How do you unit test private methods?
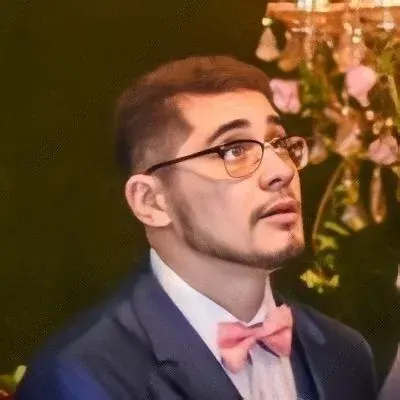
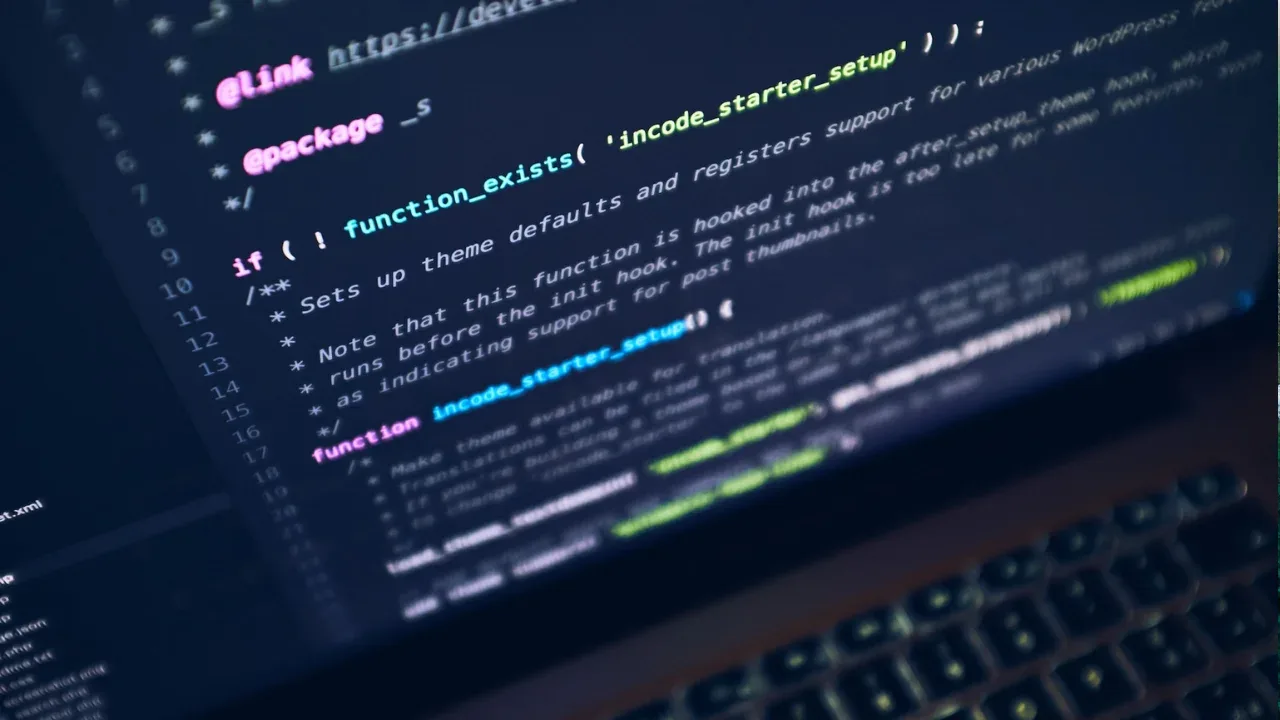
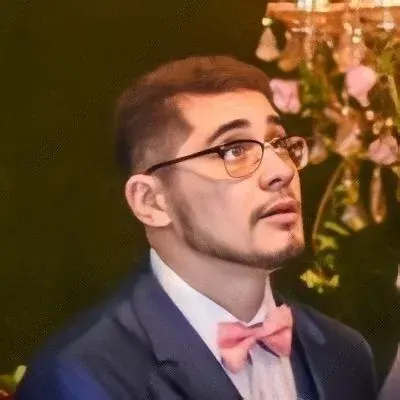
How to Unit Test Private Methods: A Developer's Guide 👨💻
So, you're building a class library with some public and private methods, but you're wondering how to effectively unit test those private methods. You're in luck! In this blog post, we will explore common issues faced when unit testing private methods, provide easy solutions, and encourage reader engagement. Let's get started! 🚀
The Dilemma: Why Unit Test Private Methods?
Before delving into the solutions, let's address the question itself: why would you want to unit test private methods? While it's true that unit testing usually focuses on public methods, there are a few valid reasons to consider testing private methods:
Development Time: Testing private methods can help during development by isolating and validating smaller code components.
Future Refactoring: By having unit tests for private methods, you gain confidence in refactoring without worrying about unintentional side effects.
Corner Cases: Some behavior may be too complex to test indirectly through public methods alone. Testing private methods allows us to tackle these edge cases directly.
Solution 1: Making Private Methods Public 📢
One straightforward approach to test private methods is to temporarily make them public during testing. While this may feel counterintuitive (after all, they're private for a reason, right?), it can be a quick and effective solution.
Here's an example using Java's Reflection API:
public class MyClass {
// ...
private int myPrivateMethod(int x) {
// ...
}
// ...
}
// Test class
public class MyClassTest {
public void testMyPrivateMethod() throws Exception {
MyClass myClass = new MyClass();
Method method = MyClass.class.getDeclaredMethod("myPrivateMethod", int.class);
method.setAccessible(true);
int result = (int) method.invoke(myClass, 42);
// Assert result and continue with other tests
}
}
By temporarily making the private method accessible using setAccessible(true)
, we can test it directly.
Solution 2: Refactoring for Testability 📝
If making private methods public doesn't appeal to you, fear not! We have an alternative approach: refactoring. By extracting the private logic to separate methods or collaborating classes, we make them publicly accessible and easily testable.
Consider the following example:
public class MyClass {
// ...
private int myPrivateMethod(int x) {
// ...
}
public int myPublicMethod(int x) {
return myPrivateMethod(x * 2);
}
// ...
}
// Test class
public class MyClassTest {
public void testMyPublicMethod() {
MyClass myClass = new MyClass();
int result = myClass.myPublicMethod(42);
// Assert result and continue with other tests
}
}
By testing the public method that calls the private method, we indirectly test the private method's behavior.
📣 Engage with the Community: Share Your Thoughts and Challenges!
We hope this guide helped you understand the options for unit testing private methods. Now, it's time for your voice to be heard! 🗣️
Do you unit test private methods in your projects? What challenges have you faced? Are there any solutions we missed? Share your thoughts in the comments section below and let's start a conversation! Together, we can expand our knowledge and create better software. 💪
That's all for today, folks! 🎉 Don't forget to hit the "Share" button to spread the knowledge! 📲
Happy testing, and may the code be with you! ✨