How do you get a string from a MemoryStream?
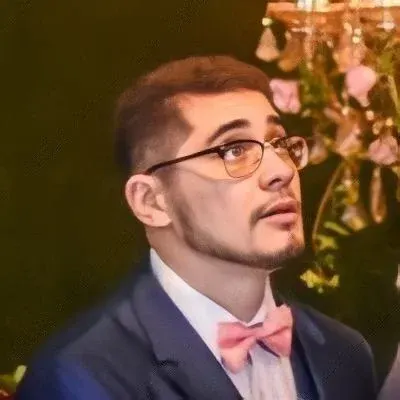

How to Get a ๐งต String from a ๐พ MemoryStream?
So, you've got this super cool ๐ฝ MemoryStream filled with some awesome ๐ฅ data, and you suddenly realize that you need to extract a ๐งต string from it. Don't worry, my tech-savvy friend, because I've got you covered! In this blog post, I'm going to show you how to easily solve this conundrum and get that precious string you desire. ๐
The Problem ๐ค
The challenge here is that a MemoryStream is typically used to store binary data ๐ฑ, and you need to extract a string from it. You might be thinking, "How in the world am I supposed to do this?" Fear not! We have some easy solutions for you! ๐
Solution 1: Using Encoding ๐
One way to tackle this problem is by leveraging the power of โ๏ธ encoding. Since a string is a sequence of characters, encoding allows us to convert a byte sequence into a corresponding string.
using System;
using System.IO;
using System.Text;
// ...
MemoryStream memoryStream = /* Your MemoryStream goes here */;
string extractedString = Encoding.UTF8.GetString(memoryStream.ToArray());
Console.WriteLine(extractedString);
In this solution, we are using the Encoding.UTF8
encoding to convert the byte array representation of the MemoryStream into the corresponding string using the GetString
method. ๐ฉ
Example:
Let's say we have a MemoryStream containing the byte array representation of the string "๐ Hello, World!". Using Solution 1, we can obtain the string like this:
MemoryStream memoryStream = new MemoryStream(new byte[] { 240, 159, 145, 139, 32, 72, 101, 108, 108, 111, 44, 32, 87, 111, 114, 108, 100, 33 });
string extractedString = Encoding.UTF8.GetString(memoryStream.ToArray());
Console.WriteLine(extractedString);
Output:
๐ Hello, World!
Mission accomplished! You've successfully obtained the desired string using Solution 1. But wait, there's more! ๐
Solution 2: Using StreamReader ๐
Another cool way to extract that string from the MemoryStream is by utilizing a StreamReader. A StreamReader provides convenient methods for reading different types of data, including strings.
using System;
using System.IO;
using System.Text;
// ...
MemoryStream memoryStream = /* Your MemoryStream goes here */;
memoryStream.Position = 0; // Reset the position for reading from the start
using StreamReader streamReader = new StreamReader(memoryStream, Encoding.UTF8);
string extractedString = streamReader.ReadToEnd();
Console.WriteLine(extractedString);
In this solution, we set the position of the MemoryStream to the beginning using memoryStream.Position = 0
. Then, we create a StreamReader, passing in the MemoryStream and the desired encoding (Encoding.UTF8
in this example). Finally, we use the ReadToEnd
method to obtain the string from the StreamReader. Voila! ๐
Example:
Let's revisit our previous example of a MemoryStream containing the byte array representation of the string "๐ Hello, World!". Using Solution 2, we can extract the string as follows:
MemoryStream memoryStream = new MemoryStream(new byte[] { 240, 159, 145, 139, 32, 72, 101, 108, 108, 111, 44, 32, 87, 111, 114, 108, 100, 33 });
memoryStream.Position = 0;
using StreamReader streamReader = new StreamReader(memoryStream, Encoding.UTF8);
string extractedString = streamReader.ReadToEnd();
Console.WriteLine(extractedString);
Output:
๐ Hello, World!
Conclusion ๐
And that's it, my tech-savvy friend! We've explored two simple solutions to help you extract a string from a MemoryStream. Whether you prefer encoding or StreamReader, both methods will get you the desired result! ๐
Now it's your turn to give it a try! Use one of the solutions above and let me know how it goes. Did you encounter any issues or have a different approach? Feel free to share your thoughts and experiences in the comments below. Let the tech-conversation begin! ๐ฌ
Keep on coding! ๐
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
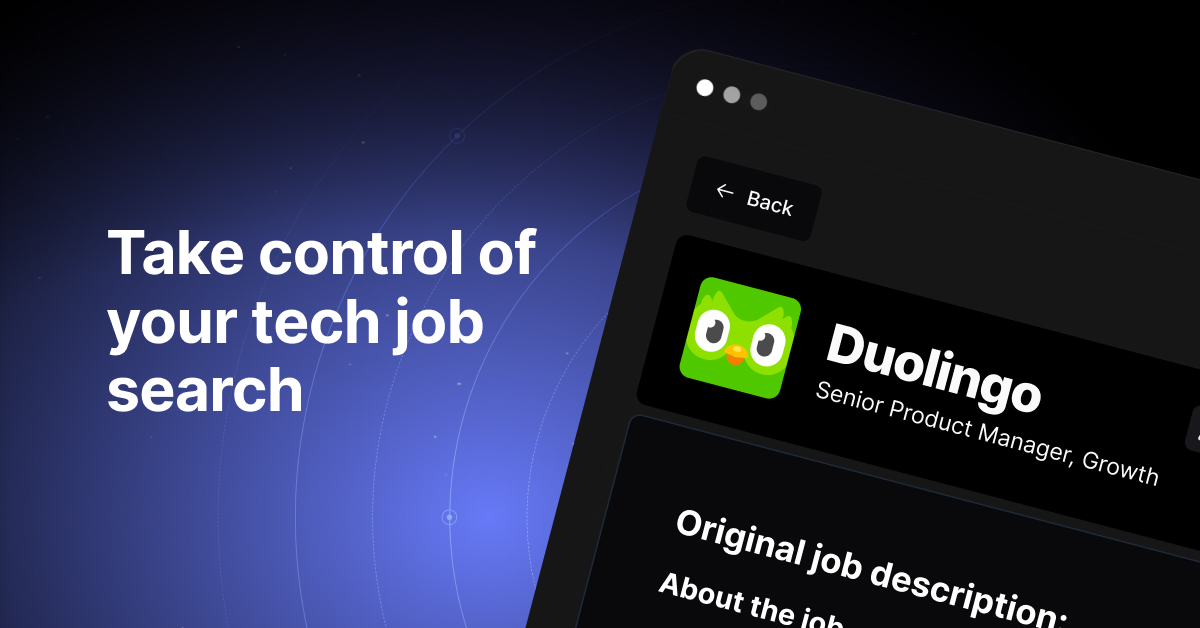