How do you configure an OpenFileDialog to select folders?
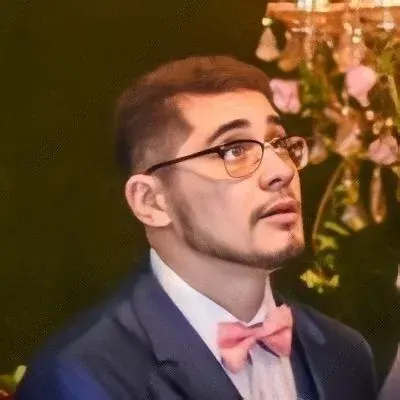
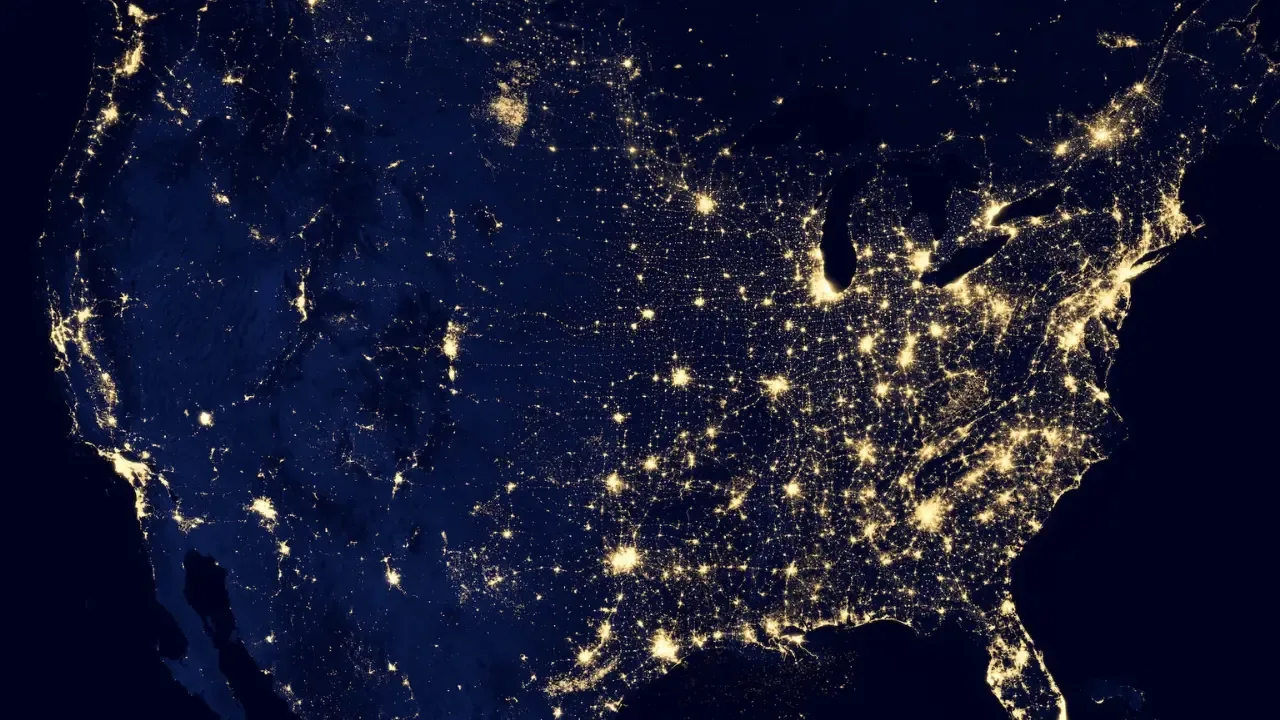
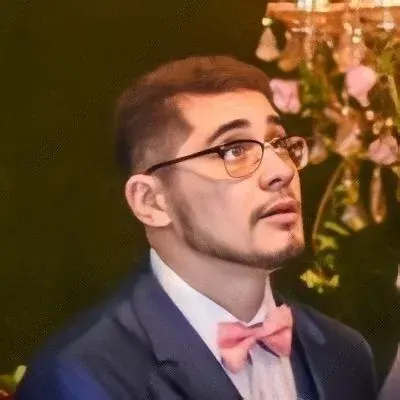
📂 Configuring an OpenFileDialog to Select Folders in .NET
So, you've come across a scenario where you need to configure an OpenFileDialog to select folders instead of files. You've probably noticed that the default OpenFileDialog doesn't allow you to do this. Don't worry, you're not alone! Many developers have faced this challenge and found ways to work around it. In this blog post, we'll explore some common issues, provide easy solutions, and satisfy your curiosity about configuring a dialog in this manner.
🤔 Why not just use the FolderBrowserDialog?
Before diving into alternative solutions, let's address the question of why some developers prefer not to use the FolderBrowserDialog. One common reason is that it may not provide the desired user experience. For example, it may start too small or restrict the ability to type a path directly. Additionally, sometimes developers want to maintain a consistent UI experience by using an OpenFileDialog-like dialog for selecting folders.
📁 How can we tackle this problem?
1. Leverage Unmanaged Code
While there isn't a direct way to configure an OpenFileDialog to select folders in .NET, you can achieve this behavior by leveraging unmanaged code. By modifying the dialog at a lower level, you can customize its behavior. However, this approach might require more effort and may not be suitable for all scenarios.
2. Use Custom Dialog Extensions
Another approach is to use custom dialog extensions provided by the operating system. Unfortunately, this solution only works on Vista and later versions of Windows. If you're targeting XP or an older version, this won't be viable.
3. Implement a Custom OpenFileDialog-like Dialog
If you're determined to have a consistent UI experience across different Windows versions, you can consider implementing a custom dialog that mimics the OpenFileDialog behavior but allows folder selection. This approach will require more development effort, but it offers full control over the dialog and ensures a consistent experience across different Windows versions.
⚡️ Example: Custom Folder Selection Dialog
Here's a simplified example of how you can implement a custom dialog in C# that allows folder selection:
using System;
using System.Windows.Forms;
namespace CustomFolderSelectionDialog
{
public partial class FolderSelectionDialog : Form
{
public string SelectedFolder { get; private set; }
public FolderSelectionDialog()
{
InitializeComponent();
}
private void btnSelectFolder_Click(object sender, EventArgs e)
{
using (var folderBrowserDialog = new FolderBrowserDialog())
{
if (folderBrowserDialog.ShowDialog() == DialogResult.OK)
{
SelectedFolder = folderBrowserDialog.SelectedPath;
}
}
Close();
}
}
}
In this example, we create a new form called FolderSelectionDialog, which contains a "Select Folder" button. When the button is clicked, we open the FolderBrowserDialog and store the selected folder's path in the SelectedFolder property. You can further customize this dialog to match your desired UI.
📢 Call-to-Action: Share Your Thoughts and Experiences!
Now that you have learned about different approaches to configuring an OpenFileDialog to select folders, it's time to engage with us! Have you encountered this challenge before? Which solution worked best for you, and why? Share your thoughts and experiences in the comments below. Let's continue the technical conversation and learn from each other!
Remember, while it's essential to satisfy your curiosity, it's equally important to consider the best solution for your specific use case. Happy coding! 💻🚀