Handling Dialogs in WPF with MVVM
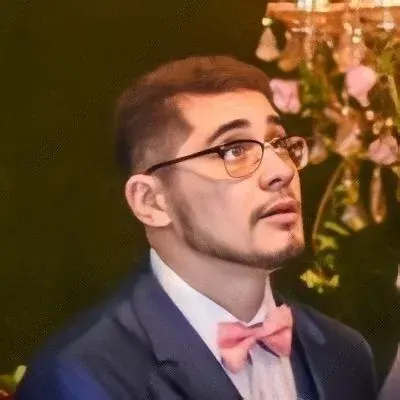
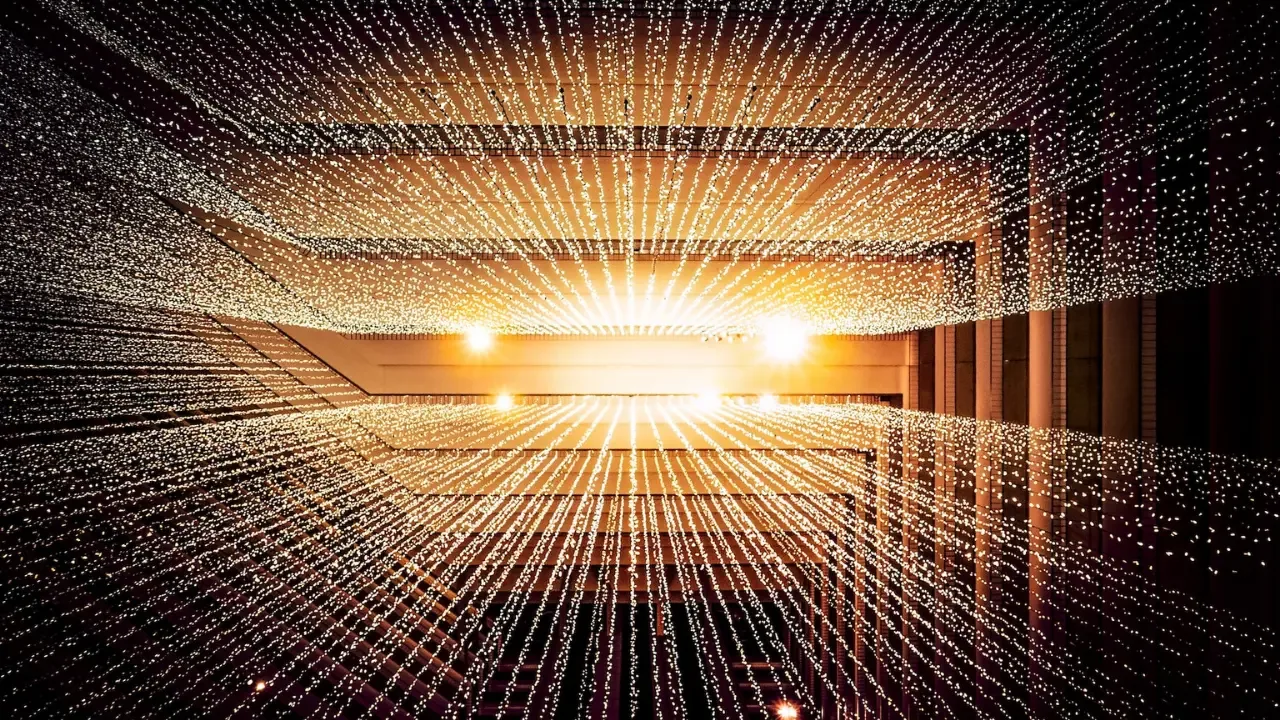
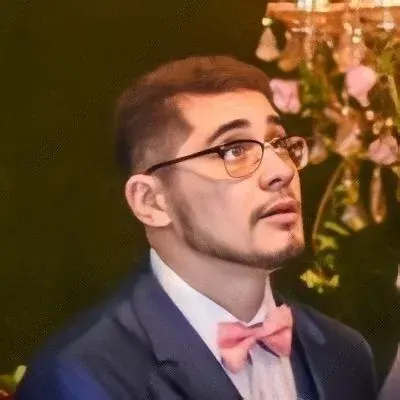
📝💡 Handling Dialogs in WPF with MVVM: A Guide for Easy and Effective Dialog Communication 💻📢
Are you a developer working with WPF and struggling with handling dialogs in the MVVM pattern? Trust me, you're not alone! 🤝💼
In the realm of MVVM, dialog communication can be a bit tricky since the view model doesn't have direct knowledge about the view. But fear not, because I've got some easy solutions for you! 🛠️👍
📌 The Problem: Dialog Communication in WPF MVVM
So, imagine you have a scenario where you need to show a dialog (like a MessageBox) in response to a user action. How can you handle the results from these dialogs in a clean and MVVM-friendly way? 🤔
Some developers resort to exposing an ICommand
in their view model, which the view can invoke to display the dialog. This approach works, but it means the view requires code, and that's something we'd like to avoid in the MVVM pattern. 😕
🌟 The Solution: A Cleaner Approach
One effective way to handle dialog communication without cluttering the view with code is by utilizing events. Here's how you can make it happen: 💡
First, declare an event in your view model that will serve as the trigger for displaying the dialog. Here's an example:
public event EventHandler<MyDeleteArgs> RequiresDeleteDialog;
In your view, subscribe to this event to be notified when a dialog is required. This way, the view can take appropriate action to display the dialog:
viewModel.RequiresDeleteDialog += ShowDeleteDialog;
When the event is raised in the view model, you can create a handler method (
ShowDeleteDialog
in this example) that will handle the logic for displaying the dialog. Inside this method, you have the freedom to use any dialog box mechanism that suits your needs, such as aMessageBox
:
private void ShowDeleteDialog(object sender, MyDeleteArgs e)
{
// Your code to show delete dialog using MessageBox or any other preferred way
}
🎉 Voila! You've Nailed Dialog Communication in MVVM
And there you have it! By following this approach, you can handle dialogs in WPF MVVM without polluting your view with unnecessary code. 🙌✨
Feel free to use this solution, tweak it to match your specific needs, and let your dialogs shine within the MVVM pattern! 💫🎭
📢 Your Turn: Engage and Share!
I hope you found this guide helpful in tackling dialog communication in WPF MVVM. Now, it's your turn to engage and share! ✨📣
Have you encountered any other challenges when handling dialogs in WPF MVVM? Share your experiences in the comments below! 💬👇
Did you find this solution useful? Are there any other approaches you'd like to recommend? Let's have a constructive discussion! 💡🗣️
Keep coding, keep learning, and keep building amazing WPF applications with smooth dialog communication in the MVVM pattern! 🚀🔧
Remember, together we can make the tech world a better place! 😉💪