Fluent Validation vs. Data Annotations
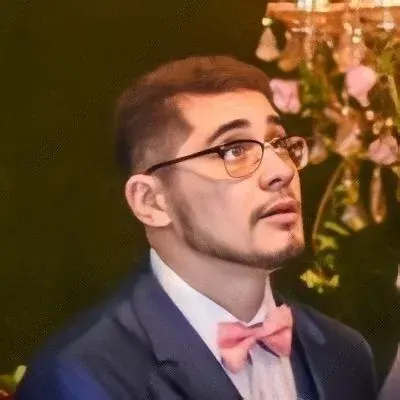
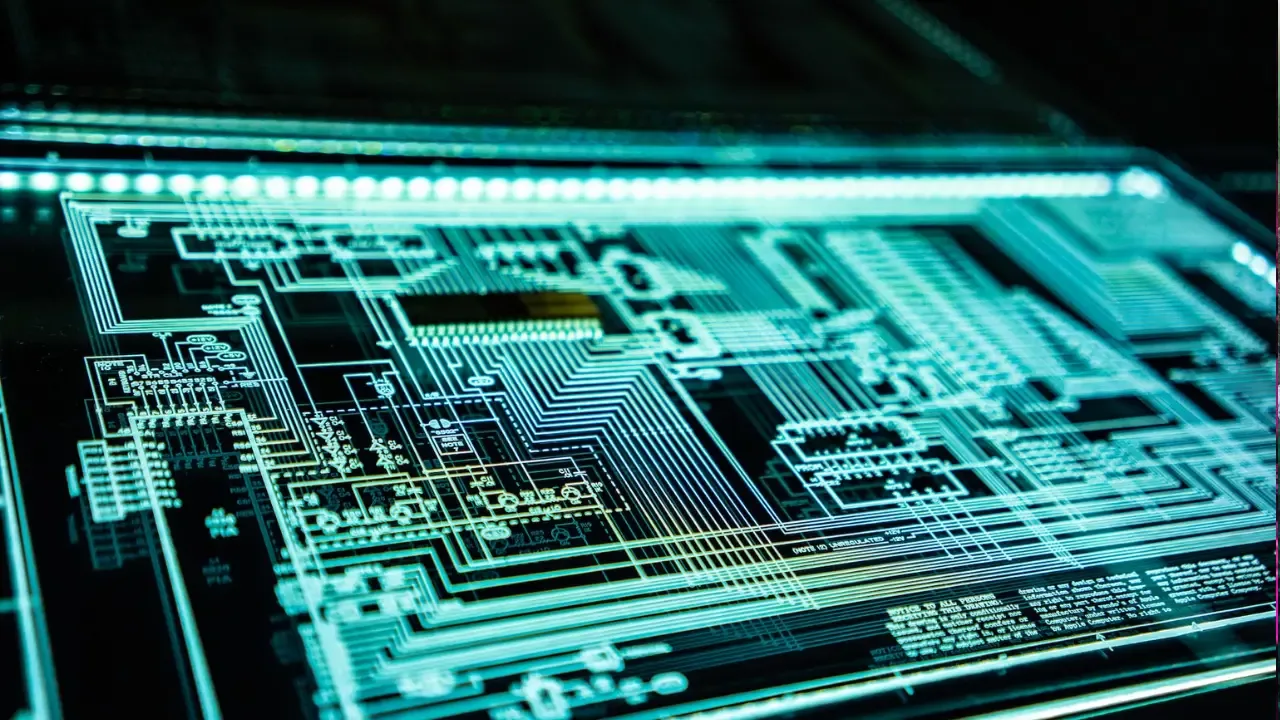
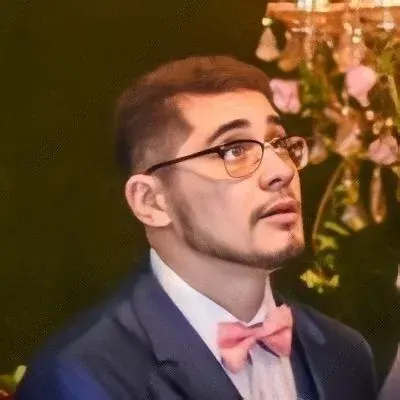
Fluent Validation vs. Data Annotations: Unraveling the Mystery 🕵️♀️
So you're building an awesome ASP.NET MVC application, and one thing you need to take care of is the validation of user inputs. You've come across two validation packages - Fluent Validation and Data Annotations, but you're not sure which one to use or what the differences are. Well, fear not! In this blog post, we'll dive deep into the world of validation and explore the similarities, differences, and best use cases for these two packages. Let's get started!
