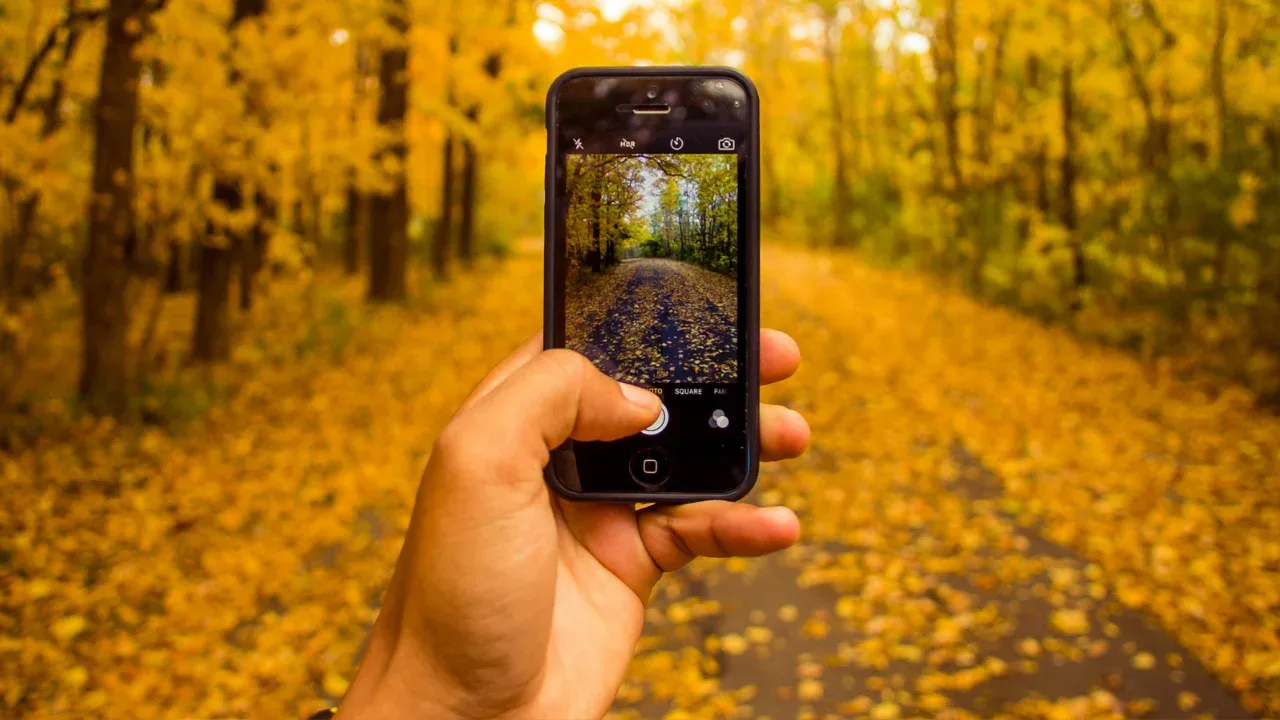
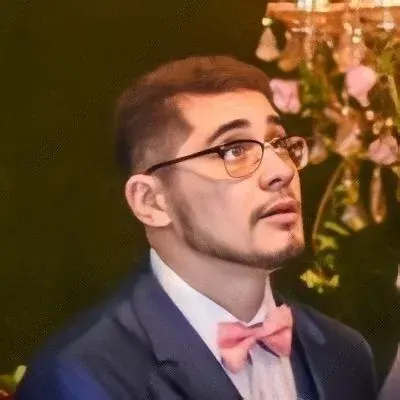
ToList() or ToArray(): Which should you choose?
So you're working with LINQ queries in C# and you've stumbled upon a dilemma: Should you use ToList()
or ToArray()
? π€
The Problem
Let's begin by understanding the scenario. You have a LINQ query that you want to evaluate right where you declare it. This might be because you need to iterate over it multiple times and the computation is expensive. Here's an example to illustrate:
string raw = "...";
var lines = (from l in raw.Split('\n')
let ll = l.Trim()
where !string.IsNullOrEmpty(ll)
select ll).ToList();
This code works perfectly fine. However, if you're not planning to modify the result and just want to iterate over it, you might consider using ToArray()
instead of ToList()
. But before making a decision, let's dive into the underlying implementation details.
The Concern
Your concern is whether ToArray()
is implemented by first calling ToList()
, making it less memory efficient because it allocates memory twice. Valid question, but fear not! π¦ΈββοΈ
The Solution
When you call ToArray()
, it doesn't internally use ToList()
. The LINQ query will be directly converted into an array. Hence, calling ToArray()
is memory efficient and avoids any unnecessary memory allocation.
Therefore, you can confidently use ToArray()
if you're sure that you won't need to modify the result later on.
Here's the modified code using ToArray()
:
string raw = "...";
var lines = (from l in raw.Split('\n')
let ll = l.Trim()
where !string.IsNullOrEmpty(ll)
select ll).ToArray();
But wait, there's more!
Now that you know the difference between ToList()
and ToArray()
, you might wonder when to use each of them. Let's break it down:
Use
ToList()
when you need a dynamically sized list that you might modify later on.Use
ToArray()
in situations where you only need to iterate over the result and won't be making any modifications.
Wrap up and Engage!
So, you're not crazy after all! Calling ToArray()
is perfectly safe and won't allocate memory twice like ToList()
usually does. Feel free to choose whichever method suits your needs.
Now it's your turn to share your thoughts! Which method do you prefer to use in LINQ queries? Let me know in the comments section below! π
Remember, sharing is caring! If you found this blog post helpful, don't forget to share it with your friends and colleagues who might be struggling with the same dilemma. Let's spread the knowledge together! πͺ
#LINQ #CSharp #CodingTips #MemoryEfficiency