Databinding an enum property to a ComboBox in WPF
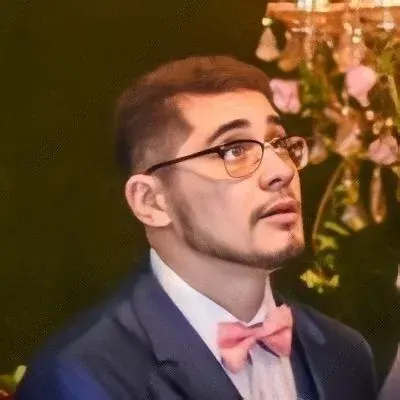
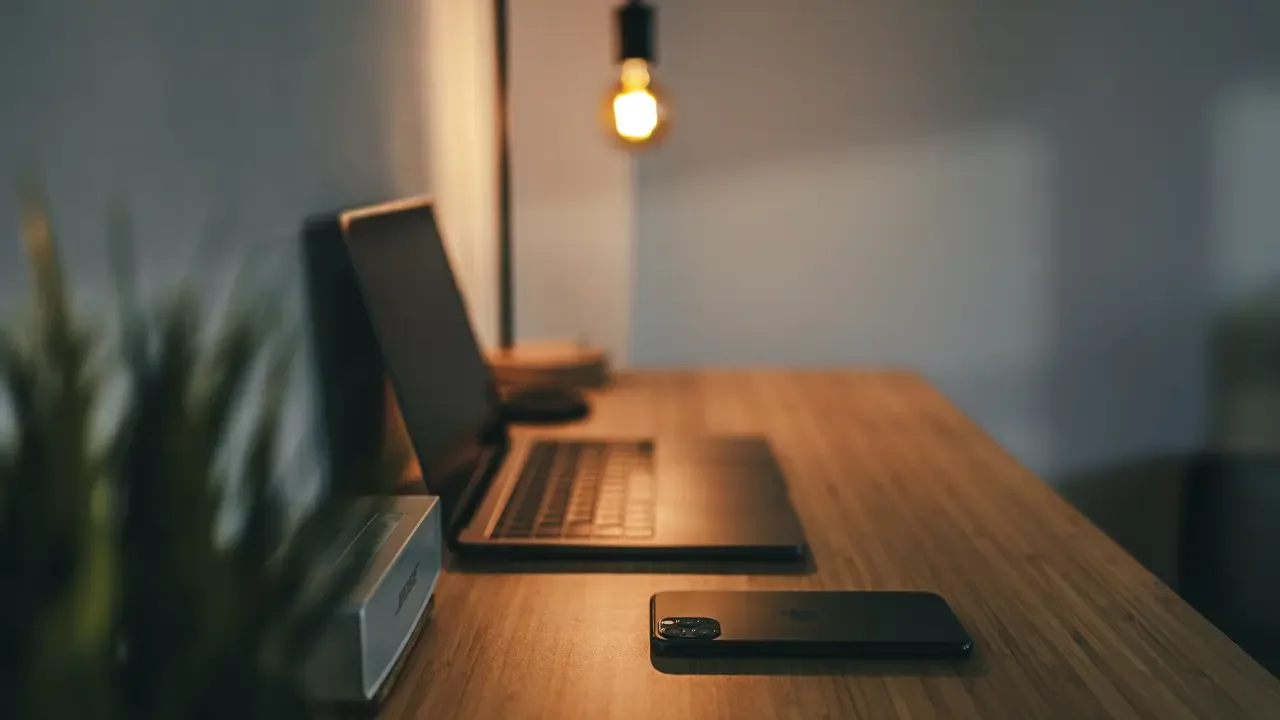
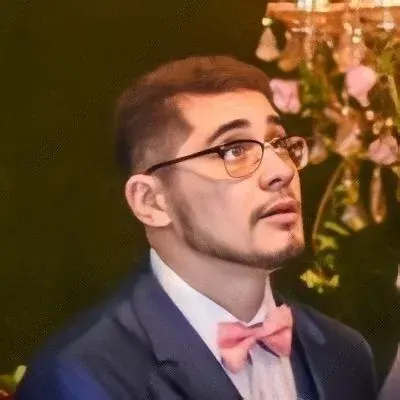
📝 Tech Blog: Databinding an Enum Property to a ComboBox in WPF 🖥️
Are you struggling with databinding an enum property to a ComboBox in WPF? 🤔 Don't worry, I've got you covered! In this blog post, I'll walk you through the common issues you might encounter and provide easy solutions to make your ComboBox work in mode TwoWay. Let's dive in and find out the secret! 💪
💡 The Challenge: Databinding an Enum Property to a ComboBox
Let's start with the code snippet you provided as an example:
public enum ExampleEnum { FooBar, BarFoo }
public class ExampleClass : INotifyPropertyChanged
{
private ExampleEnum example;
public ExampleEnum ExampleProperty
{
get { return example; }
set { /* set and notify */; }
}
}
The goal is to databind the ExampleProperty
enum to a ComboBox, displaying the options "FooBar" and "BarFoo" and allowing TwoWay binding. But how can we achieve that? 🤷
🔑 The Solution: Databinding the Enum Property
To populate the ComboBox with the right values and enable databinding, we can follow these steps:
Step 1️⃣: Retrieve the Enum values as a list of strings using an ObjectDataProvider and the static Enum.GetValues
method. Add the following code within the <Window.Resources>
section:
<Window.Resources>
<ObjectDataProvider MethodName="GetValues"
ObjectType="{x:Type sys:Enum}"
x:Key="ExampleEnumValues">
<ObjectDataProvider.MethodParameters>
<x:Type TypeName="ExampleEnum" />
</ObjectDataProvider.MethodParameters>
</ObjectDataProvider>
</Window.Resources>
Step 2️⃣: Use the ExampleEnumValues
as the ItemsSource
for your ComboBox. Update your ComboBox definition like this:
<ComboBox ItemsSource="{Binding Source={StaticResource ExampleEnumValues}}"
SelectedItem="{Binding Path=ExampleProperty}" />
That's it! Now your ComboBox should be populated with "FooBar" and "BarFoo", and the TwoWay binding should work as expected. 🥳
🌍 Bonus Tip: Internationalization (i18n)
If you want to add internationalization support to your ComboBox, you can utilize resource files to store different language values. But don't worry, we won't dive deep into it now. It's a topic for another blog post! 😉
✨ Reader Engagement: Share Your Experience!
Now that you have learned how to databind an enum property to a ComboBox in WPF, give it a try and share your experience with us! Did it solve your problem? Do you have any additional tips or tricks to add? Let's make this blog post an interactive hub for knowledge sharing. Leave a comment below and let's learn from each other! 🚀
I hope you found this blog post helpful and enjoyable to read! Stay tuned for more tech tips and tricks in the future. Happy coding! 👩💻👨💻
Remember, this blog post aims to simplify the process of databinding an enum property to a ComboBox in WPF, helping readers understand and implement it easily. The use of emojis adds a touch of fun and engagement to keep the readers entertained.