Understanding the Basics 📚
Both Fluent Validation and Data Annotations are popular solutions for implementing validation in ASP.NET MVC applications. They both provide a way to define validation rules for your models, ensuring that the user's input is accurate and meets your application's requirements. However, they differ in their approach and syntax.
Data Annotations: The Microsoft "Baked-in" Solution 🍰
Data Annotations is the built-in validation framework provided by Microsoft in ASP.NET MVC. It offers a range of attributes that you can apply to your model properties, such as [Required]
, [StringLength]
, and [RegularExpression]
. These attributes represent the validation rules and constraints for your fields.
Here's an example of how you can use Data Annotations to validate an email address property:
public class User
{
[Required]
[EmailAddress]
public string Email { get; set; }
}
In this case, the [Required]
attribute ensures that the email field is not empty, while the [EmailAddress]
attribute checks if the input follows the proper email format.
Fluent Validation: The 3rd Party Powerhouse 💪
On the other hand, Fluent Validation is a third-party library developed by Jeremy Skinner. It provides a more fluent and expressive way to define your validation rules. Instead of relying on attributes, Fluent Validation uses a separate set of validator classes that you can create for your models.
Let's take a look at how you can define the same validation rule for the email address property using Fluent Validation:
public class UserValidator : AbstractValidator<User>
{
public UserValidator()
{
RuleFor(user => user.Email)
.NotEmpty()
.EmailAddress();
}
}
Here, we create a validator class for the User
model and define the validation rules using the RuleFor
method. The NotEmpty
rule ensures that the email field is not empty, while the EmailAddress
rule checks the email format.
Choosing the Right Tool for the Job 🛠️
Now that we understand the basics of Data Annotations and Fluent Validation, let's discuss when it's best to use each of them.
Data Annotations: Simplicity and Quick Wins ✨
If you're looking for a quick and straightforward way to implement validation without adding any external dependencies, Data Annotations is the way to go. It's built-in, easy to use, and covers most of the common validation scenarios. It's a great choice for small to medium-sized projects where simplicity and convenience are priorities.
Fluent Validation: Customization and Flexibility 🎨
On the other hand, if you require advanced validation scenarios or need more control over your validation logic, Fluent Validation shines. With its expressive syntax and extensibility, you can easily create complex validation rules, cross-property validations, and even custom validation logic. Fluent Validation is best suited for larger projects or cases where you need fine-grained control over the validation process.
Wrap-up and Your Next Steps 👋
And there you have it! A comprehensive overview of Fluent Validation vs. Data Annotations for ASP.NET MVC validation. By now, you should have a clear understanding of their differences and when to use each of them.
If your project requires simplicity and basic validation, go with Data Annotations. It's there for you, baked into ASP.NET MVC. But if you need more advanced validation scenarios or want full control over your validation logic, Fluent Validation is your go-to.
No matter which tool you choose, always remember that proper validation is crucial for your application's integrity and security. So take your time, define your rules, and ensure that your users' inputs are validated accurately.
So, which validation package are you going to use in your ASP.NET MVC project? Share your thoughts and experiences in the comments below! Together, let's build better and more secure applications. 🚀